Mollie Payment gateway integration in Laravel 8
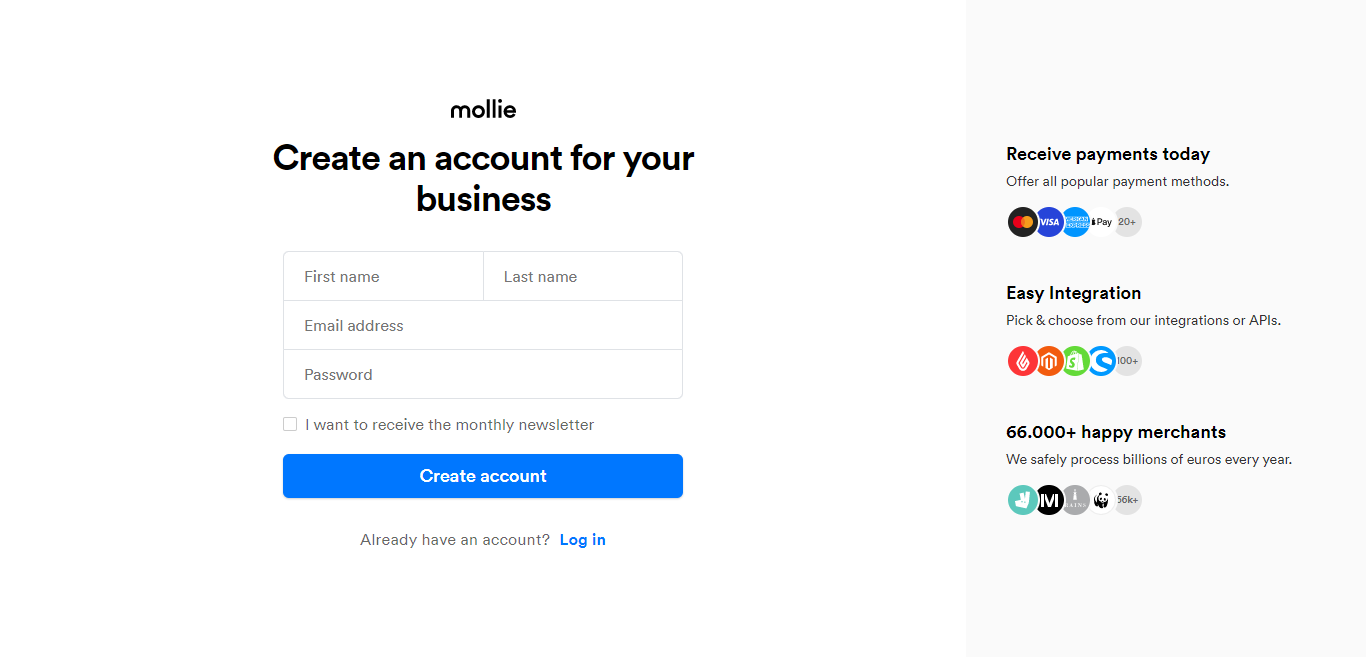
Before moving towards the topic I want to tell you what is a payment gateway and how it is beneficial for our website.
What is the Payment Gateway?
A payment gateway is a merchant service provided by an e-commerce application service provider that authorizes credit card or direct payments processing for e-businesses, online retailers. Payment gateway like Stripe, Razorpay, Mollie, Authorize are very popular nowadays.
Benefits of Mollie Payment Gateway
- Secure transactions
- Expanded customer base
- Faster transaction processing
Requirements
- Mollie account
- The Basic knowledge of PHP
- The basic idea of Laravel Framework
Create a Mollie account by clicking on this link.
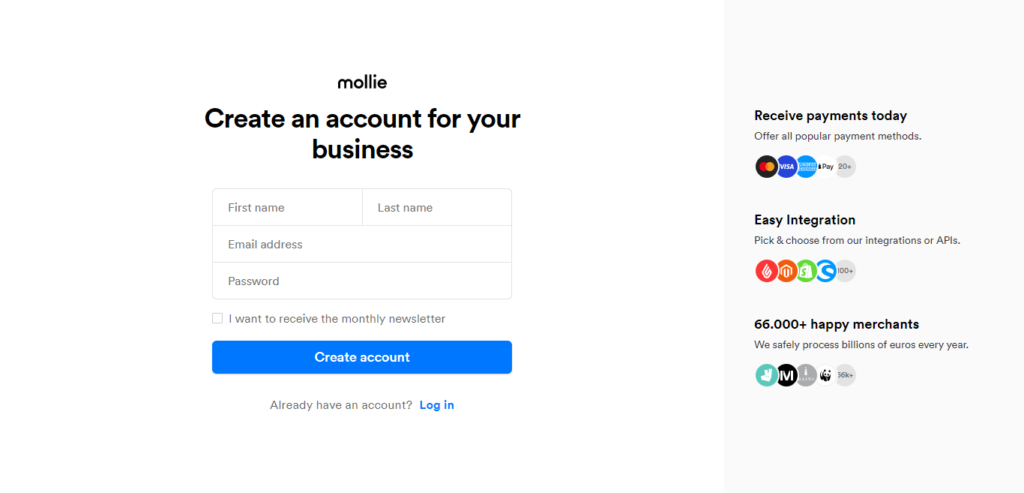
Login to Mollie dashboard in the sidebar you will see developers, click on developers to get your API Key.

Don’t forget to activate your payment method, as it gives errors during the payment. To activate the payment method you have to go settings -> website profile, then under the website profile, you can see the payment methods. You have to activate them.

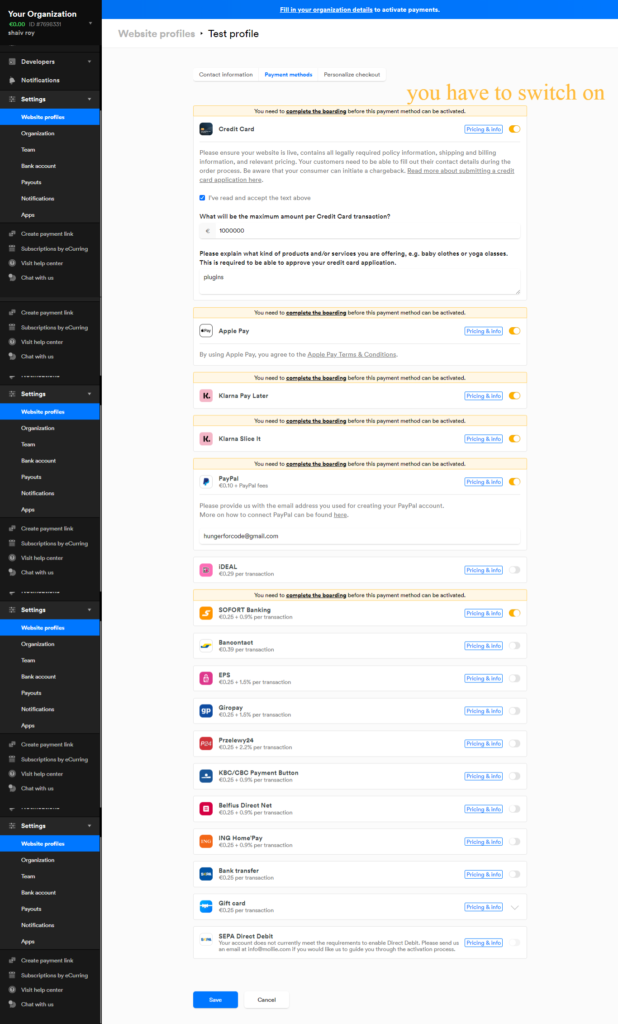
Installation
Add Laravel-Mollie to your composer file via the composer require
command:
composer require mollie/laravel-mollie
You can set the mollie API key in two way either by putting API key in you .env file like below
MOLLIE_KEY=test_xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx
Or by calling it in your constructor function like below.
public function __construct() {
Mollie::api()->setApiKey('test_FbVACj7UbsdkHtAUWnCnmSNUFHKuuA');
}
Add on the top of web.php file
use App\Http\Controllers\MollieController;
Add routes in your web.php file
Route::get('mollie-paymnet',[MollieController::Class,'preparePayment'])->name('mollie.payment');
Route::get('payment-success',[MollieController::Class, 'paymentSuccess'])->name('payment.success');
Create a controller by using the following command
php artisan make:controller MollieController
Under your controller put the following code
<?php
namespace App\Http\Controllers;
use Mollie\Laravel\Facades\Mollie;
class MollieController extends Controller
{
public function __construct() {
Mollie::api()->setApiKey('test_FbVACj7UbsdkHtAUWnCnmSNGFWMuuA'); // your mollie test api key
}
/**
* Redirect the user to the Payment Gateway.
*
* @return Response
*/
public function preparePayment()
{
$payment = Mollie::api()->payments()->create([
'amount' => [
'currency' => 'EUR', // Type of currency you want to send
'value' => '10.00', // You must send the correct number of decimals, thus we enforce the use of strings
],
'description' => 'Payment By codehunger',
'redirectUrl' => route('payment.success'), // after the payment completion where you to redirect
]);
$payment = Mollie::api()->payments()->get($payment->id);
// redirect customer to Mollie checkout page
return redirect($payment->getCheckoutUrl(), 303);
}
/**
* Page redirection after the successfull payment
*
* @return Response
*/
public function paymentSuccess() {
echo 'payment has been received';
}
}
Visit the below URL
http://localhost/mollie/public/mollie-payment
After visiting on the URL, you are redirected to the Mollie payment page

Here all your payment methods will be shown, which you activated in your profile. You can choose any payment method to make the payment.
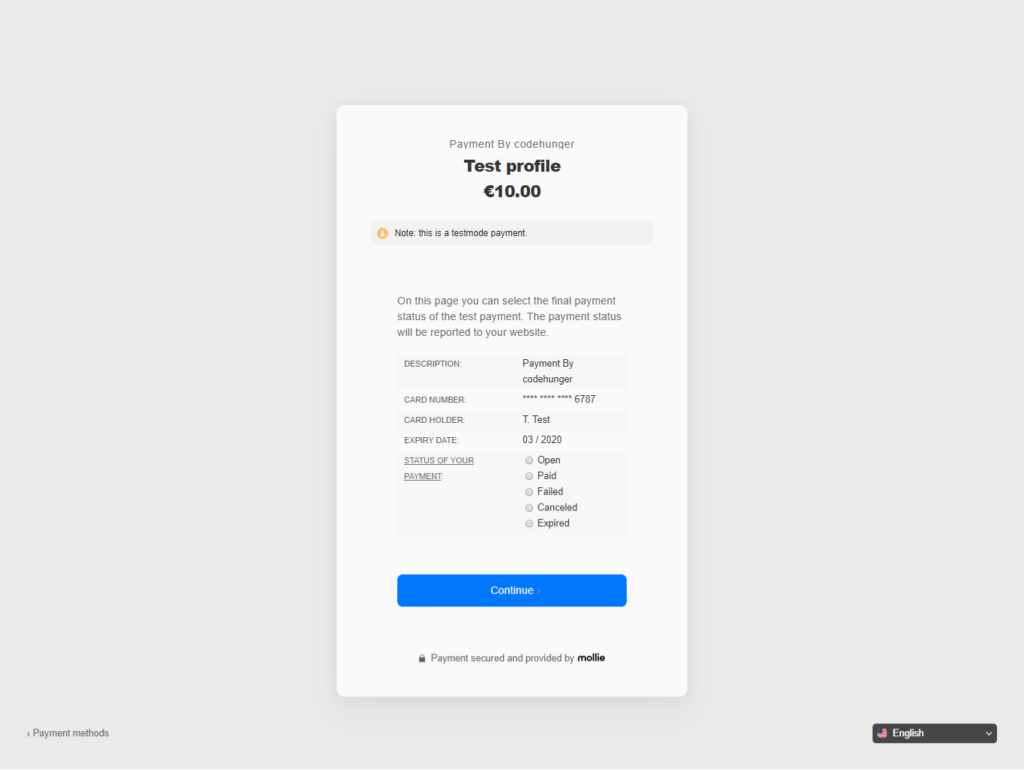
Now you can click on paid for your successful payment. You can see your all payment details under your transaction details.

Still have questions in mind feel free to ask, we are here to help you.
This is helpful
yes, it’s really helpful content provided by CodeHunger.
Could you also do one for Cashier Mollie (recurring payments). There are literally 0 tutorials on that besides the hard to understand github
Yes, sure we will make a video on that soon. Till subscribed to our blog, so that you will get notified about that.