React Native – ListView

In this chapter, we will show you how to create a list in React Native.
A core component designed for efficient display of vertically scrolling lists of changing data. The minimal API is to create a ListView.DataSource, populate it with a flat array of data blobs, and instantiate a ListView component with that data source and a renderRow callback which takes a blob from the data array and returns a renderable component.
The ListView component has deprecated. To implement the list component use new list components FlatList or SectionList.
import React, { Component } from 'react'
import { Text, View, TouchableOpacity, StyleSheet } from 'react-native'
class List extends Component {
state = {
names: [
{
id: 0,
name: 'Ram',
},
{
id: 1,
name: 'Laksham',
},
{
id: 2,
name: 'Bharat',
},
{
id: 3,
name: 'Shatrughan',
}
]
}
alertItemName = (item) => {
alert(item.name)
}
render() {
return (
<View>
{
this.state.names.map((item, index) => (
<TouchableOpacity
key = {item.id}
style = {styles.container}
onPress = {() => this.alertItemName(item)}>
<Text style = {styles.text}>
{item.name}
</Text>
</TouchableOpacity>
))
}
</View>
)
}
}
export default List
const styles = StyleSheet.create ({
container: {
padding: 10,
marginTop: 3,
backgroundColor: '#d9f9b1',
alignItems: 'center',
},
text: {
color: '#4f603c'
}
})
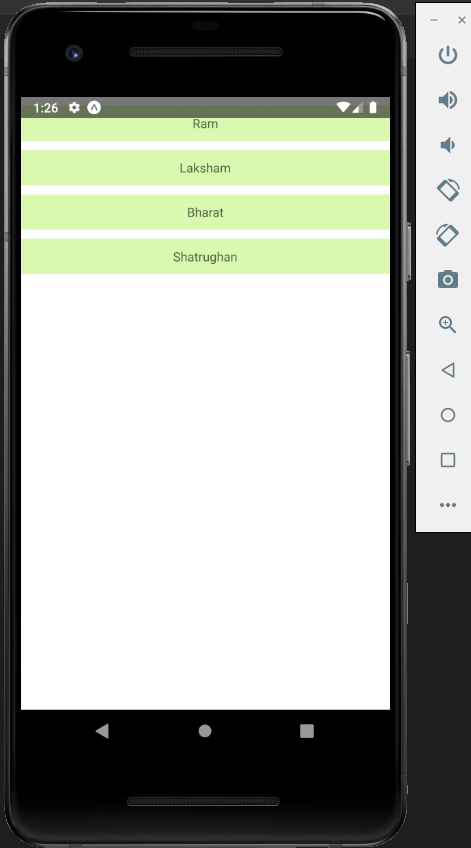

In the above code, we create an instance of ListViewDataSource in the constructor. The ListViewDataSource is a parameter and accept an argument which contain any of these four:
- getRowData(dataBlob, sectionID, rowID)
- getSectionHeaderData(dataBlob, sectionID)
- rowHasChanged(previousRowData, nextRowData)
- sectionHeaderHasChanged(previousSectionData, nextSectionData)
ListView also supports more advanced features, including sections with sticky section headers, header and footer support, callbacks on reaching the end of the available data (onEndReached
) and on the set of rows that are visible in the device viewport change (onChangeVisibleRows
), and several performance optimizations.
There are a few performance operations designed to make ListView scroll smoothly while dynamically loading potentially very large (or conceptually infinite) data sets:
- Only re-render changed rows – the rowHasChanged function provided to the data source tells the ListView if it needs to re-render a row because the source data has changed – see ListViewDataSource for more details.
- Rate-limited row rendering – By default, only one row is rendered per event-loop (customizable with the
pageSize
prop). This breaks up the work into smaller chunks to reduce the chance of dropping frames while rendering rows.