
While working with TextInput in native app development we don’t have to take care of the focus and keyboard avoiding because it is done by the app itself but in case of React Native, we have to take care of all this stuff so that we can provide a full native feel to the user.
Request Focus is used to set the focus to the TextInput while the keyboard is up and visible. It also helps to navigate to the next TextInput Using the following way.
How to use Request Focus
ref={ref => {
this.currentinputReferance= ref;
}}
returnKeyType="next"
onSubmitEditing={() =>
this.nextinputReferance && this.nextinputReferance.focus()
}
Keyboard Avoiding View is used to close the keyboard. It wraps the whole form that we made using TextInput and close the keyboard if touched outside the TextInput. It is very helpful in the case of IOS because it does have a back button to close the keyboard. This feature is by default in React Native TextInput but if we are using Request Focus then sometimes it becomes very essential to use the Keyboard Avoiding View.
<KeyboardAvoidingView enabled>
//Your whole view(Form)
</KeyboardAvoidingView>
Example
import React, { Component } from 'react';
import {
StyleSheet,
TextInput,
View,
Button,
Text,
KeyboardAvoidingView,
Keyboard,
TouchableOpacity,
ScrollView,
} from 'react-native';
export default class App extends Component {
constructor(props) {
super(props);
this.state = {
UserName: '',
UserEmail: '',
UserAge: '',
UserAddress: '',
};
}
render() {
return (
<View
style={{
flex: 1,
backgroundColor: '#FFFFFF',
justifyContent: 'center',
}}>
<ScrollView keyboardShouldPersistTaps="handled">
<View style={{ marginTop: 100 }}>
<KeyboardAvoidingView enabled>
<View style={styles.SectionStyle}>
<TextInput
style={{ flex: 1, color: '#413E4F' }}
onChangeText={UserName => this.setState({ UserName })}
underlineColorAndroid="#413E4F"
placeholder="Enter Name"
placeholderTextColor="#413E4F"
autoCapitalize="sentences"
ref={ref => {
this._nameinput = ref;
}}
returnKeyType="next"
onSubmitEditing={() =>
this._emailinput && this._emailinput.focus()
}
blurOnSubmit={false}
/>
</View>
<View style={styles.SectionStyle}>
<TextInput
style={{ flex: 1, color: '#413E4F' }}
onChangeText={UserEmail => this.setState({ UserEmail })}
underlineColorAndroid="#413E4F"
placeholder="Enter Email"
placeholderTextColor="#413E4F"
autoCapitalize="sentences"
keyboardType="email-address"
ref={ref => {
this._emailinput = ref;
}}
returnKeyType="next"
onSubmitEditing={() =>
this._ageinput && this._ageinput.focus()
}
blurOnSubmit={false}
/>
</View>
<View style={styles.SectionStyle}>
<TextInput
style={{ flex: 1, color: '#413E4F' }}
onChangeText={UserAge => this.setState({ UserAge })}
underlineColorAndroid="#413E4F"
placeholder="Enter Age"
placeholderTextColor="#413E4F"
autoCapitalize="sentences"
keyboardType="numeric"
ref={ref => {
this._ageinput = ref;
}}
onSubmitEditing={() =>
this._addressinput && this._addressinput.focus()
}
blurOnSubmit={false}
/>
</View>
<View style={styles.SectionStyle}>
<TextInput
style={{ flex: 1, color: '#413E4F' }}
onChangeText={UserAddress => this.setState({ UserAddress })}
underlineColorAndroid="#413E4F"
placeholder="Enter Address"
placeholderTextColor="#413E4F"
autoCapitalize="sentences"
ref={ref => {
this._addressinput = ref;
}}
returnKeyType="next"
onSubmitEditing={Keyboard.dismiss}
blurOnSubmit={false}
/>
</View>
<TouchableOpacity
style={styles.ButtonStyle}
activeOpacity={0.5}
onPress={this.UserRegisterFunction}>
<Text
style={{
color: '#FFFFFF',
paddingVertical: 10,
fontSize: 16,
}}>
REGISTER
</Text>
</TouchableOpacity>
</KeyboardAvoidingView>
</View>
</ScrollView>
</View>
);
}
}
const styles = StyleSheet.create({
SectionStyle: {
flexDirection: 'row',
height: 40,
marginTop: 20,
marginLeft: 35,
marginRight: 35,
margin: 10,
},
ButtonStyle: {
backgroundColor: '#51D8C7',
borderWidth: 0,
color: '#FFFFFF',
borderColor: '#51D8C7',
height: 40,
alignItems: 'center',
borderRadius: 5,
marginLeft: 35,
marginRight: 35,
marginTop: 30,
},
});
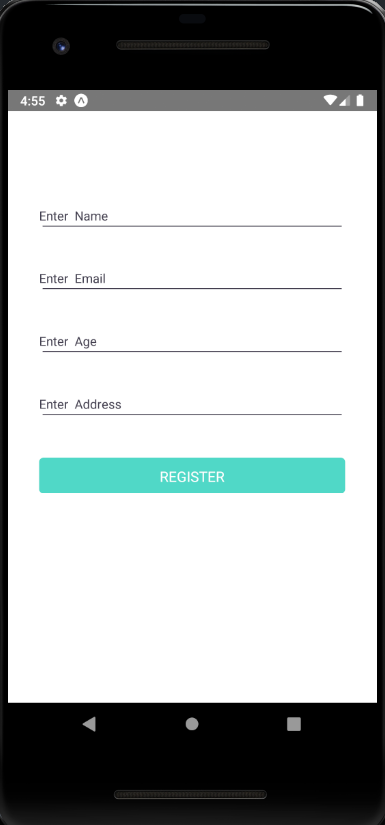
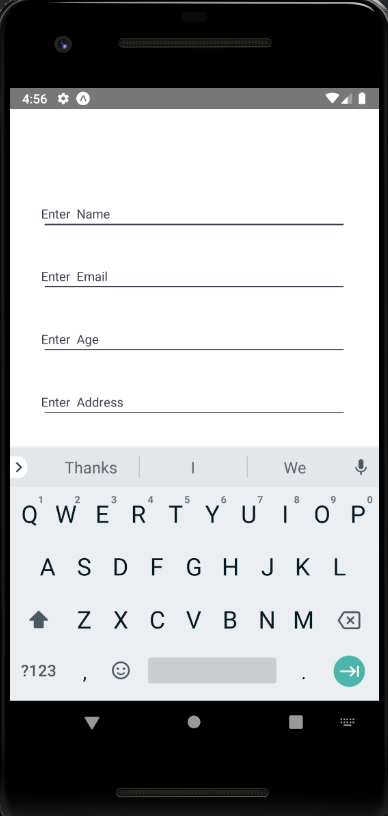