Laravel
Step- by- Step companion to Planting a Laravel Project
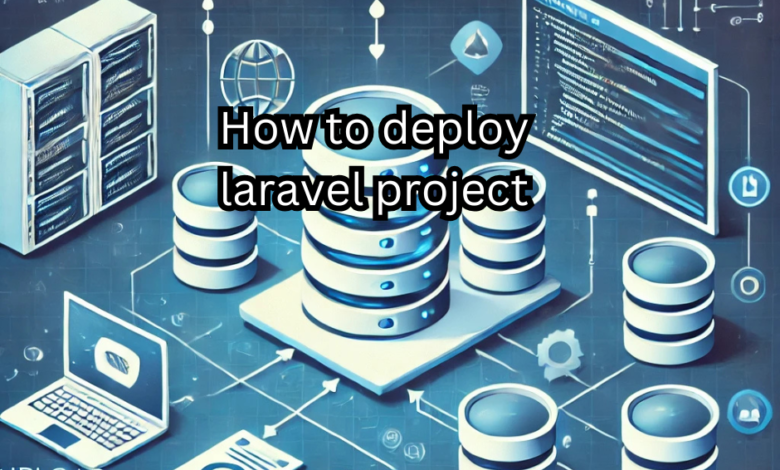
Step- by- Step companion to Planting a Laravel Project:
Step 1: Prepare Your Environment
- Server Selection: Choose a hosting environment like a VPS, shared hosting, or cloud platforms like AWS, DigitalOcean, or Heroku.
- Install PHP & Composer:
- Ensure the server supports the required PHP version for Laravel.Install Composer (a dependency manager for PHP).
sudo apt update
sudo apt install php-cli unzip curl
curl -sS https://getcomposer.org/installer | php
sudo mv composer.phar /usr/local/bin/composer
- Install Database: Set up a database like MySQL, PostgreSQL, or SQLite.
Step 2: Upload Your Laravel Project
- Compress your project files (excluding
node_modules
andvendor
directories) into a.zip
file. - Upload the file to your server using tools like FileZilla, cPanel, or the command line (
scp
). - Extract the files in the server directory (e.g.,
/var/www/html/project_name
).
unzip project.zip -d /var/www/html/project_name
Step 3: Configure the Environment
- Set File Permissions:
- Ensure the
storage
andbootstrap/cache
directories are writable
- Ensure the
chmod -R 775 storage bootstrap/cache
Set the Environment File:
- Update
.env
with the correct database and application configurations:
APP_NAME=YourAppName
APP_ENV=production
APP_KEY=base64:generated_key
APP_DEBUG=false
APP_URL=http://yourdomain.com
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=your_db_user
DB_PASSWORD=your_db_password
Step 4: Install Dependencies
Run Composer to install dependencies on the server:
composer install --optimize-autoloader --no-dev
Step 5: Generate the Application Key
Run the following command to generate a key for your Laravel application:
php artisan key:generate
Step 6: Migrate the Database
Run database migrations to create tables in your database:
php artisan migrate --force
Step 7: Configure Apache/Nginx
- Set Up Virtual Host (Apache): Create a virtual host configuration file:
sudo nano /etc/apache2/sites-available/yourdomain.com.conf
Add the following configuration:
<VirtualHost *:80>
ServerName yourdomain.com
DocumentRoot /var/www/html/project_name/public
<Directory /var/www/html/project_name>
AllowOverride All
Require all granted
</Directory>
ErrorLog ${APACHE_LOG_DIR}/error.log
CustomLog ${APACHE_LOG_DIR}/access.log combined
</VirtualHost>
Enable the site and restart Apache:
sudo a2ensite yourdomain.com.conf
sudo a2enmod rewrite
sudo systemctl restart apache2
Set Up Server Block (Nginx): Create a configuration file:
sudo nano /etc/nginx/sites-available/yourdomain.com
Add the following:
server {
listen 80;
server_name yourdomain.com;
root /var/www/html/project_name/public;
index index.php index.html;
location / {
try_files $uri $uri/ /index.php?$query_string;
}
location ~ \.php$ {
include snippets/fastcgi-php.conf;
fastcgi_pass unix:/var/run/php/php7.4-fpm.sock;
fastcgi_param SCRIPT_FILENAME $document_root$fastcgi_script_name;
include fastcgi_params;
}
location ~ /\.ht {
deny all;
}
}
Enable the configuration and restart Nginx:
sudo ln -s /etc/nginx/sites-available/yourdomain.com /etc/nginx/sites-enabled/
sudo systemctl restart nginx
Step 8: Optimize Your Application
- Cache the configuration:bashCopy code
php artisan config:cache
php artisan route:cache
php artisan view:cache
Clear unnecessary caches:
php artisan cache:clear
Step 9: Test Your Application
Visit your domain (e.g., http://yourdomain.com
) to ensure the application works. Check logs if you encounter issues:
tail -f storage/logs/laravel.log
Step 10: Secure Your Application
- Enable SSL: Use Let’s Encrypt or another SSL provider to secure your site with HTTPS.
- Harden Permissions: Ensure the
.env
file and other sensitive files are not accessible publicly. - Regular Updates: Keep Laravel and server packages updated.