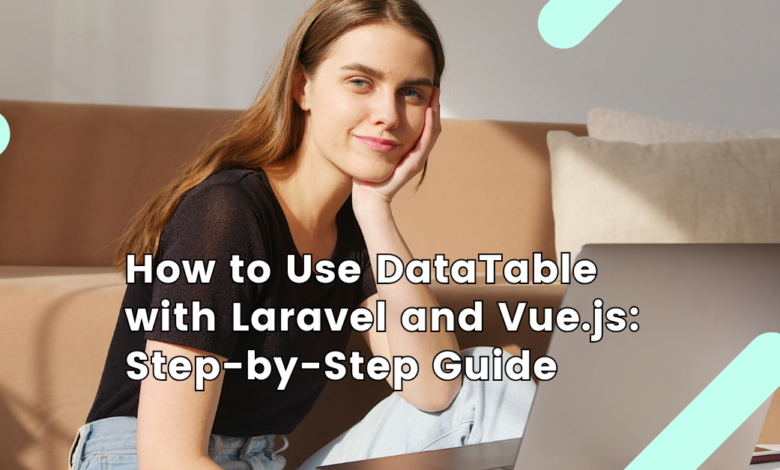
Introduction
If you’re working on a Laravel and Vue.js project, handling large datasets efficiently can be a challenge. That’s where DataTable comes in. It helps you display, sort, and paginate data seamlessly, improving user experience and application performance.
In this guide, we’ll walk through integrating DataTable with Laravel and Vue.js step by step. By the end, you’ll have a fully functional dynamic table displaying data efficiently.
Step 1: Setting Up Laravel Backend
1. Install Laravel
First, if you haven’t already set up Laravel, create a new project using Composer:
composer create-project --prefer-dist laravel/laravel laravel-datatable
cd laravel-datatable
2. Configure Database
Update your .env
file with your database credentials:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=your_username
DB_PASSWORD=your_password
3. Create a Model and Migration
Run the following command to generate a model and migration for a sample users
table:
php artisan make:model User -m
Modify the database/migrations/xxxx_xx_xx_create_users_table.php
file:
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamps();
});
}
Run the migration:
php artisan migrate
4. Create a Controller and API Route
Generate a controller for handling API requests:
php artisan make:controller UserController
Modify app/Http/Controllers/UserController.php
:
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
public function index()
{
return response()->json(User::paginate(10));
}
}
Define the API route in routes/api.php
:
use App\Http\Controllers\UserController;
Route::get('/users', [UserController::class, 'index']);
Step 2: Setting Up Vue.js Frontend
1. Install Vue in Laravel
If Vue.js is not already installed in your Laravel project, install it using:
npm install vue
Next, install Axios for API requests:
npm install axios
2. Install and Configure DataTables in Vue
Install the DataTables library:
npm install vue3-datatable
Now, create a new Vue component for displaying users.
Inside resources/js/components/UserTable.vue
, add the following code:
<template>
<div>
<h2>User DataTable</h2>
<vue3-datatable :items="users" :columns="columns"></vue3-datatable>
</div>
</template>
<script>
import { ref, onMounted } from 'vue';
import axios from 'axios';
export default {
setup() {
const users = ref([]);
const columns = ref([
{ key: 'id', label: 'ID' },
{ key: 'name', label: 'Name' },
{ key: 'email', label: 'Email' }
]);
onMounted(async () => {
const response = await axios.get('/api/users');
users.value = response.data.data;
});
return { users, columns };
}
};
</script>
Step 3: Integrate Vue Component in Laravel
1. Register Vue Component
Modify resources/js/app.js
to register the Vue component:
import { createApp } from 'vue';
import UserTable from './components/UserTable.vue';
const app = createApp({});
app.component('user-table', UserTable);
app.mount('#app');
2. Add Component to Blade File
Modify resources/views/welcome.blade.php
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Laravel Vue DataTable</title>
@vite(['resources/js/app.js'])
</head>
<body>
<div id="app">
<user-table></user-table>
</div>
</body>
</html>
Step 4: Run and Test the Application
Now, compile the assets and run the Laravel development server:
npm run dev
php artisan serve
Visit http://127.0.0.1:8000
, and you should see the DataTable displaying user data dynamically.
Using DataTable with Laravel and Vue.js makes it easier to manage large datasets, improve performance, and provide a better user experience. By following these steps, you now have a working example of how to integrate DataTable in a Laravel Vue.js application.
If you’re looking for expert website development solutions, CodeHunger is one of the top companies to consider.