C# – Arrays
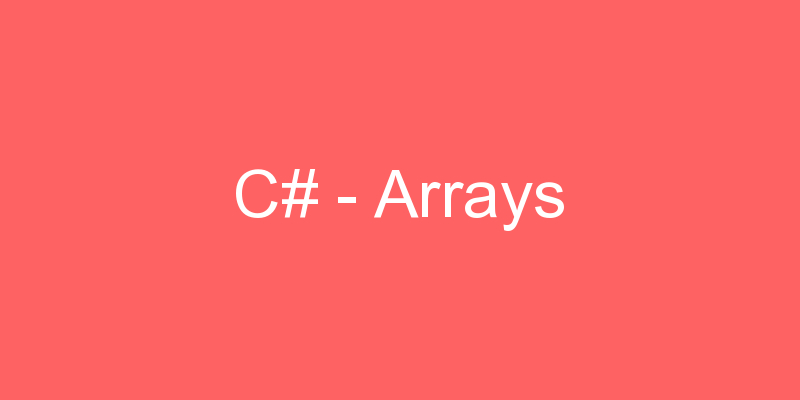
An array is a collection of variables of the same type that are referred to by a common name. In C#, arrays can have one or more dimensions, although the one-dimensional array is the most common. Arrays are used for a variety of purposes because they offer a convenient means of grouping together related variables. For example, you might use an array to hold a record of the daily high temperature for a month, a list of stock prices, or your collection of programming books.
Arrays are vital for most programming languages. They are collections of variables, which we call elements.
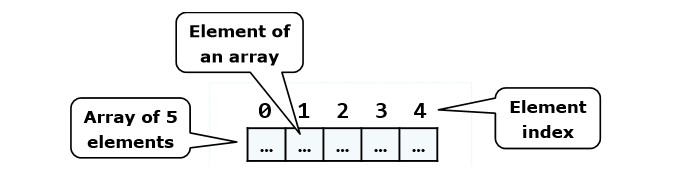
An array’s elements in C# are numbered with 0, 1, 2, … N-1. Those numbers are called indices. The total number of elements in a given array we call length of an array. All elements of a given array are of the same type, no matter whether they are primitive or reference types. This allows us to represent a group of similar elements as an ordered sequence and work on them as a whole.
Syntax – type[ ] array-name = new type[size];
Example
int[] arr;
In this example the variable arr is the name of the array, which is of integer type (int[]). This means that we declared an array of integer numbers. With [] we indicate, that the variable, which we are declaring, is an array of elements, not a single element. When we declare an array type variable, it is a reference, which does not have a value (it points to null). This is because the memory for the elements is not allocated yet.
int[] arr = new int[6];
In this example we allocate an array with length of 6 elements of type int. This means that in the dynamic memory (heap) an area of 6 integer numbers is allocated and they all are initialized with the value 0. In C#, the elements of an array are always stored in the dynamic memory (called also heap).
Initializing an Array
In the preceding program, the nums array was given values by hand, using ten separate assignment statements. While that is perfectly correct, there is an easier way to accomplish this. Arrays can be initialized when they are created. The general form for initializing a onedimensional array is shown here:
type[ ] array-name = { val1, val2, val3, …, valN };
Here, the initial values are specified by val1 through valN. They are assigned in sequence, left to right, in index order. C# automatically allocates an array large enough to hold the initializers that you specify. There is no need to use the new operator explicitly.
For example, here is a better way to write the Average program:
// Compute the average of a set of values.
using System;
class Average
{
static void Main() {
int[] nums = { 99, 10, 100, 18, 78, 23, 63, 9, 87, 49 };
int avg = 0;
for(int i=0; i < 10; i++)
avg = avg + nums[i];
avg = avg / 10;
Console.WriteLine("Average: " + avg);
}
}
As a point of interest, although not needed, you can use new when initializing an array. For example, this is a proper, but redundant, way to initialize nums in the foregoing program:
int[] nums = new int[] { 99, 10, 100, 18, 78, 23, 63, 9, 87, 49 };
string[] daysOfWeek = { "Monday", "Tuesday", "Wednesday","Thursday", "Friday", "Saturday", "Sunday" };
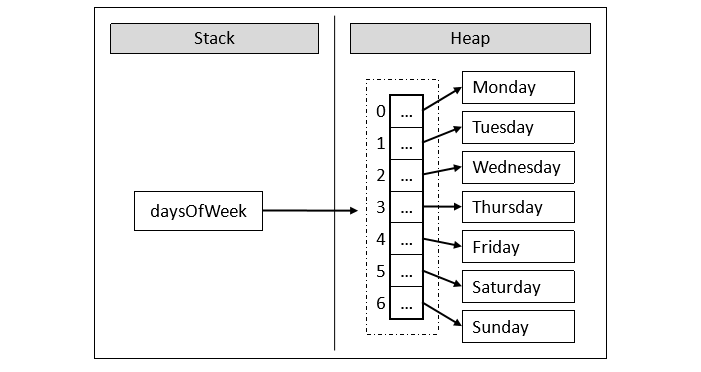
We can also access the elements of the array like this :
int[] arr = new int[6];
arr[1] = 1;
arr[5] = 5;
Boundaries Are Enforced in Arrays
Array boundaries are strictly enforced in C#; it is a runtime error to overrun or underrun the ends of an array. If you want to confirm this for yourself, try the following program that purposely overruns an array:
// Demonstrate an array overrun.
using System;
class ArrayErr {
static void Main() {
int[] sample = new int[10];
int i; // Generate an array overrun.
for(i = 0; i < 100; i = i+1)
sample[i] = i;
}
}
As soon as i reaches 10, an IndexOutOfRangeException is generated and the program is terminated.