C# – Data Types

Data types are sets (ranges) of values that have similar characteristics. For instance byte type specifies the set of integers in the range of [0 … 255]. C# is a strongly-typed language. It means we must declare the type of a variable which indicates the kind of values it is going to store such as integer, float, decimal, text, etc.
Characteristics
Data types are characterized by:
- Name – for example, int;
- Size (how much memory they use) – for example, 4 bytes;
- Default value – for example 0.
Example: Variables of Different Data Types
string stringVar = "Hello World!!";
int intVar = 100;
float floatVar = 10.2f;
char charVar = 'A';
bool boolVar = true;
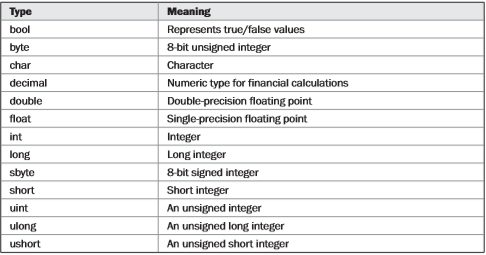
Types
Basic data types in C# are distributed into the following types:
- Integer types – sbyte, byte, short, ushort, int, uint, long, ulong;
- Real floating-point types – float, double;
- Real type with decimal precision – decimal;
- Boolean type – bool;
- Character type – char;
- String – string;
- Object type – object.
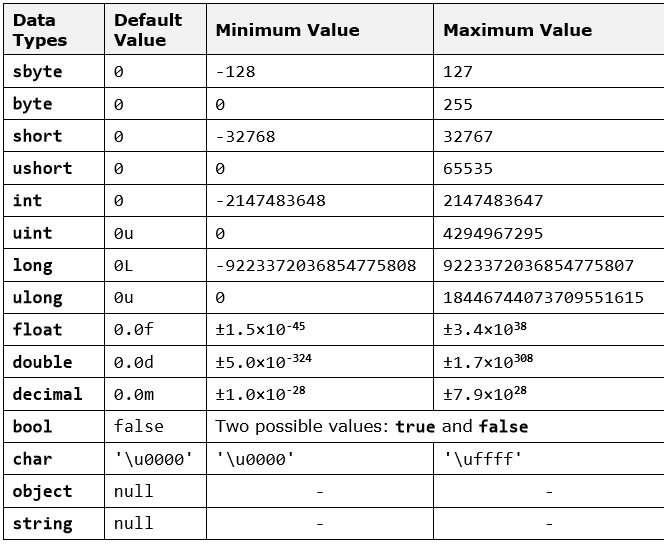
Integers
C# defines nine integer types: char, byte, sbyte, short, ushort, int, uint, long, and ulong. However, the char type is primarily used for representing characters, and it is discussed later in this chapter. The remaining eight integer types are used for numeric calculations.
// Declare some variables
byte centuries = 20;
ushort years = 2000;
uint days = 730480;
ulong hours = 17531520;
// Print the result on the console
Console.WriteLine(centuries + " centuries are " + years + " years, or " + days + " days, or " + hours + " hours.");
// Console output: // 20 centuries are 2000 years, or 730480 days, or 17531520 // hours.
ulong maxIntValue = UInt64.MaxValue;
Console.WriteLine(maxIntValue); // 18446744073709551615
Floating-Point Types
The floating-point types can represent numbers that have fractional components. There are two kinds of floating-point types, float and double, which represent single- and doubleprecision numbers, respectively. The type float is 32 bits wide and has an approximate range of 1.5E–45 to 3.4E+38. The double type is 64 bits wide and has an approximate range of 5E–324 to 1.7E+308.
class FindRadius
{
static void Main()
{
Double r;
Double area;
area = 10.0;
r = Math.Sqrt(area / 3.1416);
Console.WriteLine("Radius is " + r);
}
}
The output from the program is shown here:
Radius is 1.78412203012729
Real Type Double
The second real floating-point type in the C# language is the double type. It is also called double precision real number and is a 64-bit type with a default value of 0.0d and 0.0D (the suffix ‘d’ is not mandatory because by default all real numbers in C# are of type double). This type has precision of 15 to 16 decimal digits. The range of values, which can be recorded in double (rounded with precision of 15-16 significant decimal digits), is from ±5.0 × 10-324 to ±1.7 × 10308.
float floatPI = 3.14f;
Console.WriteLine(floatPI); // 3.14
double doublePI = 3.14;
Console.WriteLine(doublePI); // 3.14
double nan = Double.NaN;
Console.WriteLine(nan); // NaN
double infinity = Double.PositiveInfinity;
Console.WriteLine(infinity); // Infinity
Boolean Type
Boolean type is declared with the keyword bool. It has two possible values: true and false. Its default value is false. It is used most often to store the calculation result of logical expressions.
int a = 1;
int b = 2;
// Which one is greater?
bool greaterAB = (a > b);
// Is 'a' equal to 1? bool equalA1 = (a == 1);
// Print the results on the console
if (greaterAB)
{
Console.WriteLine("A > B");
}
else
{
Console.WriteLine("A <= B");
}
Console.WriteLine("greaterAB = " + greaterAB);
Console.WriteLine("equalA1 = " + equalA1);
// Console output: // A <= B // greaterAB = False // equalA1 = True
Characters
In C#, characters are not 8-bit quantities like they are in many other computer languages, such as C++. Instead, C# uses a 16-bit character type called Unicode. Unicode defines a character set that is large enough to represent all of the characters found in all human languages. Although many languages, such as English, French, and German, use relatively small alphabets, some languages, such as Chinese, use very large character sets that cannot be represented using just 8 bits. To address this situation, in C#, char is an unsigned 16-bit type having a range of 0 to 65,535. The standard 8-bit ASCII character set is a subset of Unicode and ranges from 0 to 127. Thus, the ASCII characters are still valid C# characters.
// Declare a variable
char ch = 'a';
// Print the results on the console
Console.WriteLine( "The code of '" + ch + "' is: " + (int)ch);
ch = 'b';
Console.WriteLine( "The code of '" + ch + "' is: " + (int)ch);
ch = 'A';
Console.WriteLine( "The code of '" + ch + "' is: " + (int)ch);
// Console output:
// The code of 'a' is: 97
// The code of 'b' is: 98
// The code of 'A' is: 65
Strings
Strings are sequences of characters. In C# they are declared by the keyword string. Their default value is null. Strings are enclosed in quotation marks. Various text-processing operations can be performed using strings: concatenation (joining one string with another), splitting by a given separator, searching, replacement of characters and others. The string type is an alias for the System.String class. It is derived from object type.
// Declare some variables
string firstName = "John";
string lastName = "Smith";
string fullName = firstName + " " + lastName;
// Print the results on the console
Console.WriteLine("Hello, " + firstName + "!");
Console.WriteLine("Your full name is " + fullName + ".");
// Console output:
// Hello, John!
// Your full name is John Smith.
Object Type
Object type is a special type, which is the parent of all other types in the .NET Framework. Declared with the keyword object, it can take values from any other type. It is a reference type, i.e. an index (address) of a memory area which stores the actual value.
// Declare some variables
object container1 = 5;
object container2 = "Five";
// Print the results on the console
Console.WriteLine("The value of container1 is: " + container1);
Console.WriteLine("The value of container2 is: " + container2);
// Console output:
// The value of container1 is: 5
// The value of container2 is: Five.
Pointer Type
Pointer type variables store the memory address of another type. Pointers in C# have the same capabilities as the pointers in C or C++.
Syntax for declaring a pointer type is −
type* identifier;
For example,
char* cptr; int* iptr;