Change password in Laravel 8

In this article, we will learn about changing passwords in Laravel 8, don’t be confused with forgetting passwords and changing passwords. Change password happens when the user’s logged in and wants’s to update or change his password.
The user uses the forgotten password when he is unable to log in with the password he remembered.
Earlier I was applying the same change password concept in one of my projects, I have gone through many blogs, but I didn’t find the simplest way to achieve the change password in laravel 8. Some of them use the validation rule to do that thing.
I will show you the simplest way to change passwords in Laravel 8, so let’s begin the journey.
To achieve change password follow below steps
1.Write your form code in the blade file
Below is the code which I have written in my blade file, to show validation messsage error i have use.
@error('current_password') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror
<form action="{{route('profile.change.password')}}" method="post" class="needs-validation" novalidate enctype="multipart/form-data"> @csrf <div class="row "> <div class="col-md-12"> <div class="main-card mb-3 card"> <div class="card-body"> <h4 class="card-title"> <h4>Change Password</h4> </h4> <div class="row"> <div class="col-md-4"> <div class="form-group mt-3"> <label for="current_password">Old password</label> <input type="password" name="current_password" class="form-control @error('current_password') is-invalid @enderror" required placeholder="Enter current password"> @error('current_password') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="col-md-4"> <div class="form-group mt-3"> <label for="new_password ">new password</label> <input type="password" name="password" class="form-control @error('password') is-invalid @enderror" required placeholder="Enter the new password"> @error('password') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="col-md-4"> <div class="form-group mt-3"> <label for="confirm_password">confirm password</label> <input type="password" name="confirm_password" class="form-control @error('confirm_password') is-invalid @enderror"required placeholder="Enter same password"> @error('confirm_password') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="d-flex justify-content-first mt-4 ml-2"> <button type="submit" class="btn btn-primary" id="formSubmit">change password</button> </div> </div> </div> </div> </div> </div> </form>
2.Create routes for change password function
Route::post('/change/password', [ChangePasswordController::class,'changePassword'])->name('profile.change.password');
3. Code for change password in controller
/** * Change the current password * @param Request $request * @return Renderable */ public function changePassword(Request $request) { $user = Auth::user(); $userPassword = $user->password; $request->validate([ 'current_password' => 'required', 'password' => 'required|same:confirm_password|min:6', 'confirm_password' => 'required', ]); if (!Hash::check($request->current_password, $userPassword)) { return back()->withErrors(['current_password'=>'password not match']); } $user->password = Hash::make($request->password); $user->save(); return redirect()->back()->with('success','password successfully updated'); }
Below images showing how it will show in the frontend
Case 1: when new password and confirm password not match
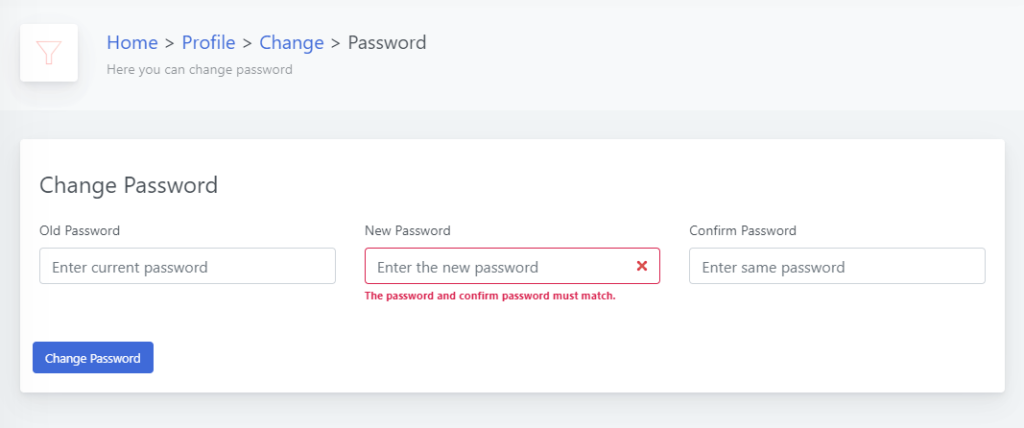
Case 2: When your current password does not match
