How to add columns to the existing table in Laravel through migration

Well, we all know how Laravel Migration feature help us to maintain database related to our modules and packages.
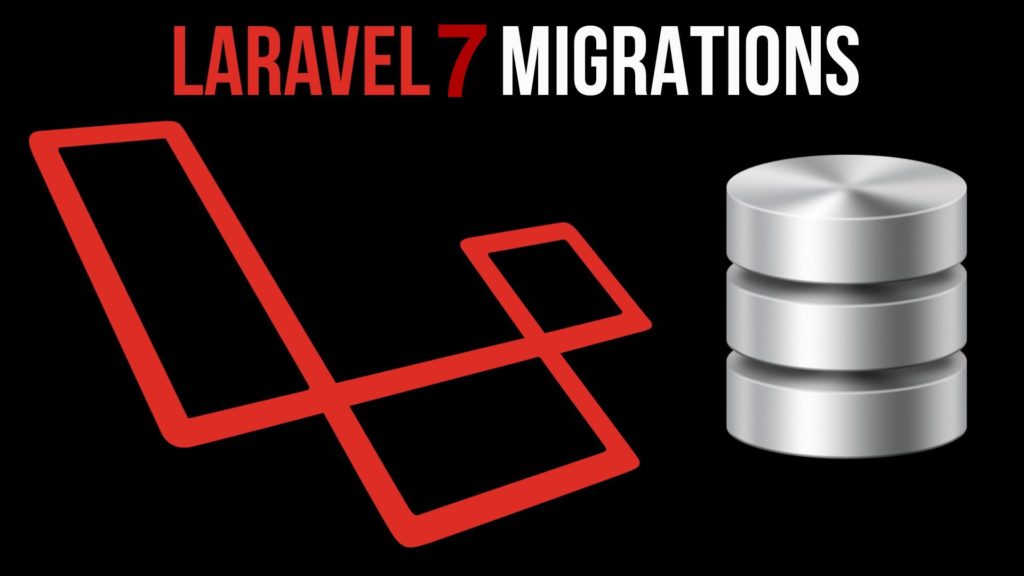
Before going to start I want to say what Laravel Migration is, Laravel migration is a way that allows you to create a table in your database by just running php artisan:migrate
in your command line.If you need more information regarding migration you can visit here https://laravel.com/docs/7.x/migrations.But the issue arises when you want to add some more columns in your existing table through migration.
Add single column through migration
So, let’s see what to do if you just want to add a single column in your existing table through migration. Suppose you already have a users table in which you have been migrated and you want to add a single column in it.Just run the below command.
php artisan make:migration add_profile_to_users
It will create a file 2020_05_30_082632_add_profile_to_users.php under database/migration go open the file you can find code something like below
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class AddProfileToUsers extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('users', function (Blueprint $table) {
$table->string('proflie')->nullable;
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('users', function (Blueprint $table) {
$table->dropColumn(['profile']);
});
}
}
Note: Somehow if you didn’t find any column name after the migration command you can add it by your own.
Add multiple columns through migration
If you want to add more than one column in your existing table in Laravel through migration then just run the below command
php artisan make:migration add_multiple_column_to_users
It will create a file 2020_05_30_082633_add_multiple_column_to_users.php under database/migration go open the file you can find code with no column you can as much as the column you want to add in the existing table.
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class AddMultipleColumnToUsers extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('users', function (Blueprint $table) {
$table->string('phone')->nullable;
$table->string('image')->nullable;
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('users', function (Blueprint $table) {
$table->dropColumn(['phone','image']);
});
}
}
Available Column Types
The schema builder contains a variety of column types that you may specify when building your tables:
Command | Description |
---|---|
$table->id(); | Alias of $table->bigIncrements('id') . |
$table->foreignId('user_id'); | Alias of $table->unsignedBigInteger('user_id') . |
$table->bigIncrements('id'); | Auto-incrementing UNSIGNED BIGINT (primary key) equivalent column. |
$table->bigInteger('votes'); | BIGINT equivalent column. |
$table->binary('data'); | BLOB equivalent column. |
$table->boolean('confirmed'); | BOOLEAN equivalent column. |
$table->char('name', 100); | CHAR equivalent column with a length. |
$table->date('created_at'); | DATE equivalent column. |
$table->dateTime('created_at', 0); | DATETIME equivalent column with precision (total digits). |
$table->dateTimeTz('created_at', 0); | DATETIME (with timezone) equivalent column with precision (total digits). |
$table->decimal('amount', 8, 2); | DECIMAL equivalent column with precision (total digits) and scale (decimal digits). |
$table->double('amount', 8, 2); | DOUBLE equivalent column with precision (total digits) and scale (decimal digits). |
$table->enum('level', ['easy', 'hard']); | ENUM equivalent column. |
$table->float('amount', 8, 2); | FLOAT equivalent column with a precision (total digits) and scale (decimal digits). |
$table->geometry('positions'); | GEOMETRY equivalent column. |
$table->geometryCollection('positions'); | GEOMETRYCOLLECTION equivalent column. |
$table->increments('id'); | Auto-incrementing UNSIGNED INTEGER (primary key) equivalent column. |
$table->integer('votes'); | INTEGER equivalent column. |
$table->ipAddress('visitor'); | IP address equivalent column. |
$table->json('options'); | JSON equivalent column. |
$table->jsonb('options'); | JSONB equivalent column. |
$table->lineString('positions'); | LINESTRING equivalent column. |
$table->longText('description'); | LONGTEXT equivalent column. |
$table->macAddress('device'); | MAC address equivalent column. |
$table->mediumIncrements('id'); | Auto-incrementing UNSIGNED MEDIUMINT (primary key) equivalent column. |
$table->mediumInteger('votes'); | MEDIUMINT equivalent column. |
$table->mediumText('description'); | MEDIUMTEXT equivalent column. |
$table->morphs('taggable'); | Adds taggable_id UNSIGNED BIGINT and taggable_type VARCHAR equivalent columns. |
$table->uuidMorphs('taggable'); | Adds taggable_id CHAR(36) and taggable_type VARCHAR(255) UUID equivalent columns. |
$table->multiLineString('positions'); | MULTILINESTRING equivalent column. |
$table->multiPoint('positions'); | MULTIPOINT equivalent column. |
$table->multiPolygon('positions'); | MULTIPOLYGON equivalent column. |
$table->nullableMorphs('taggable'); | Adds nullable versions of morphs() columns. |
$table->nullableUuidMorphs('taggable'); | Adds nullable versions of uuidMorphs() columns. |
$table->nullableTimestamps(0); | Alias of timestamps() method. |
$table->point('position'); | POINT equivalent column. |
$table->polygon('positions'); | POLYGON equivalent column. |
$table->rememberToken(); | Adds a nullable remember_token VARCHAR(100) equivalent column. |
$table->set('flavors', ['strawberry', 'vanilla']); | SET equivalent column. |
$table->smallIncrements('id'); | Auto-incrementing UNSIGNED SMALLINT (primary key) equivalent column. |
$table->smallInteger('votes'); | SMALLINT equivalent column. |
$table->softDeletes('deleted_at', 0); | Adds a nullable deleted_at TIMESTAMP equivalent column for soft deletes with precision (total digits). |
$table->softDeletesTz('deleted_at', 0); | Adds a nullable deleted_at TIMESTAMP (with timezone) equivalent column for soft deletes with precision (total digits). |
$table->string('name', 100); | VARCHAR equivalent column with a length. |
$table->text('description'); | TEXT equivalent column. |
$table->time('sunrise', 0); | TIME equivalent column with precision (total digits). |
$table->timeTz('sunrise', 0); | TIME (with timezone) equivalent column with precision (total digits). |
$table->timestamp('added_on', 0); | TIMESTAMP equivalent column with precision (total digits). |
$table->timestampTz('added_on', 0); | TIMESTAMP (with timezone) equivalent column with precision (total digits). |
$table->timestamps(0); | Adds nullable created_at and updated_at TIMESTAMP equivalent columns with precision (total digits). |
$table->timestampsTz(0); | Adds nullable created_at and updated_at TIMESTAMP (with timezone) equivalent columns with precision (total digits). |
$table->tinyIncrements('id'); | Auto-incrementing UNSIGNED TINYINT (primary key) equivalent column. |
$table->tinyInteger('votes'); | TINYINT equivalent column. |
$table->unsignedBigInteger('votes'); | UNSIGNED BIGINT equivalent column. |
$table->unsignedDecimal('amount', 8, 2); | UNSIGNED DECIMAL equivalent column with a precision (total digits) and scale (decimal digits). |
$table->unsignedInteger('votes'); | UNSIGNED INTEGER equivalent column. |
$table->unsignedMediumInteger('votes'); | UNSIGNED MEDIUMINT equivalent column. |
$table->unsignedSmallInteger('votes'); | UNSIGNED SMALLINT equivalent column. |
$table->unsignedTinyInteger('votes'); | UNSIGNED TINYINT equivalent column. |
$table->uuid('id'); | UUID equivalent column. |
$table->year('birth_year'); | YEAR equivalent column. |
I hope the above blog post help you to solve your issue, if your issue still doesn’t resolve ping us, we are here to help you.You can also read our other post on laravel here https://blog.codehunger.in/category/laravel/