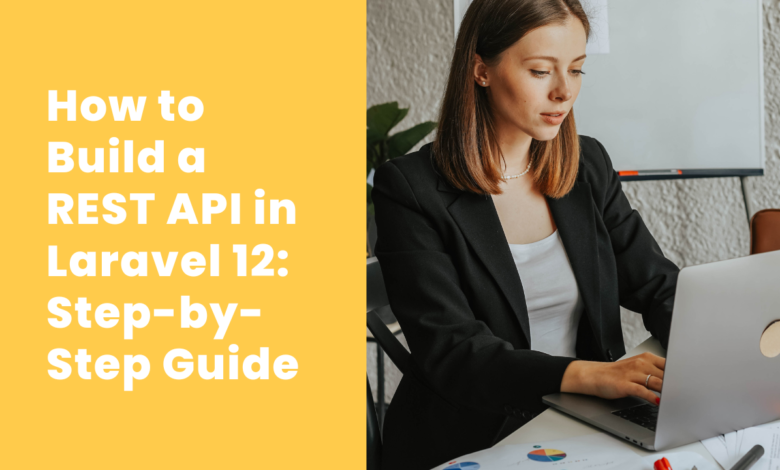
Introduction
Thinking about creating a REST API using Laravel 12 but not sure where to start? You’re in the right place! Laravel has always made API development easy, but with version 12, things have become even more streamlined and developer-friendly. Whether you’re a beginner or someone with a bit of coding experience, this step-by-step guide will walk you through building a functional REST API with Laravel 12 in no time. Let’s dive in together!
Step 1: Install Laravel 12
Create a New Laravel Project
First things first, make sure you have Composer installed on your system. Then, open your terminal and run the following command:
composer create-project laravel/laravel laravel-api
This command will create a fresh Laravel 12 project named laravel-api
.
Step 2: Set Up the Database
Configure .env
File
Once your project is ready, head over to the .env
file and update the database credentials:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database
DB_USERNAME=your_username
DB_PASSWORD=your_password
Make sure your MySQL server is running and the database you entered exists.
Step 3: Create a Model and Migration
Let’s say you want to build an API for managing posts.
Run Artisan Command
php artisan make:model Post -m
This command creates a Post
model and its corresponding migration file.
Define Table Structure
Open the migration file in database/migrations
and add the following:
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
Now run:
php artisan migrate
Step 4: Create a Controller
Use API Resource Controller
Run this command:
php artisan make:controller Api/PostController --api
This creates a controller with default methods for RESTful APIs like index
, store
, show
, update
, and destroy
.
Step 5: Define API Routes
Open the file routes/api.php
and add:
use App\Http\Controllers\Api\PostController;
Route::apiResource('posts', PostController::class);
This will automatically create all the routes needed for a standard CRUD API.
Step 6: Build the Controller Methods
Inside your PostController
, add logic to each method. Here’s a quick setup:
use App\Models\Post;
use Illuminate\Http\Request;
public function index()
{
return response()->json(Post::all(), 200);
}
public function store(Request $request)
{
$request->validate([
'title' => 'required',
'content' => 'required',
]);
$post = Post::create($request->all());
return response()->json($post, 201);
}
public function show($id)
{
$post = Post::findOrFail($id);
return response()->json($post);
}
public function update(Request $request, $id)
{
$post = Post::findOrFail($id);
$post->update($request->all());
return response()->json($post, 200);
}
public function destroy($id)
{
Post::findOrFail($id)->delete();
return response()->json(null, 204);
}
Step 7: Allow Mass Assignment
In Post.php
model, allow mass assignment by adding:
protected $fillable = ['title', 'content'];
Step 8: Test Your API
You can now test your API using tools like Postman or Insomnia. Try creating, reading, updating, and deleting posts using your new endpoints:
GET /api/posts
POST /api/posts
GET /api/posts/{id}
PUT /api/posts/{id}
DELETE /api/posts/{id}
And there you have it! You’ve successfully built a fully functional REST API using Laravel 12. Laravel makes it incredibly easy to set up clean and scalable APIs, perfect for powering your mobile apps, frontend frameworks like React or Vue, or even microservices.
If you’re looking to take your Laravel skills to the next level or want help with custom web development, CodeHunger is a great place to start. Their expert team helps individuals and businesses build high-quality Laravel applications with clean code and strong architecture.