jQuery
How to get selected checkbox value using jquery
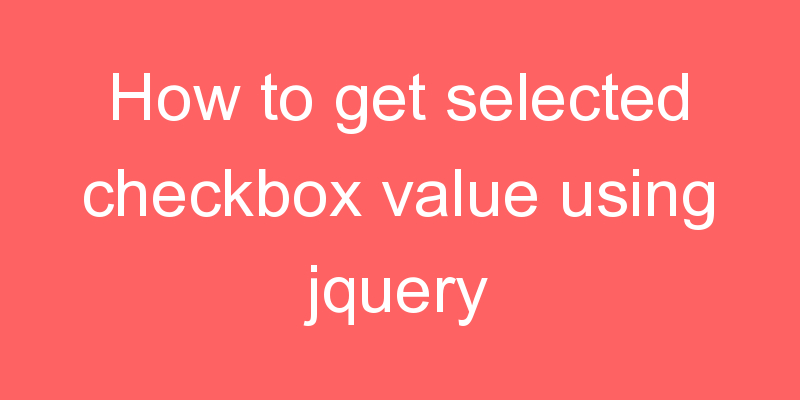
Today I will show you how we can get the value of selected checkbox using jquery, In day to day programming, we need those types of things like to get the value from checkbox and save it into our database using ajax. To achieve that thing I am going to use input[type=checkbox]:checked
with foreach because we have multiple checkboxes, whenever you click on your checkbox we store it in an array.
Create a file, for example, code.html and put the below code in it.
<!DOCTYPE html>
<html>
<head>
<title>Get selected checkbox value from checkboxlist in Jquery - CodeHunger</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
</head>
<body>
<form>
<input id="movie1" type="checkbox" value="avenger"/><label for="avenger">Avenger</label><br>
<input id="movie1" type="checkbox" value="baaghi3"/><label for="baaghi3">Baaghi3</label><br>
<input id="movie1" type="checkbox" value="jumanji"/><label for="jumanji">Jumanji</label><br>
<input id="movie1" type="checkbox" value="tubelight"/><label for="tubelight">Tubelight</label><br>
<input id="movie1" type="checkbox" value="vatan"/><label for="vatan">Vatan</label><br>
<input type="button" class="btnClick" value="Click Me" />
</form>
</body>
<script type="text/javascript">
$(function () {
$(".btnClick").click(function () {
var selected = new Array();
$("input[type=checkbox]:checked").each(function () {
selected.push(this.value); // pushing selected value in array
});
if (selected.length > 0) { // check length if no box is selected then alert will be not shown
alert("Selected values: " + selected.join(","));
}
});
});
</script>
</html>
Still having confusion feel free to ask, we are here to help you.