How to Use Amazon FIFO SQS in Laravel queue?
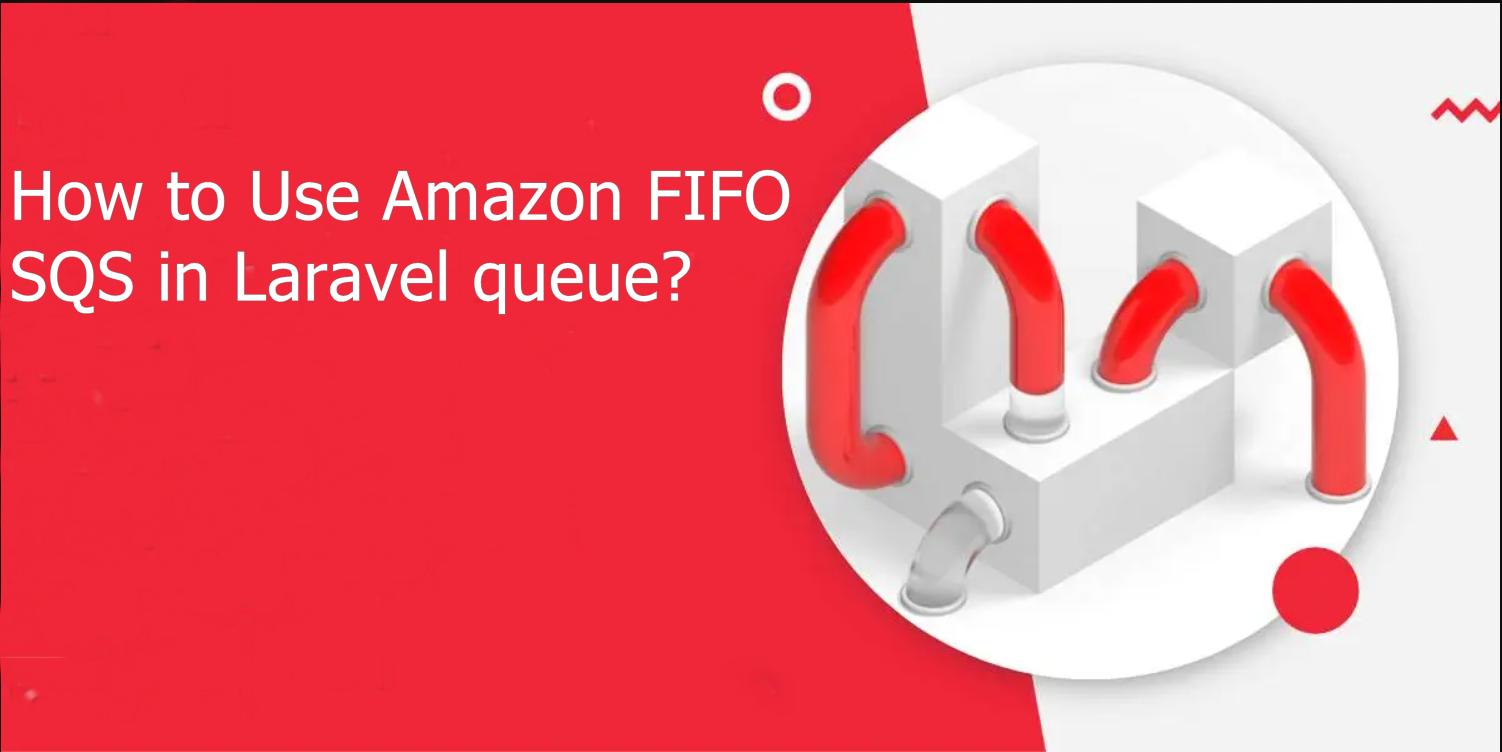
I want to point out to others who might stumble across the same issue that, although editing SqsQueue.php
works, it will easily be reset by a composer install
or composer update
. An alternative is to implement a new Illuminate\Queue\Connectors\ConnectorInterface
for SQS FIFO then add it to Laravel’s queue manager.
My approach is as follows:
- Create a new
SqsFifoQueue
class that extendsIlluminate\Queue\SqsQueue
but supports SQS FIFO. - Create a new
SqsFifoConnector
class that extendsIlluminate\Queue\Connectors\SqsConnector
that would establish a connection usingSqsFifoQueue
. - Create a new
SqsFifoServiceProvider
that registers theSqsFifoConnector
to Laravel’s queue manager. - Add
SqsFifoServiceProvider
to yourconfig/app.php
. - Update
config/queue.php
to use the new SQS FIFO Queue driver.
Example:
- Create a new
SqsFifoQueue
class that extendsIlluminate\Queue\SqsQueue
but supports SQS FIFO.
<?php class SqsFifoQueue extends \Illuminate\Queue\SqsQueue { public function pushRaw($payload, $queue = null, array $options = []) { $response = $this->sqs->sendMessage([ 'QueueUrl' => $this->getQueue($queue), 'MessageBody' => $payload, 'MessageGroupId' => uniqid(), 'MessageDeduplicationId' => uniqid(), ]); return $response->get('MessageId'); } }
2. Create a new SqsFifoConnector
class that extends Illuminate\Queue\Connectors\SqsConnector
that would establish a connection using SqsFifoQueue
.
<?php use Aws\Sqs\SqsClient; use Illuminate\Support\Arr; class SqsFifoConnector extends \Illuminate\Queue\Connectors\SqsConnector { public function connect(array $config) { $config = $this->getDefaultConfiguration($config); if ($config['key'] && $config['secret']) { $config['credentials'] = Arr::only($config, ['key', 'secret']); } return new SqsFifoQueue( new SqsClient($config), $config['queue'], Arr::get($config, 'prefix', '') ); } }
3. Create a new SqsFifoServiceProvider
that registers the SqsFifoConnector
to Laravel’s queue manager.
<?php class SqsFifoServiceProvider extends \Illuminate\Support\ServiceProvider { public function register() { $this->app->afterResolving('queue', function ($manager) { $manager->addConnector('sqsfifo', function () { return new SqsFifoConnector; }); }); } }
4. Add SqsFifoServiceProvider
to your config/app.php
.
<?php return [ 'providers' => [ ... SqsFifoServiceProvider::class, ], ];
5. Update config/queue.php
to use the new SQS FIFO Queue driver.
<?php return [ 'default' => 'sqsfifo', 'connections' => [ 'sqsfifo' => [ 'driver' => 'sqsfifo', 'key' => 'my_key' 'secret' => 'my_secret', 'queue' => 'my_queue_url', 'region' => 'my_sqs_region', ], ], ];
Then your queue should now support SQS FIFO Queues.