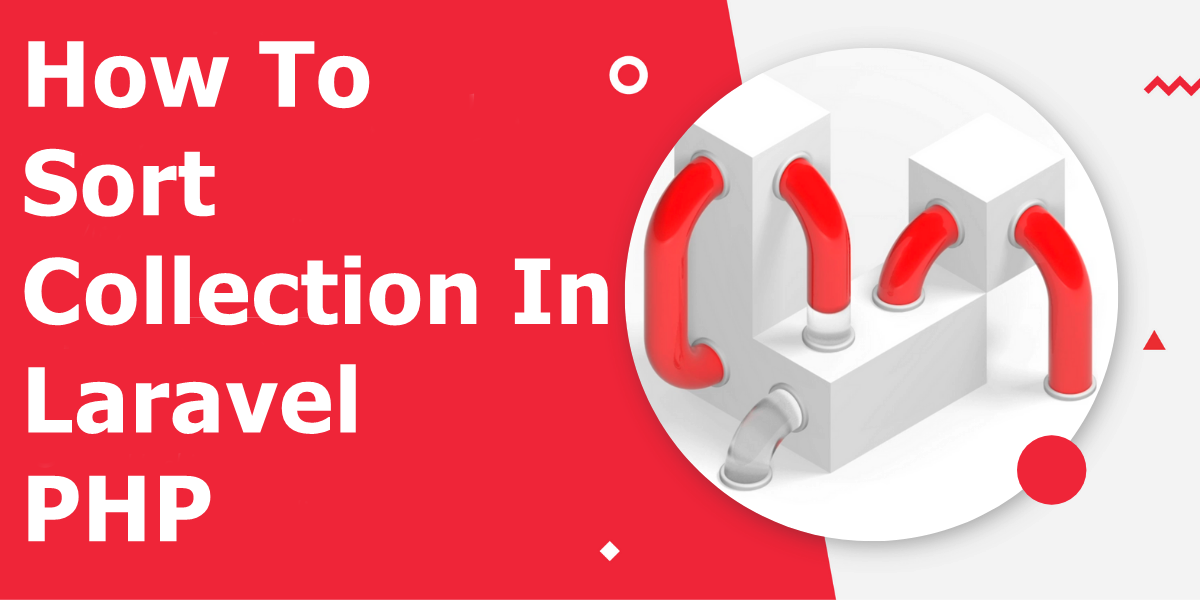
You can use sortBy for laravel collection for sorting by custom orders.
You can do like this:
$collection = collect($array); $order = [1,2,3,4,5]; $collection->sortBy(function($model) use ($order){ return array_search($model->order_id, $order); }
Also, you could add an attribute accessor to your model which does the same.
public function getSortedItemsAttribute() { if ( ! is_null($this->item_order)) { $order = $this->item_order; $list = $this->items->sortBy(function($model) use ($order){ return array_search($model->getKey(), $order); }); return $list; } return $this->items; }
foreach ($list->sortedItems as $item) { // More Code Here }
If you need this sort of functionality in multiple places I suggest you create your own Collection class.
class MyCollection extends Illuminate\Database\Eloquent\Collection { public function sortByIds(array $ids){ return $this->sortBy(function($model) use ($ids){ return array_search($model->getKey(), $ids); } } }
Then, to actually use that class override newCollection()
in your model. In this case it would be in the ChecklistItems
class:
You can also read: How to use usort in laravel with example
public function newCollection(array $models = array()) { return new MyCollection($models); }
I hope it will help you, feel free to comment if you have any question.