Laravel Tips and Tricks 2022
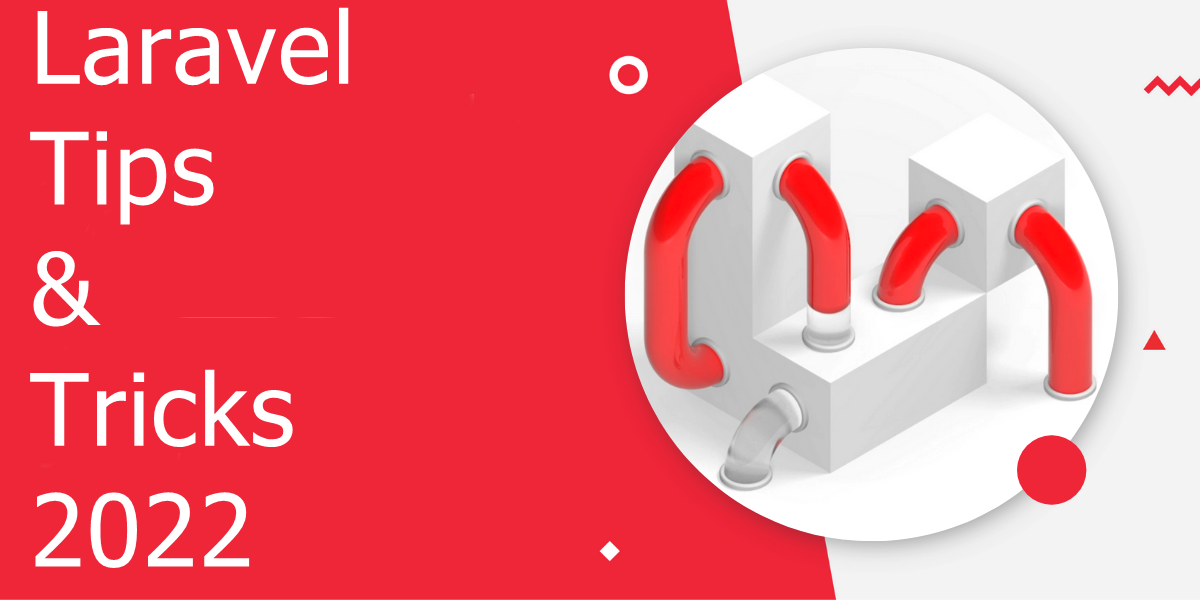
In this article, we will learn about laravel Tips and tricks what are the new things announced for the laravel in 2022, I hope you will lot of useful information after reading the entire post.
Before moving forward let’s know what laravel is
Laravel is an open-source PHP framework, which is robust and straightforward to understand. It is used for building a wide range of custom web applications. It supports a model-view-controller design pattern.
If you are old to laravel then you know that laravel has some great features like dependency injection, queues, and scheduled jobs, and expressive database abstraction layer, unit, and integration testing, and many more.
We will read about the below features in this blog post
- How to install laravel older version
- Simple Pagination
- How to send notification to anyone
- Use @auth in blade file
- Check the view file exists
- Create migration without underscore _ symbol
- No timestamp columns
- Soft-deletes: multiple restore
- Change Default Timestamp Fields
- Column name change
How to install laravel older version
If you want to use the older Laravel version instead of the newest Laravel, You can do this by running this command:
composer create-project --prefer-dist laravel/laravel project "7.*"
Simple Pagination
In pagination, if you need just “Previous/next” links instead of all the page numbers (and have fewer DB queries because of that), You can do this easily by changing paginate() to simplePaginate():
// Instead of
$products = Product::paginate(8);
// Now you can do this
$products = Product::simplePaginate(8);
How to send notification to anyone
You can send Laravel Notifications not only to a specific user with $user->notify()
, but also to anyone you want, via Notification::route(), with so-called “on-demand” notifications:
Notification::route('mail', 'Jhon@gmail.com')
->route('test', '123456')
->route('slack', 'https://hooks.slack.com/services/...')
->notify(new InvoicePaid($invoice));
use @auth in blade file
Instead of an if-statement to check logged-in users, You can use the @auth directive in your blade file.
@auth
// The user is authenticated.
@endauth
@guest
// The user is not authenticated.
@endguest
Check the view file exist
You can check if the View file exists before actually loading it.
if (view()->exists('admin.dashboard')) {
// Load the view
}
You can even load an array of views, and only the first existing will be loaded.
return view()->first(['admin.dashboard', 'dashboard'], $data);
Create migration without underscore _ symbol
When you are running a make:migration
command, you don’t necessarily have to write underscore _ symbol between parts, like create_users_table.
// with underscore _ symbol
php artisan make:migration create_users_table
You can enter the name into quotes and then utilize spaces instead of underscores.
// without underscore _ symbol
php artisan make:migration "create users table"
No timestamp columns
If your DB table doesn’t contain timestamp fields created_at
and updated_at
, you can specify that Eloquent model wouldn’t use them, with $timestamps = false
property.
class Company extends Model
{
public $timestamps = false;
}
Soft-deletes: multiple restore
When using soft-deletes, you can restore multiple rows in one sentence.
Post::onlyTrashed()->where('author_id', 1)->restore();
Change Default Timestamp Fields
class Role extends Model
{
const CREATED_AT = 'create_time';
const UPDATED_AT = 'update_time';
}
Column Name Change
$users = DB::table('users')->select('name', 'email as user_email')->get();
I hope you learn a lot from this blog, keep sharing this post with your friends and rate me 5, I believe in sharing is caring.