React Native – State

There are two types of data that control a component: props and state. props are set by the parent and they are fixed throughout the lifetime of a component. For data that is going to change, we have to use state.
In general, you should initialize state in the constructor, and then call setState when you want to change it.
state : is mutable. This means that state can be updated in the future while props can’t. we can initialize state in the constructor, and then call setState when we want to change it.
We can change our state by handling the Events in React Native Application.
“State” is used for data that is going to change. Its role is very similar to properties for a class in OOP. That is why it is only for class components.
The Basic Features of State
- “State” is a JS Object which contains data related to the component
- Updating “state” causes the component to rerender
- “State” must be initialized when the component is created
- “State” can only be updated by
setState()
method
In this example, we create a Text component with state data. The content of Text component will be updated by clicking on it. The event onPress call the setState, and it updates the state “myState” text.
import React, {Component} from 'react';
import { Text, View } from 'react-native';
export default class App extends Component {
state = {
myState: 'This is a text component, created using state data. It will change or updated on clicking it.'
}
updateState = () => this.setState({myState: 'The state is updated'})
render() {
return (
<View>
<Text onPress={this.updateState}> {this.state.myState} </Text>
</View>
);
}
}

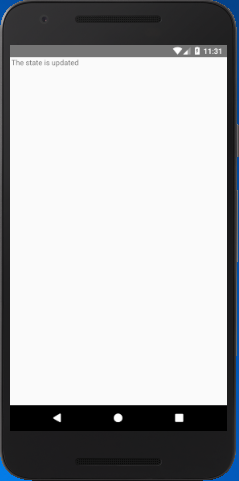
Handling events with React elements is very similar to handling events on DOM elements. There are some syntactic differences:
React events are named using camelCase, rather than lowercase.
With JSX you pass a function as the event handler, rather than a string.
For example, the HTML:
<button onclick="activateLasers()">
Activate Lasers
</button>
is slightly different in React:
<button onClick={activateLasers}>
Activate Lasers
</button>
Keep in mind not to update state directly using this.state. Always use setState to update the state objects. Using setState re-renders the component and all the child components. This is great, because you don’t have to worry about writing event handlers like other languages.