React Props

The simplest way to explain props would be to say that they function similarly to HTML attributes. In other words, props provide configuration values for the component. For example, in the code below a Badge
component is created and it is expecting a ‘name’ prop to be sent when the component is instantiated.
“All the user needs to do is, change the parent component’s state, while the changes are passed down to the child component through props.”
Props is a shorthand for properties (You guessed it right!). React uses ‘props’ to pass attributes from ‘parent’ component to ‘child’ component.
“Props” is a special keyword in React, which stands for properties and is being used for passing data from one component to another.
But the important part here is that data with props are being passed in a uni-directional flow. (one way from parent to child)
Furthermore, props data is read-only, which means that data coming from the parent should not be changed by child components.
Props are the argument passed to the function or component which is ultimately processed by React. Let me illustrate this with an example.
App.js Code
import React, { Component } from 'react';
class App extends React.Component {
render() {
return (
<div>
<h1> Welcome to { this.props.name } </h1>
</div>
);
}
}
export default App;
index.js code
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
ReactDOM.render(
<React.StrictMode>
<App name = "CodeHunger!!" />
</React.StrictMode>,
document.getElementById('root')
);
// If you want your app to work offline and load faster, you can change
// unregister() to register() below. Note this comes with some pitfalls.
// Learn more about service workers: https://bit.ly/CRA-PWA
serviceWorker.unregister();

Props in Functional Component
import React, { Component } from 'react';
class ParentComponent extends Component {
render() {
return (
<ChildComponent name="First Child" />
);
}
}
const ChildComponent = (props) => {
return <p>{props.name}</p>;
};
export default ParentComponent;
Firstly, we need to define/get some data from the parent component and assign it to a child component’s “prop” attribute.
<ChildComponent name="First Child" />
“Name” is a defined prop here and contains text data. Then we can pass data with props like we’re giving an argument to a function:
const ChildComponent = (props) => {
// statements
};
And finally, we use dot notation to access the prop data and render it:
return <p>{props.name}</p>;
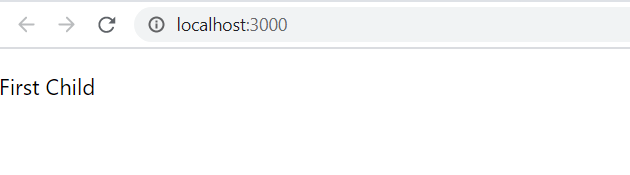