Copy Text to clipboard in vue js
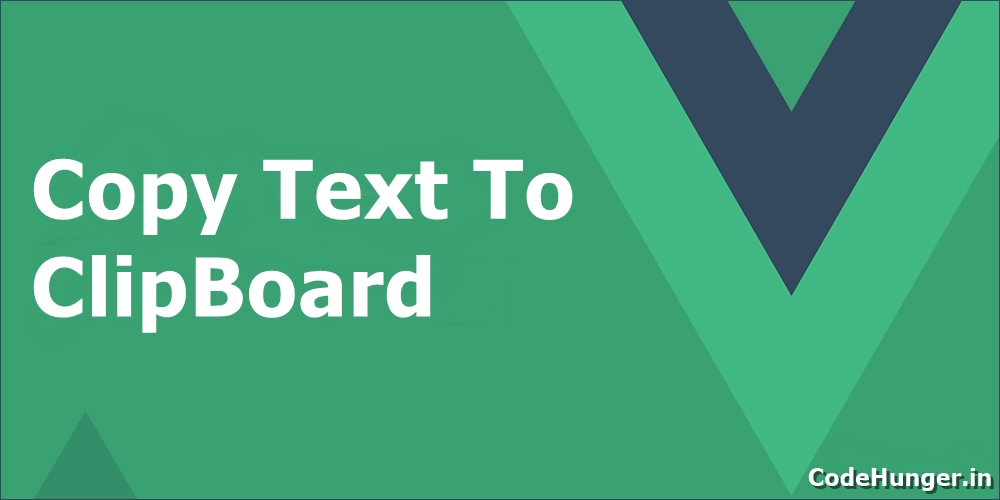
Hello buddy, I hope you are doing well in this blog we will learn about how to copy text to clipboard in vue js or javascript.
I will show you the easiest way to copy the URL or text to the clipboard in Vue js or in javascript.
To copy the text or URL in to the clipboard we will use navigator.clipboard.writeText. I have added the basic detail of navigator.clipboard below.
How navigator.clipboard works
The Clipboard API adds to the Navigator
interface the read-only clipboard
property, which returns the Clipboard
object used to read and write the clipboard’s contents.
The Clipboard API can be used to implement cut, copy, and paste features within a web application.
Use of the asynchronous clipboard read and write methods requires that the user grant the web site or app permission to access the clipboard. This permission must be obtained from the Permissions API using the "clipboard-read"
and/or "clipboard-write"
permissions.
To learn more about navigator.clipboard you can visit this URL
Implementing Navigator.clipboard in code
In this example what I am trying to do, is copy the text with the click of a button.
<template>
<button class="btn btn-primary" @click="copyURL('https://codehunger.in/')">Button</button>
</template>
Now we will implement our script code
<script>
export default {
methods: {
copyURL(url) {
/* Copy the text inside the text field */
navigator.clipboard.writeText(url);
},
}
}
</script>
So when you click on the button it will copy the www.codehunger.in URL , I hope now you understood, how easily we can copy the text in vue js or javascript.
Full code
So our entire vue js template will look like the below one
<template>
<button class="btn btn-primary" type="submit" @click="copyURL('https://codehunger.in/')">Button</button>
</template>
<script>
export default {
methods: {
copyURL(url) {
/* Copy the text inside the text field */
navigator.clipboard.writeText(url);
},
}
}
</script>
I hope now you understood how to copy text or url in vue js or javascript by using navigator.clipboard method.