Google Recaptcha v3 in Laravel without package
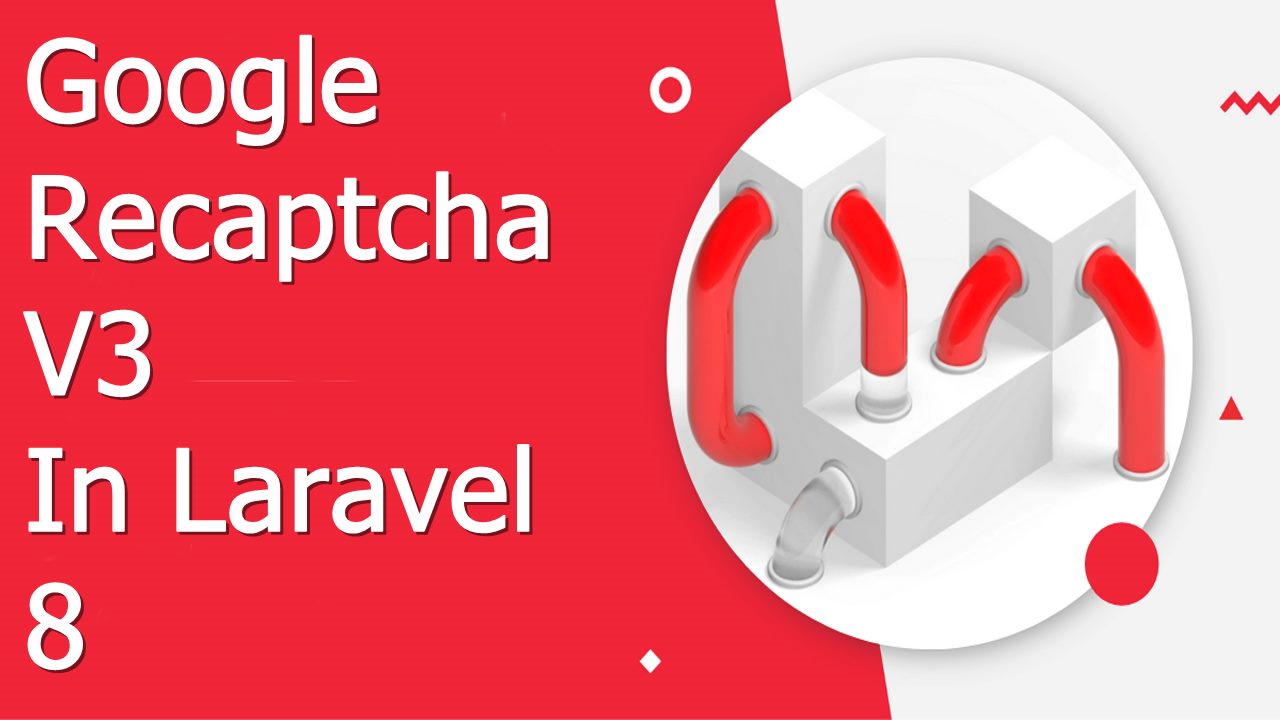
Hello everyone in this blog we will learn how we can set up google Recaptcha v3 in laravel without using any package, for your better understanding I will explain this thing with the basic example.
To enable google recaptcha follow below step
- Install Laravel Project
- Get google recaptcha site key
- Add route
- Create Controller
- Create view file
- Test the integration
The below example is based on the inquiry contact form, where I have used google Recaptcha during the submission of the form.
Step-1 Install Laravel Project
Go to your xampp/htdocs and run the below command it will create a laravel project named as example-app
composer create-project laravel/laravel example-app
Step-2 Get Google recaptcha site key
Now we need the google Recaptcha site key If you don’t have a site key and secret key then you can create from here. First click on this link: Recaptcha Admin
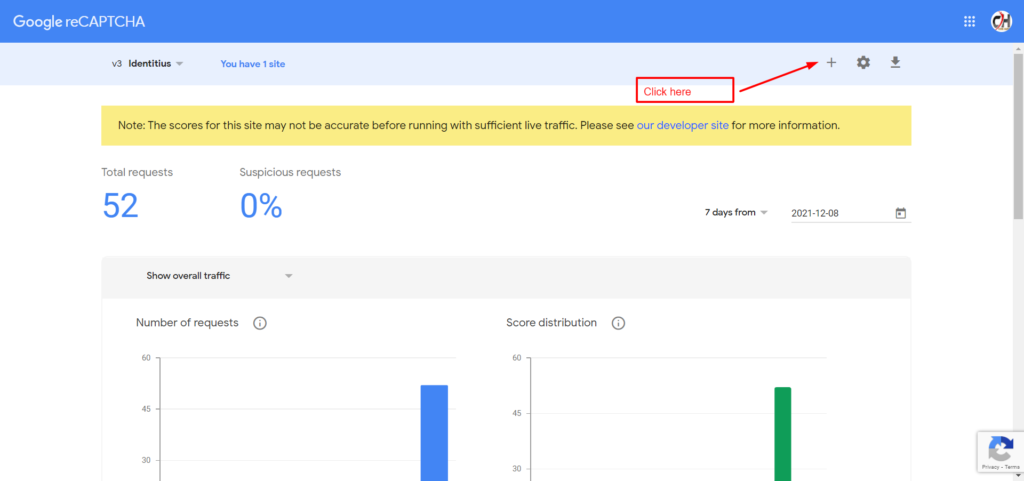
Click on the plus sign then you have the screen something like the below one.
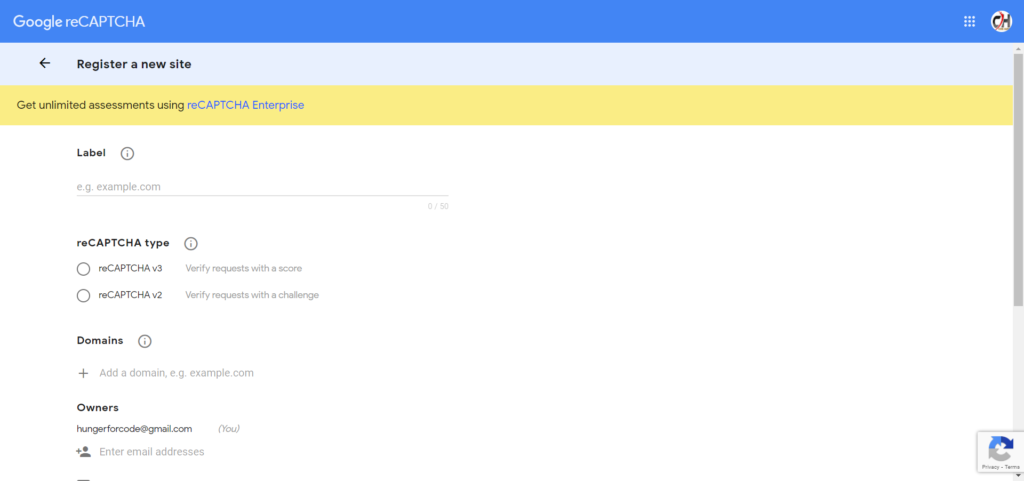
In the Label you can enter localhost as your site name, then choose recaptcha v3 add localhost as your domain name and click on save.
when you click on the save then you have a screen like the below one.
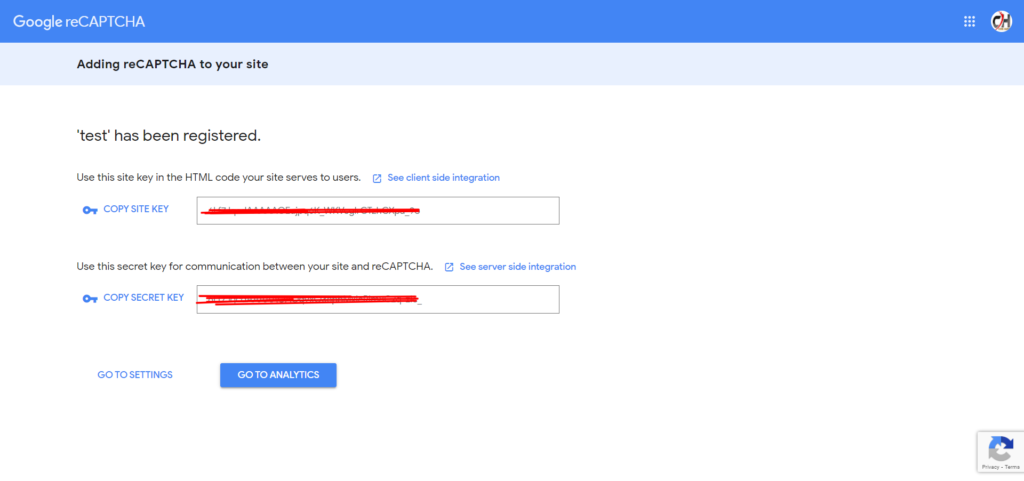
RECAPTCHA_SITE_KEY=YOur-site-key
RECAPTCHA_SECRET_KEY=your-secret-key
Step-3 Add route
Now we have to add the route, go, and the below code in your web.php
<?php
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
use App\Http\Controllers\EnquiryController;
Route::get('enquiry', [EnquiryController::class, 'index'])->name('enquiry');
Route::post('save-enquiry', [EnquiryController::class, 'sendEnquiry'])->name('save.enquiry');
Step-4 Create a controller
Now create the controller by using the below command
php artisan make:controller EnquiryController
this controller will be created inside app/http/controller now add the below code in your EnquiryController.php
<?php
namespace App\Http\Controllers\Front;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
use App\Models\Contact;
use Illuminate\Support\Facades\Http;
use Illuminate\Support\Facades\Session;
class EnquiryController extends Controller
{
/**
* Create a new controller instance.
*
* @return void
*/
public function index()
{
return view('register');
}
/**
* Store Enquiry in Contacts Table
*
*/
public function sendEnquiry(Request $request)
{
$data = $request->all();
$rules = [
'name' => 'required',
'email' => 'required|email',
'message' => 'required'
];
$validator = \Validator::make($data, $rules);
// Validate the input and return correct response
if ($validator->fails()) {
return \Response::json(array(
'success' => false,
'errors' => $validator->getMessageBag()->toArray()
), 200); // 400 being the HTTP code for an invalid request.
}
$response = Http::asForm()->post('https://www.google.com/recaptcha/api/siteverify', [
'secret' => env('RECAPTCHA_SECRET_KEY'),
'response' => $request->recaptchaToken,
]);
$recaptchaResponse = $response->json();
if ($recaptchaResponse['success'] == true) {
Contact::create($data);
$message = [
'success' => true,
];
Session::flash('contact-msg', 'Will Contact you soon');
return response()->json($message, 200);
} else {
$recaptchaFail = 'Something went wrong !';
return response()->json($recaptchaFail, 200);
}
}
}
Step-5 Create the view file
Now we will create the view file for our contact form and the below code into it.
<form id="biscolab-recaptcha-invisible-form" class="py-4" method="post">
@csrf
<div class="form-floating mb-3">
<input type="text" class="form-control name" id="name" name="name"
aria-describedby="name" placeholder="Full Name" required>
<label for="name">Full Name</label>
</div>
<div class="form-floating mb-3">
<input type="email" class="form-control email" id="email" name="email" required>
<label for="email">Email Address</label>
</div>
<div class="form-floating">
<textarea class="form-control message" name="message"
placeholder="Leave a comment here" id="contactMessage" style="height: 100px" required></textarea>
<label for="contactMessage">Comments</label>
</div>
<button class="g-recaptcha btn btn-warning my-2 float-end" data-sitekey="{{env('RECAPTCHA_SITE_KEY')}}"
data-callback='onSubmit' data-action='submit'>Submit</button>
</form>
<script>
function onSubmit(token) {
var bodyFormData = {
'name' : $('#name').val(),
'email' : $('#email').val(),
'message' : $('#contactMessage').val(),
'recaptchaToken': token,
};
axios({
method: "post",
url: "{{route('send.enquiry')}}",
data: bodyFormData,
})
.then(function (response) {
if(response.data.success == false) {
$.each(response.data.errors, function(key, value) {
$('.'+key).addClass("is-invalid");
});
} else if(response.data.success == true) {
location.reload();
}
})
.catch(function (response) {
console.log(response);
});
}
</script>
Step-6 Test the integration
To the above code, run your project in the development environment, to run the project in the development environment run php artisan serve in your command then visit the below URL in your browser.
localhost:8000
Below is my contact form image

You can see in the above image in the right corner you can see privacy terms, which means google Recaptcha v3 is added and working properly. now see what happens when i click on submit button.

When you zoom the image you can see they hit the reload URL which is the google Recaptcha v3 URL where it’s verified that the form data is not spoofed.
I hope now you understood the working principle of google Recaptcha V3, If the above blog helps you to resolve your issue please rate me 5.