
In this article I will guide you what is the difference between Laravel 7 an Laravel 8 along with I will also tell you what’s new in Laravel 7 and in the Laravel 8.
Difference Between Laravel 7 and Laravel 8, after the difference I will each point briefly.
LARAVEL 8 | LARAVEL 7 |
---|---|
app/Models Directory change | Laravel Sanctum |
New Landing Page | Custom Eloquent Casts |
Controllers Routing Namespacing | Blade Component Tags & Improvements |
Route Caching | HTTP Client |
Attributes on Extended Blade Components | Fluent String Operations |
Better Syntax for Event Listening | Route Caching Speed Improvements |
Queueable Anonymous Event Listeners | CORS Support |
Maintenance Mode | Query Time Casts |
Closure Dispatch “Catch” | Multiple Mail Drivers |
Exponential Backoff Strategy | Route Model Binding Improvements |
Let’s begin with what’s new in Laravel 8. Below are features that are released in Laravel 8
1 . app/Models Directory
-> The artisan:make model
command will create the model in the app/Models directory.
2. New Landing Page
-> Laravel 8 comes with a new landing page for a brand new install. It is now redesigned and It is built using TailwindCSS, includes light and dark-mode capabilities, and by default extends links to SaaS products and community sites.
3. Controllers Routing Namespacing
-> No more double prefix issues! In previous versions of Laravel, the RouteServiceProvider had an attribute called namespace that was used to prefix the controllers in the routes files. That created a problem when you were trying to use a callable syntax on your controllers, causing Laravel to mistakenly double prefix it for you. This attribute was removed and now you can import and use it without the issue.

4. Route Caching
-> Laravel uses route caching to compile your routes in a PHP array that is more efficient to deal with. In Laravel 8, it’s possible to use this feature even if you have closures as actions to your routes. This should extend the usage of route caching for improved performance.
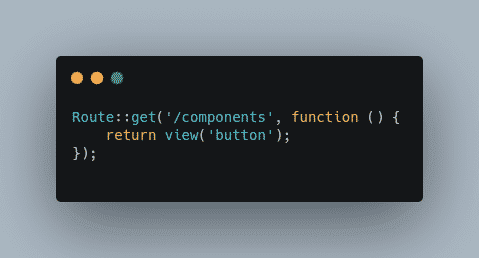
5. Attributes on Extended Blade Components
-> In Laravel 7 the child components didn’t have access to the $attributes
passed to it. In Laravel 8 these components were enhanced and it is now possible to merge nested component attributes. This makes it easier to create extended components.
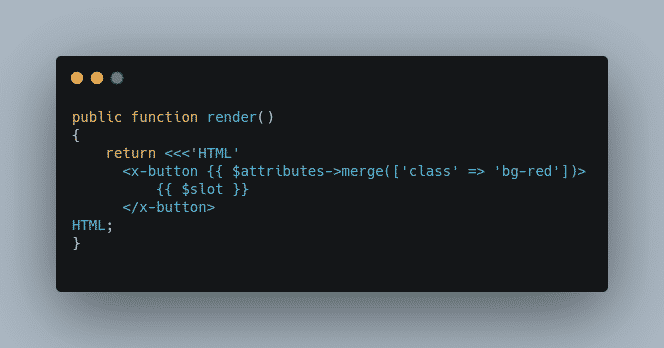
6. Better Syntax for Event Listening
-> In the previous versions of Laravel, when creating a closure based event listener there was much repetition and a cumbersome syntax.
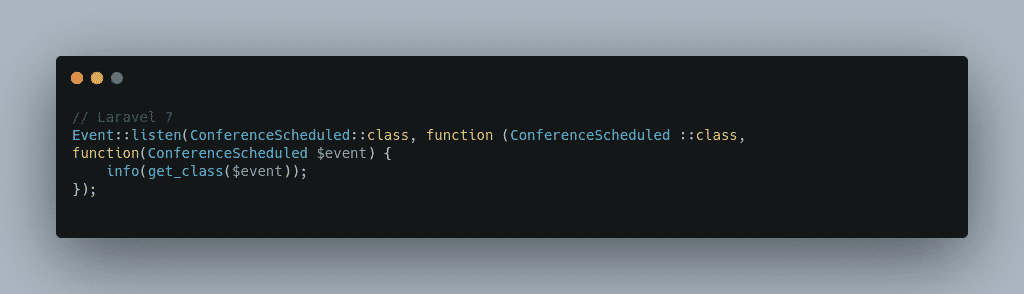
In Laravel 8 it’s simpler and cleaner:
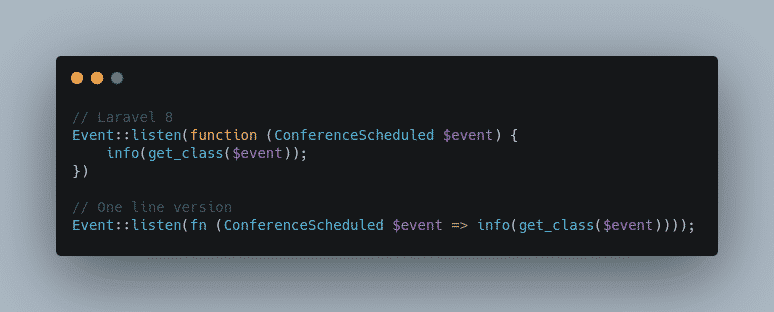
7. Queueable Anonymous Event Listeners
-> In Laravel 8 it is possible to create queueable closure from anywhere in the code. This will create a queue of anonymous event listeners that will get executed in the background. This feature makes it easier to do this, while in previous versions of Laravel you would need to use an event class and an event listener (using the ShouldQueue trait).
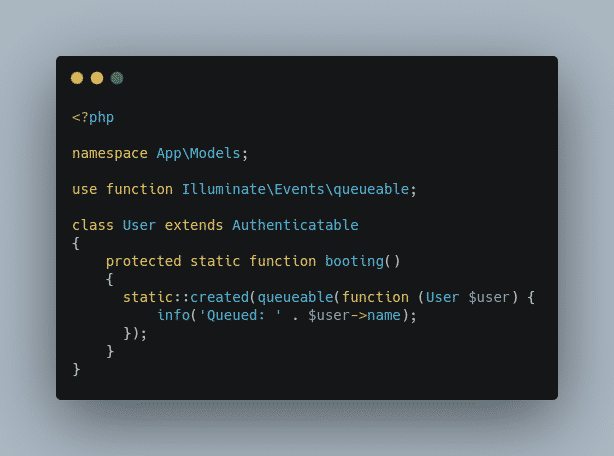
8. Maintenance Mode
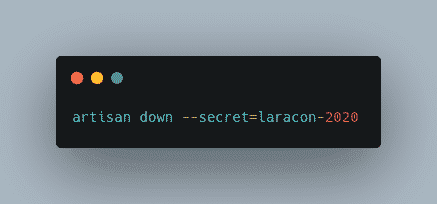
-> This is especially useful when you need to do some maintenance on your application and you want to take it down for your users but still let your developers investigate bugs. This will create a secret cookie that will be granted to whoever hits the correct endpoint, allowing it to use the application while in maintenance mode.
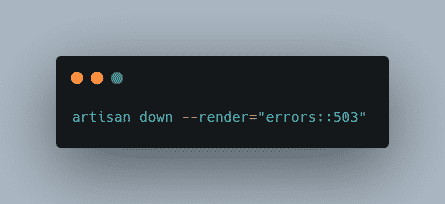
-> Pre-rendering an error view is a safe way for not exposing errors to your end user when your application is down (during a new deployment, for example). The Laravel 8 framework guarantees that your predefined error page will be displayed before everything else from the application.
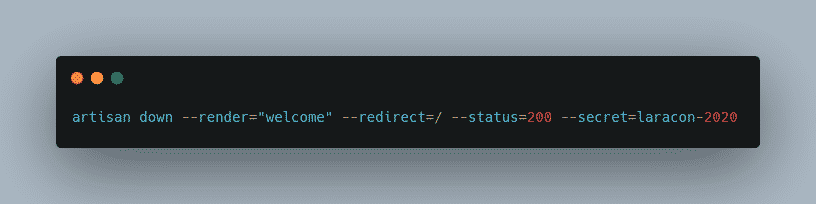
-> This combines the new features and will make the application only display a single predefined route, while still allowing for the people who have the secret to test and debug. This can be very useful when launching a new app.
9. Closure Dispatch “Catch”
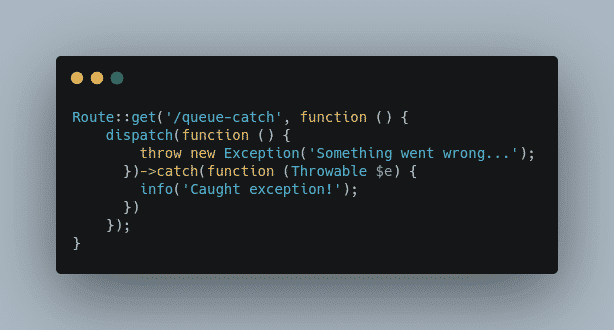
-> Laravel has a pretty robust queue system that accepts a closure queue that will get serialized and executed in the background. Now we have a way to handle failures in case your job fails.
10. Exponential Backoff Strategy
-> This is an algorithm that decreases the rate of your job in order to gradually find an acceptable rate.
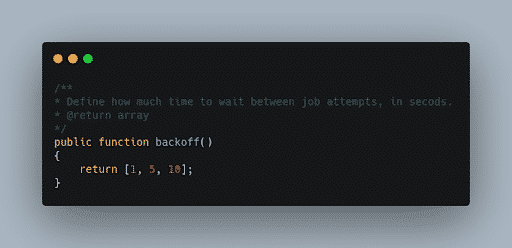
Now Laravel has this capability too, which is handy for jobs that deal with external APIs, where you wouldn’t want to try again in the same amount of time. It can also be used to dynamically generate the return array, setting different times to wait before retrying.
The above content is written by the help of moduscreate.
Now we will see what’s new in Laravel 7.
1. Laravel Sanctum
-> Laravel Sanctum provides a featherweight authentication system for SPAs (single page applications), mobile applications, and simple, token based APIs. Sanctum allows each user of your application to generate multiple API tokens for their account. These tokens may be granted abilities / scopes which specify which actions the tokens are allowed to perform.
For more information on Laravel Sanctum, consult the Sanctum documentation.
2. Custom Eloquent Casts
-> Laravel has a variety of built-in, helpful cast types; however, you may occasionally need to define your own cast types. You may now accomplish this by defining a class that implements the CastsAttributes
interface.
Classes that implement this interface must define a get
and set
methods. The get
method is responsible for transforming a raw value from the database into a cast value, while the set
method should transform a cast value into a raw value that can be stored in the database. As an example, we will re-implement the built-in json
cast type as a custom cast type:
<?php
namespace App\Casts;
use Illuminate\Contracts\Database\Eloquent\CastsAttributes;
class Json implements CastsAttributes
{
/**
* Cast the given value.
*
* @param \Illuminate\Database\Eloquent\Model $model
* @param string $key
* @param mixed $value
* @param array $attributes
* @return array
*/
public function get($model, $key, $value, $attributes)
{
return json_decode($value, true);
}
/**
* Prepare the given value for storage.
*
* @param \Illuminate\Database\Eloquent\Model $model
* @param string $key
* @param array $value
* @param array $attributes
* @return string
*/
public function set($model, $key, $value, $attributes)
{
return json_encode($value);
}
}
Once you have defined a custom cast type, you may attach it to a model attribute using its class name:
<?php
namespace App;
use App\Casts\Json;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
/**
* The attributes that should be cast to native types.
*
* @var array
*/
protected $casts = [
'options' => Json::class,
];
}
3. Blade Component Tags & Improvements
-> Blade components have been overhauled to allow tag-based rendering, attribute management, component classes, inline view components, and more. Since the overhaul of Blade components is so extensive, please consult the full Blade component documentation to learn about this feature.
4 . HTTP Client
-> Laravel now provides an expressive, minimal API around the Guzzle HTTP client, allowing you to quickly make outgoing HTTP requests to communicate with other web applications. Laravel’s wrapper around Guzzle is focused on its most common use cases and a wonderful developer experience. For example, the client makes it a breeze to POST
and interface with JSON data:
use Illuminate\Support\Facades\Http;
$response = Http::withHeaders([
'X-First' => 'foo',
'X-Second' => 'bar'
])->post('http://test.com/users', [
'name' => 'Taylor',
]);
return $response['id'];
In addition, the HTTP client provides fantastic, ergonomic testing functionality:
Http::fake([
// Stub a JSON response for GitHub endpoints...
'github.com/*' => Http::response(['foo' => 'bar'], 200, ['Headers']),
// Stub a string response for Google endpoints...
'google.com/*' => Http::response('Hello World', 200, ['Headers']),
// Stub a series of responses for Facebook endpoints...
'facebook.com/*' => Http::sequence()
->push('Hello World', 200)
->push(['foo' => 'bar'], 200)
->pushStatus(404),
]);
To learn more about all of the features of the HTTP client, please consult the HTTP client documentation.
5. Fluent String Operations
ou are likely familiar with Laravel’s existing Illuminate\Support\Str
class, which provides a variety of helpful string manipulation functions. Laravel 7 now offers a more object-oriented, fluent string manipulation library built on top of these functions. You may create a fluent Illuminate\Support\Stringable
object using the Str::of
method. A variety of methods may then be chained onto the object to manipulate the string:
return (string) Str::of(' Laravel Framework 6.x ')
->trim()
->replace('6.x', '7.x')
->slug();
For more information on the methods available via fluent string manipulation, please consult its full documentation.
6 . Route Caching Speed Improvements
-> Laravel 7 includes a new method of matching compiled, cached routes that have been cached using the route:cache Artisan command. On large applications (for example, applications with 800 or more routes), these improvements can result in a 2x speed improvement in requests per second on a simple “Hello World” benchmark. No changes to your application are required.
7. Route Model Binding Improvements
Route model binding improvements were contributed by Taylor Otwell.
Key Customization
Sometimes you may wish to resolve Eloquent models using a column other than id
. To do so, Laravel 7 allows you to specify the column in the route parameter definition:
Route::get('api/posts/{post:slug}', function (App\Post $post) {
return $post;
});
Automatic Scoping
Sometimes, when implicitly binding multiple Eloquent models in a single route definition, you may wish to scope the second Eloquent model such that it must be a child of the first Eloquent model. For example, consider this situation that retrieves a blog post by slug for a specific user:
use App\Post;
use App\User;
Route::get('api/users/{user}/posts/{post:slug}', function (User $user, Post $post) {
return $post;
});
When using a custom keyed implicit binding as a nested route parameter, Laravel 7 will automatically scope the query to retrieve the nested model by its parent using conventions to guess the relationship name on the parent. In this case, it will be assumed that the User
model has a relationship named posts
(the plural of the route parameter name) which can be used to retrieve the Post
model.
8. Multiple Mail Drivers
-> Laravel 7 allows the configuration of multiple “mailers” for a single application. Each mailer configured within the mail
configuration file may have its own options and even its own unique “transport”, allowing your application to use different email services to send certain email messages. For example, your application might use Postmark to send transactional mail while using Amazon SES to send bulk mail.
9. CORS Support
-> Laravel 7 includes first-party support for configuring Cross-Origin Resource Sharing (CORS) OPTIONS
request responses by integrating the popular Laravel CORS package written by Barry vd. Heuvel. A new cors
configuration is included in the default Laravel application skeleton.
10. Query Time Casts
-> Sometimes you may need to apply casts while executing a query, such as when selecting a raw value from a table. For example, consider the following query:
use App\Post;
use App\User;
$users = User::select([
'users.*',
'last_posted_at' => Post::selectRaw('MAX(created_at)')
->whereColumn('user_id', 'users.id')
])->get();
The last_posted_at
attribute on the results of this query will be a raw string. It would be convenient if we could apply a date
cast to this attribute when executing the query. To accomplish this, we may use the withCasts
method provided by Laravel 7:
$users = User::select([
'users.*',
'last_posted_at' => Post::selectRaw('MAX(created_at)')
->whereColumn('user_id', 'users.id')
])->withCasts([
'last_posted_at' => 'date'
])->get();
I hope you understand all the differences laravel 7 and laravel 8.
One Comment