.Net
Why multiple inheritance not allowed in C#?
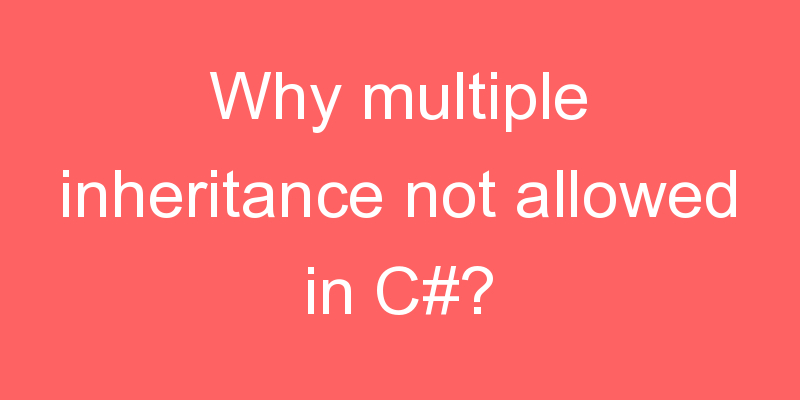
The one of problem for not supporting the multiple inheritance lies in the Diamond Problem.
The diamond problem is an ambiguity that arises when two classes B and C inherit from A, and class D inherits from both B and C. If a method in D calls a method defined in A (and does not override the method), and B and C have overridden that method differently, then from which class does it inherit: B, or C?
Let me give you a example.
public class A
{
public virtual void A_Method()
{
Console.WriteLine("Class A Method");
}
}
public class B:A
{
public override void A_Method()
{
Console.WriteLine("Class B Method");
}
}
public class C:A
{
public override void A_Method()
{
Console.WriteLine("Class C Method");
}
}
public class D:B,C // If Multiple inheritence is possible in C# then
{
public override void A_Method()
{
Console.WriteLine("Class C Method");
}
}
public static void main()
{
D objD = new D();
objD.A_Method();// Making object of class D and calling overloaded method A_method will confuse the compiler which class method to call as both inherited class methods has been overloaded.
}
C# doesn’t allow multiple inheritance with classes but it can be implemented using interface
. The reason behind is:
- Multiple inheritance add too much complexity with little benefit.
- There are huge chances of conflicting base class member. For example, if there is a methodÂ
calculate()
 in two base class and both are doing different calculation. What happened if user callÂcalculate()
 in child class? Which method will work? Inheritance
 withÂInterface
 provides same job of multiple inheritance.- Multiple Inheritance inject a lots of burden into implementation and it cause slow program execution.