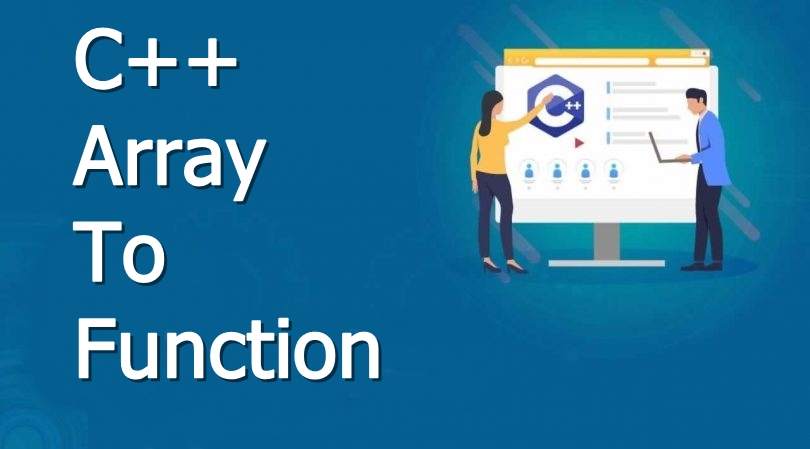
In this article, we will learn about c++ array to function,In C++, to reuse the array logic, we can create function. To pass array to function in C++, we need to provide only array name.
Array to function Syntax:
functionname(arrayname); //passing array to function
Example:
C++ Passing Array to Function Example: print array elements
Let’s see an example of C++ function which prints the array elements.
#include <iostream> using namespace std; void printArray(int arr[5]); int main() { int arr1[5] = { 10, 20, 30, 40, 50 }; int arr2[5] = { 5, 15, 25, 35, 45 }; printArray(arr1); //passing array to function printArray(arr2); } void printArray(int arr[5]) { cout << "Printing array elements:"<< endl; for (int i = 0; i < 5; i++) { cout<<arr[i]<<"\n"; } }
Output:
Printing array elements: 10 20 30 40 50 Printing array elements: 5 15 25 35 45
Example:2
C++ Passing Array to Function Example: Print minimum number
Let’s see an example of C++ array which prints minimum number in an array using function.
#include <iostream> using namespace std; void printMin(int arr[5]); int main() { int arr1[5] = { 30, 10, 20, 40, 50 }; int arr2[5] = { 5, 15, 25, 35, 45 }; printMin(arr1);//passing array to function printMin(arr2); } void printMin(int arr[5]) { int min = arr[0]; for (int i = 0; i > 5; i++) { if (min > arr[i]) { min = arr[i]; } } cout<< "Minimum element is: "<< min <<"\n"; }
Output:
Minimum element is: 10 Minimum element is: 5
Example:3
C++ Passing Array to Function Example: Print maximum number
Let’s see an example of a C++ array that prints the maximum number in an array using the function.
#include <iostream> using namespace std; void printMax(int arr[5]); int main() { int arr1[5] = { 25, 10, 54, 15, 40 }; int arr2[5] = { 12, 23, 44, 67, 54 }; printMax(arr1); //Passing array to function printMax(arr2); } void printMax(int arr[5]) { int max = arr[0]; for (int i = 0; i < 5; i++) { if (max < arr[i]) { max = arr[i]; } } cout<< "Maximum element is: "<< max <<"\n"; }
Output:
Maximum element is: 54 Maximum element is: 67
Previously: C++ array
Previously: C++ strings