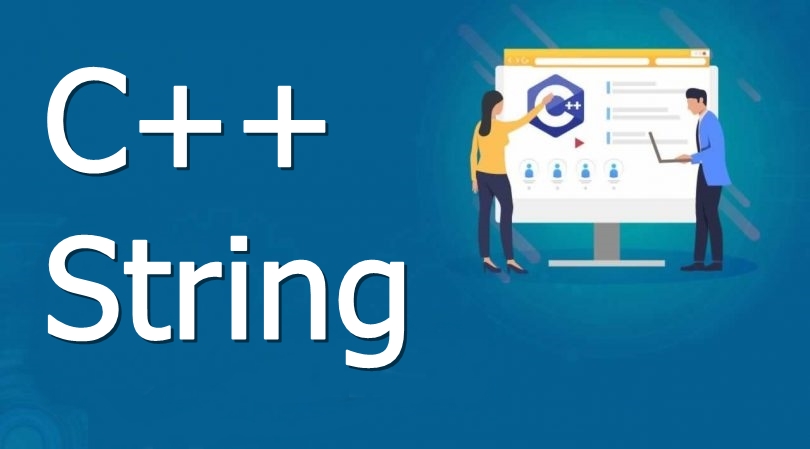
In this article, we will learn about c++ strings.
In C++, the string is an object of std::string class that represents a sequence of characters. We can perform many operations on strings such as concatenation, comparison, conversion, etc.
C++ String Example
Let’s see the simple example of C++ string.
#include <iostream> using namespace std; int main( ) { string s1 = "Hello"; char ch[] = { 'C', '+', '+'}; string s2 = string(ch); cout<<s1<<endl; cout<<s2<<endl; }
Output:
Hello C++
C++ String Compare Example
Let’s see the simple example of string comparison using strcmp() function.
#include <iostream> #include <cstring> using namespace std; int main () { char key[] = "mango"; char buffer[50]; do { cout<<"What is my favourite fruit? "; cin>>buffer; } while (strcmp (key,buffer) != 0); cout<<"Answer is correct!!"<<endl; return 0; }
Output:
What is my favourite fruit? apple What is my favourite fruit? banana What is my favourite fruit? mango Answer is correct!!
Previously: C++ array to function
Next: C++ pointers