How to collect the form data through PHP?
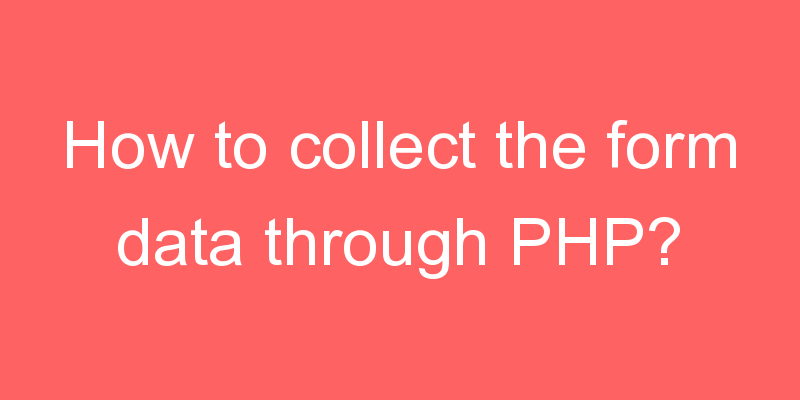
you’ll learn how to collect user inputs submitted through a form using the PHP Superglobal variables $_GET,$_POST,$_REQUEST
FIRSTLY CREATE A SIMPLE FORM
we are going to create a simple HTML contact form that allows users to enter their comment and feedback then displays it to the browser using PHP.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Contact Form</title>
</head>
<body>
<h2>Contact Us</h2>
<p>Please fill in this form and send us.</p>
<form action="action.php" method="post">
<p>
<label for="Name">Name:<sup>*</sup></label>
<input type="text" name="name">
</p>
<p>
<label for="Email">Email:<sup>*</sup></label>
<input type="text" name="email">
</p>
<p>
<label for="Subject">Subject:</label>
<input type="text" name="subject">
</p>
<p>
<label for="Comment">Message:<sup>*</sup></label>
<textarea name="message" rows="5" cols="30"></textarea>
</p>
<input type="submit" value="Submit">
<input type="reset" value="Reset">
</form>
</body>
</html>
Explanation of code
Notice that there are two attributes within the opening <form> tag:
- The action attribute references a PHP file “action.php” that receives the data entered into the form when user submit it by pressing the submit button.
- The method attribute tells the browser to send the form data through POST method.
Rest of the elements inside the form are basic form controls to receive user inputs
Fetch Form Data with PHP
To access the value of a particular form field, you can use the following Superglobal variables. These variables are available in all scopes throughout a script.
Superglobal | Description |
---|---|
$_GET | Contains a list of all the field names and values sent by a form using the get method (i.e. via the URL parameters). |
$_POST | Contains a list of all the field names and values sent by a form using the post method (data will not visible in the URL). |
$_REQUEST | Contains the values of both the $_GET and $_POST variables as well as the values of the $_COOKIE superglobal variable. |
When a user submit the above contact form through clicking the submit button, the form data is sent to the “action.php” file on the server for processing. It simply captures the information submitted by the user and displays it to browser.
Below is a PHP code in which you can fetch the form data. In this php file we used html by which php response become more attractive you can add css also it’s only up to you .
<!DOCTYPE html>
<html lang="en">
<head>
<title>Contact Form Data</title>
</head>
<body>
<h1>Thank You</h1>
<p>Here is the information you have submitted:</p>
<ol>
<li><em>Name:</em> <?php echo $_POST["name"]?></li>
<li><em>Email:</em> <?php echo $_POST["email"]?></li>
<li><em>Subject:</em> <?php echo $_POST["subject"]?></li>
<li><em>Message:</em> <?php echo $_POST["message"]?></li>
</ol>
</body>
</html>
So In this we can access the form data through php file using php Superglobal variable.