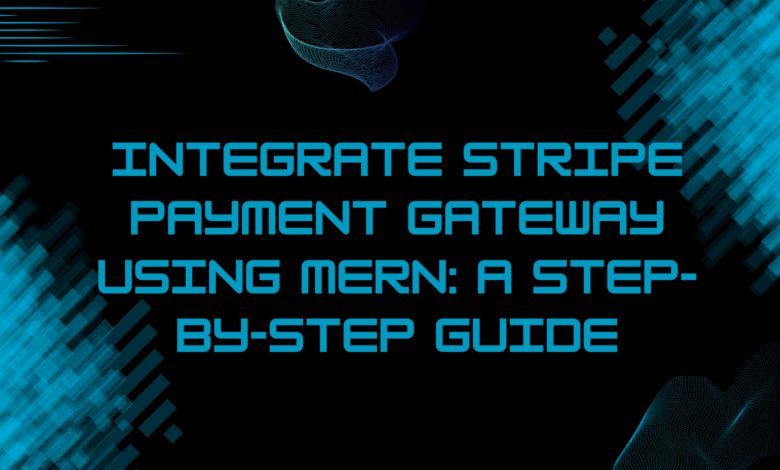
Introduction
If you’re building a web application with the MERN stack (MongoDB, Express.js, React, and Node.js) and need a seamless way to accept payments, Stripe is an excellent choice. It offers secure, fast, and developer-friendly payment solutions. In this guide (Integrate Stripe Payment Gateway Using MERN) we’ll walk you through the process of integrating Stripe into a MERN project, making online transactions smooth and efficient.
Why Choose Stripe for Payments?
Stripe is widely used by developers because of its ease of integration, robust security, and support for multiple payment methods. Whether you’re handling subscriptions, one-time payments, or marketplace transactions, Stripe provides the flexibility needed to scale your business.
Step 1: Setting Up Your Stripe Account
Before integrating Stripe, you need to create a Stripe account and get your API keys:
- Go to the Stripe website and sign up.
- Navigate to the Developers section and retrieve your Publishable Key and Secret Key from the API keys section.
- Keep these keys safe, as they will be used in your backend and frontend.
Step 2: Install Stripe in Your MERN Project
You need to install the Stripe package in both your backend (Node.js/Express) and frontend (React).
Backend Installation (Node.js & Express)
Run the following command in your server directory:
npm install stripe dotenv express cors body-parser
Frontend Installation (React)
Run this command in your client directory:
npm install @stripe/react-stripe-js @stripe/stripe-js
Step 3: Set Up Stripe in the Backend
Create a .env
file in your server directory and add your secret key:
STRIPE_SECRET_KEY=your_secret_key_here
Now, in your server.js
or a dedicated route file, set up a payment route:
require("dotenv").config();
const express = require("express");
const cors = require("cors");
const stripe = require("stripe")(process.env.STRIPE_SECRET_KEY);
const app = express();
app.use(express.json());
app.use(cors());
app.post("/api/create-payment-intent", async (req, res) => {
try {
const { amount, currency } = req.body;
const paymentIntent = await stripe.paymentIntents.create({
amount,
currency,
});
res.send({ clientSecret: paymentIntent.client_secret });
} catch (error) {
res.status(500).json({ error: error.message });
}
});
app.listen(5000, () => console.log("Server is running on port 5000"));
Step 4: Integrate Stripe in the Frontend
In your React application, create a PaymentForm.js
component to handle payments:
import React, { useState } from "react";
import { CardElement, useStripe, useElements } from "@stripe/react-stripe-js";
const PaymentForm = () => {
const stripe = useStripe();
const elements = useElements();
const [processing, setProcessing] = useState(false);
const [error, setError] = useState(null);
const handleSubmit = async (event) => {
event.preventDefault();
setProcessing(true);
const { error, paymentIntent } = await stripe.confirmCardPayment(
"your_client_secret",
{
payment_method: {
card: elements.getElement(CardElement),
},
}
);
if (error) {
setError(error.message);
setProcessing(false);
} else {
alert("Payment successful!");
setProcessing(false);
}
};
return (
<form onSubmit={handleSubmit}>
<CardElement />
<button type="submit" disabled={processing}>
{processing ? "Processing..." : "Pay Now"}
</button>
{error && <p>{error}</p>}
</form>
);
};
export default PaymentForm;
Step 5: Wrap the App with Stripe Provider
In App.js
, import and wrap your application with Elements
from Stripe:
import React from "react";
import { Elements } from "@stripe/react-stripe-js";
import { loadStripe } from "@stripe/stripe-js";
import PaymentForm from "./PaymentForm";
const stripePromise = loadStripe("your_publishable_key");
function App() {
return (
<Elements stripe={stripePromise}>
<PaymentForm />
</Elements>
);
}
export default App;
Testing the Integration
- Use Stripe’s test card 4242 4242 4242 4242 with any future expiration date and CVC.
- Check the Stripe dashboard to confirm the transaction.
- Deploy your backend to a cloud service like Heroku or Vercel and set up your frontend accordingly.
Integrating Stripe with the MERN stack is a straightforward process when broken down into clear steps. With a well-structured backend and a secure frontend, you can provide your users with a seamless payment experience. If you’re looking for expert guidance in web development and payment gateway integration, CodeHunger is a great choice for building secure and scalable applications.