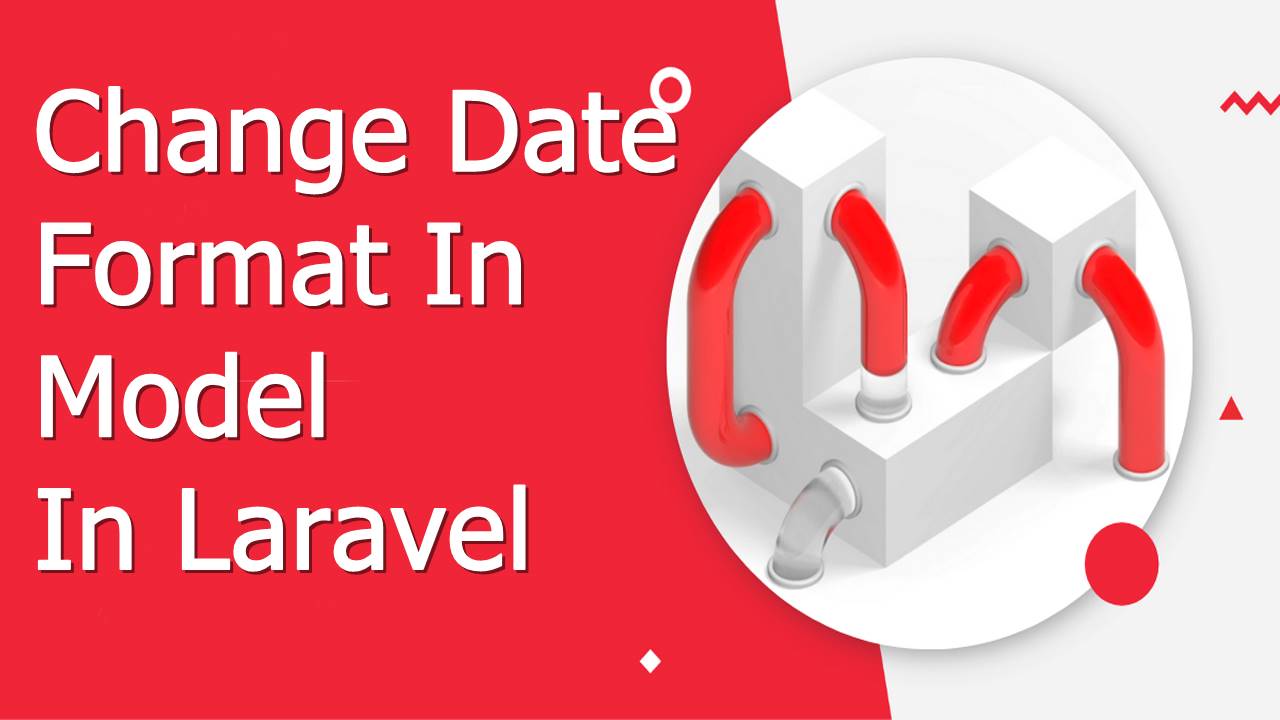
In this article, we will learn about how we can change date format via model in laravel, Well they are enormous ways are available to change date format in laravel. Let’s see each of them.
We gonna use the accessor and mutator concept of laravel to change the date format, if you want to learn more about the accessor and mutator you can learn from laravel official website.
Change Date format by defining an accessor
Accessor is beneficial when you are getting data from your table, doesn’t matter in what format you have saved the data.An accessor transforms an Eloquent attribute value when it is accessed. To define an accessor, create a get{Attribute}Attribute
method on your model where {Attribute}
is the “studly” cased name of the column you wish to access.
Look for the below example.
<?php
namespace App\Models;
Use Carbon\Carbon;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
/**
* Accessor for converting created_at value
* @param mixed $value
* return string
*/
public function getCreatedAtAttribute($value)
{
return Carbon::parse($value)->format('d-m-Y H:i:s');
}
}
By using the above code, when you get data in your model, you will get the data as per the format you have passed.
Change Date format by defining an mutator
Mutator is beneficial when you are saving data to the database at that time it will manipulate the data, with the format which you have added in your table model file.
A mutator transforms an Eloquent attribute value when it is set. To define a mutator, define a set{Attribute}Attribute
method on your model where {Attribute}
is the “studly” cased name of the column you wish to access.
<?php
namespace App\Models;
Use Carbon\Carbon;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
/**
* Accessor for converting created_at value
* @param mixed $value
* return string
*/
public function getCreatedAtAttribute($value)
{
return Carbon::parse($value)->format('d-m-Y H:i:s');
}
}
Change Date format using attribute casting
You can change the date format by using attribute casting also, in it will also work like an accessor when you get the data you will get it in the format which you have written in your table model file.
Attribute casting provides functionality similar to accessors and mutators without requiring you to define any additional methods on your model. Instead, your model’s $casts
property provides a convenient method of converting attributes to common data types.
See the below example for more clarification.
<?php
namespace App\Models;
Use Carbon\Carbon;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
protected $casts = [
'created_at' => 'date:Y-m-d',
];
}
Now there is a big question, what to do when you have to convert date-time format only for the particular query. So for that Query time casting is also available. Let’s see more about Query time casting
Change date format using Query Time Casting
Sometimes you may need to apply casts while executing a query, look for the below example and I bet you will definitely understand how query time casting will work.
$users = User::select([
'users.*',
])->withCasts([
'created_at' => 'date:Y-m-d'
])->get();
I hope now you understood what are the ways in which we can change the date format in laravel by using casting.
One Comment