Add custom attribute to laravel eloquent on load
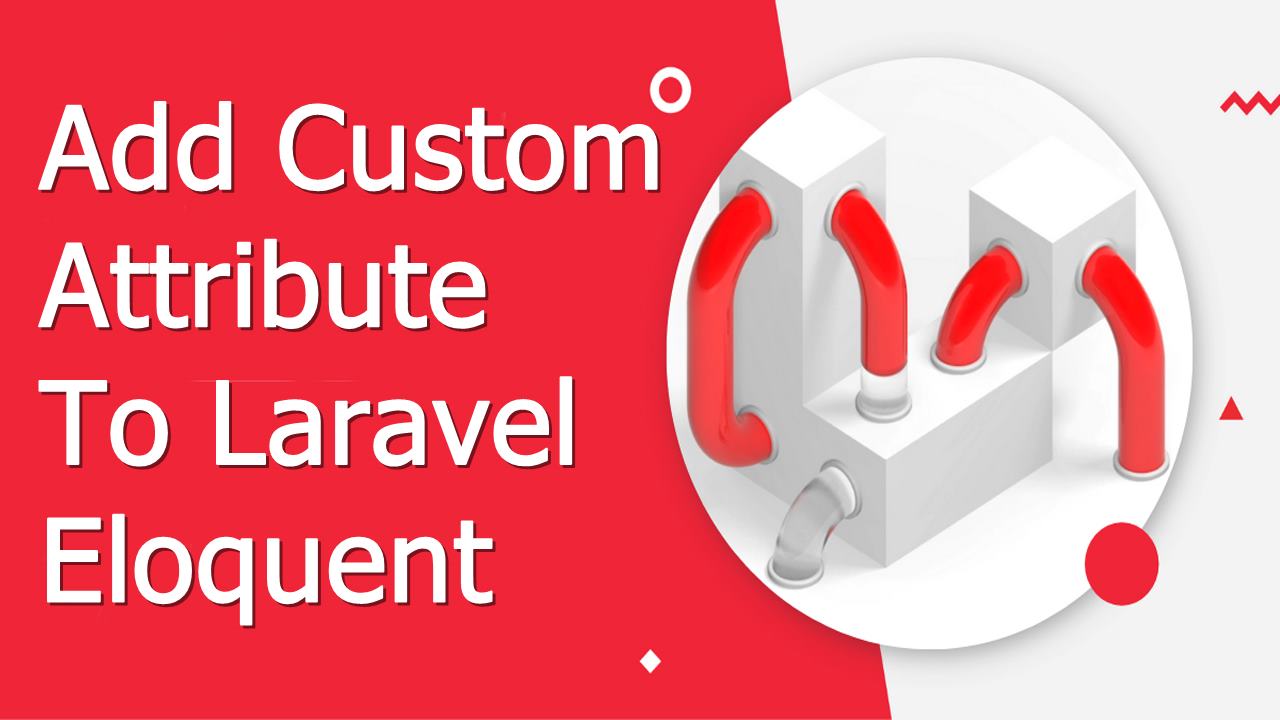
Hello there, I hope you are doing well, in this article we will learn about how to add custom attributes to laravel eloquent on load.
Or you can also say add an attribute to the model collection, we will use an accessor to achieve our goal, look for the below example.
Let’s suppose you need an IsAdmin attribute to check whether the user is admin or not, how we can do this we will learn in the below example.
Occasionally, when converting models to arrays or JSON, you may wish to add attributes that do not have a corresponding column in your database. To do so, first define an accessor for the value:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
/**
* Determine if the user is an administrator.
*
* @return bool
*/
public function getIsAdminAttribute()
{
return $this->attributes['admin'] === 'yes';
}
}
Now the part is we have to append it into our array. so we have to add an attribute name to the appends property of your model. so the final code will be something like below.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
/**
* The accessors to append to the model's array form.
*
* @var array
*/
protected $appends = ['is_admin'];
/**
* Determine if the user is an administrator.
*
* @return bool
*/
public function getIsAdminAttribute()
{
return $this->attributes['admin'] === 'yes';
}
}
If you want to learn more about how to add attributes to an object or custom attributes to eloquent then you can visit the laravel official website from here.
I hope now you understood that how we can inject custom attributes to eloquent during load time.