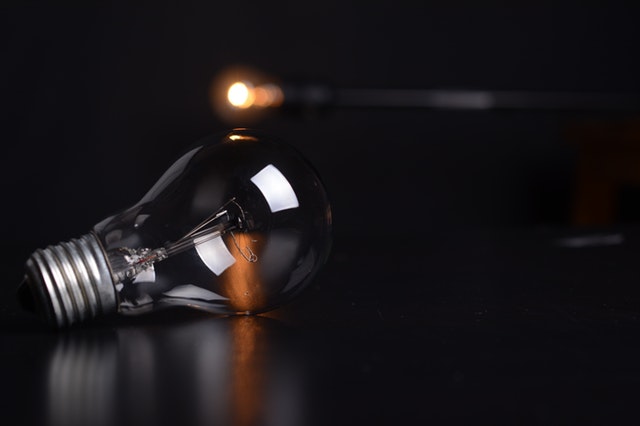
Malloc Function
It is used to allocate space in memory during runtime and it stands for memory allocation.
Below is the example of malloc function
#include<stdio.h>
#include<stdlib.h>// it includes calloc and malloc function.
void main()
{
int n,*ptr,*p,sum=0,i;
printf("enter the size of array");
scanf("%d",&n);
ptr=(int*)malloc(*sizeof(int));
p=ptr;
printf("enter the element in array " );
for(i=1;i<n;i++) {
scanf(" %d",ptr );
sum=sum+*ptr;
ptr++;
}
printf(" array element ")
for(i=1;i<=n;i++) {
printf("%d",*p);
p++;
}
printf("addtion is %d " ,sum);
}
// Output:
// enter the size of array 4
// enter the element in array 1 2 3 4
// array element 1234 addition is10
Calloc function
The calloc function allocates a block of memory for an array, it stands for contiguous allocation.
Below is the example of calloc function
#include<stdio.h>
#include<stdlib.h>
void main()
{
int n,*ptr,*p;
printf(" no of elements to be entered ":);
scanf(" %d" ,&n);
ptr=(int*)calloc(n,sizeof(int));
p=ptr;
if(ptr=NULL)
{
printf(" out of memory " );
exit(0);
}
printf("enter the element ",n);
for(i=1;i<=n;i++)
{
scanf(" %d",ptr ) ;
sum=sum+*ptr;
ptr++
}
printf("array element \n " );
for(i=1;i<=n;i++)
{
printf(" %d" ,*p);
p++;
}
printf("addition is %d " ,sum);
}
// output:
// enter size of array 3
// enter the element 123
// array element 123 addition 6
Still having confusion regarding the written blog feel free to comment.