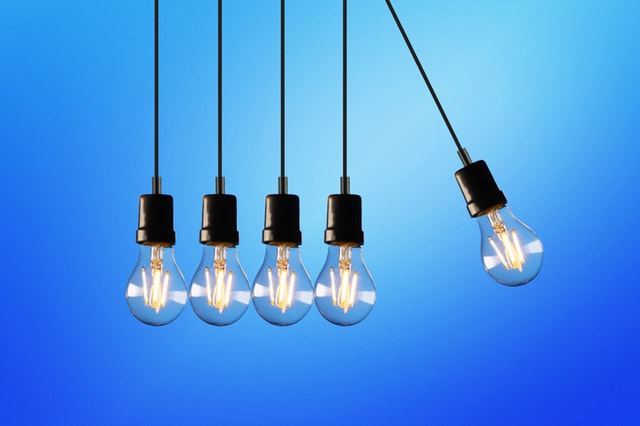
A Pointer is a variable that stores the address of another variable. A Pointer is declared using specific data type and therefore that pointer can point a variable of the same data type.
Syntax
Data_type *pt_name;
Eg:- int *ptr;
This tells the compiler few things about the variable ‘ptr’
- The asterisk(*) tells that the variable ‘ptr’ is a pointer variable.
- ‘ptr’ needs a memory location.
- ‘ptr’ points a variable of an int data type.
Advantage of pointer
- A pointer provides direct access to memory bitwise.
- Pointers are more efficient in handling the data tables.
- Pointers are also used for writing the operating system.
- They have used a graphics-intensive application that requires direct and frequent access to memory.
- Pointers reduce the length and complexity of a program.
- They increase the execution speed.
- The use of a pointer array to character strings results in saving of data storage space in memory.
Pointer to Pointer
A pointer to a pointer is a form of multiple indirections or a chain of pointers. Normally, a pointer contains the address of a variable. When we define a pointer to a pointer, the first pointer contains the address of the second pointer.
A variable that is a pointer to a pointer must be declared as such. This is done by placing an additional asterisk(*) in front of its name. For example, the following declaration declares a pointer to a pointer of type int- int **var;
Pointer to Pointer Example
#include<stdio.h>
void main()
{
int number=50;
int *p;//pointer to int
int **p2;//pointer to pointer
p=&number;
p2=&p;
printf("Address of number
variable is %x \n",&number);
printf("Address of p variable is %x \n",p);
printf("Value of *p variable is %d \n",*p);
printf("Address of p2 variable is %x \n",p2);
printf("Value of **p2 variable is %d \n",**p);
}
Still confused regarding the posted blog , feel free to comment we are here to help you.