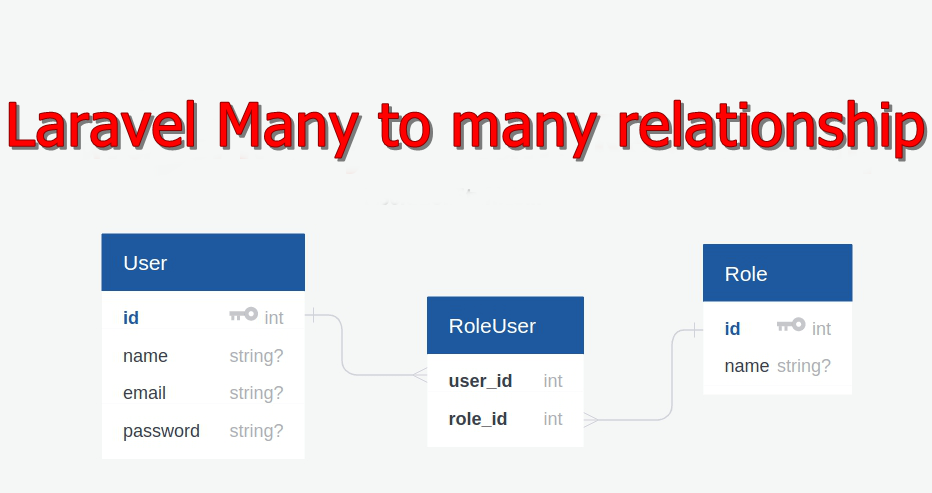
In this article we will learn about many to many relationships in Laravel 8, many to many relationships are more complicated than one to one and one many relationships.
In this example, i will create “users”, “roles” and “role_user” tables. each table is connected with each other. now we will create many to many relationships with each other by using the laravel Eloquent Model. We will first create database migration, then model, retrieve records and then how to create records too. So you can also see database table structure on below screen.
To create many to many relationship in Laravel 8 we are going to follow the below steps. If you want to know more about the many to many relationship in Laravel you can also visit Laravel’s official site from here.
- Install Laravel
- Create Migration and Model
- Write Migration Code
- Run the Migration
- Write Model Code
- Retrieve the record
- Create the record
Step-1: Download the Laravel Application
Open your command prompt under your htdocs and run the below command
composer create-project laravel/laravel laravel-relationship
Step-2: Create Migration for many to many relationships
To create migration in order to learn many to many relationship in laravel 8 we will run the below command. first, we going to create a user model and migration. The below command will create a model and migration file for users.
Note : Leave the below command if User model is already present under your app/Http/Models
php artisan make:model User -m
Now we will create a role model run the below command to create role model and migration together
php artisan make:model Role -m
Now we will create a user role model run the below command to create RoleUser model and migration together.
php artisan make:model RoleUser -m
Step -3 : Write the migration code
Go go to app/http/database and look for user_table_migration and write the below code
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
In that folder now look for roles_table_migration and add the below code
Schema::create('roles', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->timestamps();
});
now look for roles_user_table_migration and add the below code
Schema::create('role_user', function (Blueprint $table) {
$table->integer('user_id')->unsigned();
$table->integer('role_id')->unsigned();
$table->foreign('user_id')->references('id')->on('users')
->onDelete('cascade');
$table->foreign('role_id')->references('id')->on('roles')
->onDelete('cascade');
});
Step : 4 Run the migration command
Now we will run migration to create all those tables in our database, please type the below command
php artisan migrate
Step -5 : Write the model code
Here, we will create User, Role and RoleUser table model. we will also use “belongsToMany()” for relationship of both model.
Now go to app/http/models here you can see User.php open that file and add the below code.
<?php
namespace App;
use Illuminate\Notifications\Notifiable;
use Illuminate\Foundation\Auth\User as Authenticatable;
class User extends Authenticatable
{
use Notifiable;
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name', 'email', 'password',
];
/**
* The attributes that should be hidden for arrays.
*
* @var array
*/
protected $hidden = [
'password', 'remember_token',
];
/**
* The roles that belong to the user.
*/
public function roles()
{
return $this->belongsToMany(Role::class, 'role_user');
}
}
Now go to app/http/models here you can see Role.php open that file and add the below code.
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Role extends Model
{
/**
* The users that belong to the role.
*/
public function users()
{
return $this->belongsToMany(User::class, 'role_user');
}
}
Step: 6 Retrive the records
Now write the below code to retrive the records.
$user = User::find(1); dd($user->roles);
$role = Role::find(1); dd($role->users);
Step :7 Create the records
Now write the below code to create the records.
$user = User::find(2); $roleIds = [1, 2];$user->roles()->attach($roleIds);
$user = User::find(3); $roleIds = [1, 2];$user->roles()->sync($roleIds);
$role = Role::find(1); $userIds = [10, 11];$role->users()->attach($userIds);
$role = Role::find(2); $userIds = [10, 11];$role->users()->sync($userIds);
Read Also: One to one relationship in Laravel 8
Read Also: One to many relationship in Laravel 8
I hope you understood the many to many relationship in Laravel, if you feel any confusion feel free to comment.