How to set x-frame options header in Laravel 8
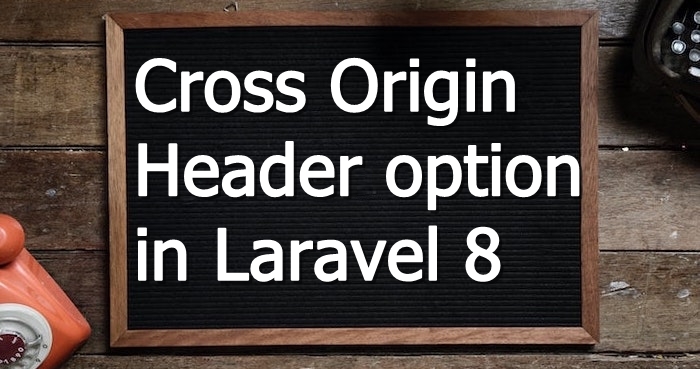
In this article I will guide you how we can set x-frame options header in Larvel 8, basically this error when trying to open something in our iframe,then in the console it shows error x-frame option ‘X-Frame-Options’ to ‘sameorigin’.
You can set the header in your Laravel application by creating a middleware, To set the header we will follow the below steps.
- Create a middleware
- Write the middlware code
- Register it in Kernel.php
Step : 1 Create a middleware
Run the below command to create middleware in Laravel.
php artisan make:middleware FrameHeadersMiddleware
The above command will create middleware inside app/Http/Middleware named as FrameHeadersMiddleware.php
Step – 2: Write the middleware code
Now open FrameHeadersMiddleware.php and write the below code.
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class FrameHeadersMiddleware
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle(Request $request, Closure $next)
{
$response = $next($request);
$response->header('X-Frame-Options', 'ALLOW FROM YOUR-URL');
return $response;
}
}
Step : 3 Add it in Kernel.php
Now go to app/Http/Kernel.php and it under your protected middleware array.
/**
* The application's global HTTP middleware stack.
*
* These middleware are run during every request to your application.
*
* @var array
*/
protected $middleware = [
// \App\Http\Middleware\TrustHosts::class,
\App\Http\Middleware\TrustProxies::class,
\Fruitcake\Cors\HandleCors::class,
\App\Http\Middleware\PreventRequestsDuringMaintenance::class,
\Illuminate\Foundation\Http\Middleware\ValidatePostSize::class,
\App\Http\Middleware\TrimStrings::class,
\Illuminate\Foundation\Http\Middleware\ConvertEmptyStringsToNull::class,
\App\Http\Middleware\HeaderHandler::class,
\App\Http\Middleware\FrameHeadersMiddleware::class, // frameHeaderMiddleware
];
Read Also: How to use usort in Laravel
I hope you like this blog and if your issue still not resolved feel free to comment.