React Native – Geolocation

In this post, we will learn, how to implement Geolocation in a React Native application.
What Is Geolocation?
The most famous and familiar location feature — Geolocation is the ability to track a device using GPS, cell phone towers, WiFi access points, etc. Geolocation uses positioning systems to trace an individual’s whereabouts right down to latitude and longitude, or more practically, a physical address. Both mobile and desktop devices can use Geolocation.
It extends the Geolocation web specification. This API is available through the navigator.geolocation globally, so that we do not need to import it.
In Android, Geolocation API uses android.location API. However, this API is not recommended by Google because it is less accurate, and slower than the recommended Google Location Services API. To use the Google Location Services API in React Native, use the react-native-geolocation-service module.
iOS
For iOS platform we include the NSLocationWhenInUseUsageDescription key in Info.plist to enable geolocation. Geolocation is by default enabled when a project is created with react-native init.
To enable the geolocation in background, we need to include the ‘NSLocationAlwaysUsageDescription’ key in Info.plist. This requires adding location as a background mode in the ‘Capabilities’ tab in Xcode.
Android
To access the location in Android, we need to add <uses-permission android:name=”android.permission.ACCESS_FINE_LOCATION” /> in AndroidManifest.xml file.
The Android API >=23 requires to request the ACCESS_FINE_LOCATION permission using the PermissionsAndroid API.
Method | Description |
---|---|
getCurrentPosition() | It invokes the success callback once with latest location information. |
watchPosition() | It invokes the success callback whenever the location changes. It returns a watchId (number). |
clearWatch() | It clears the watchId of watchPosition(). |
stopObserving() | It stops observing for device location changes as well as it removes all listeners previously registered. |
setRNConfiguration() | It sets the configuration options, which is used in all location requests. |
requestAuthorization() | It requests suitable Location permission based on the key configured on pList. |
Run the following command to install
npm install @react-native-community/geolocation --save
This command will copy all the dependency into your node_module directory.
CocoaPods Installation
After the updation of React Native 0.60, they have introduced autolinking so we do not require to link the library but need to install pods. So to install pods use
cd ios && pod install && cd ..
Permission to use the Geolocation for Android
We are using a Native Location API so we need to add some permission to the AndroidManifest.xml
file. We are going to add the following permissions in the AndroidMnifest.xml
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
Permission | Purpose |
---|---|
ACCESS_FINE_LOCATION | To access the Location Via GPS |
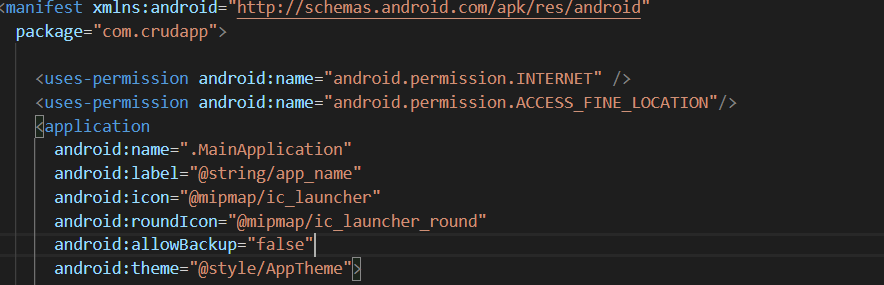
App.js
import React from 'react';
import {View, Text, StyleSheet, Image ,PermissionsAndroid,Platform} from 'react-native';
import Geolocation from '@react-native-community/geolocation';
export default class App extends React.Component {
state = {
currentLongitude: 'unknown',//Initial Longitude
currentLatitude: 'unknown',//Initial Latitude
}
componentDidMount = () => {
var that =this;
if(Platform.OS === 'ios'){
this.callLocation(that);
}else{
async function requestLocationPermission() {
try {
const granted = await PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.ACCESS_FINE_LOCATION,{
'title': 'Location Access Required',
'message': 'This App needs to Access your location'
}
)
if (granted === PermissionsAndroid.RESULTS.GRANTED) {
that.callLocation(that);
} else {
alert("Permission Denied");
}
} catch (err) {
alert("err",err);
console.warn(err)
}
}
requestLocationPermission();
}
}
callLocation(that){
Geolocation.getCurrentPosition(
(position) => {
const currentLongitude = JSON.stringify(position.coords.longitude);
const currentLatitude = JSON.stringify(position.coords.latitude);
that.setState({ currentLongitude:currentLongitude });
that.setState({ currentLatitude:currentLatitude });
},
(error) => alert(error.message),
{ enableHighAccuracy: true, timeout: 20000, maximumAge: 1000 }
);
that.watchID = Geolocation.watchPosition((position) => {
console.log(position);
const currentLongitude = JSON.stringify(position.coords.longitude);
const currentLatitude = JSON.stringify(position.coords.latitude);
that.setState({ currentLongitude:currentLongitude });
that.setState({ currentLatitude:currentLatitude });
});
}
componentWillUnmount = () => {
Geolocation.clearWatch(this.watchID);
}
render() {
return (
<View style = {styles.container}>
<Image
source={{uri:'https://png.icons8.com/dusk/100/000000/compass.png'}}
style={{width: 100, height: 100}}
/>
<Text style = {styles.boldText}>
You are Here
</Text>
<Text style={{justifyContent:'center',alignItems: 'center',marginTop:16}}>
Longitude: {this.state.currentLongitude}
</Text>
<Text style={{justifyContent:'center',alignItems: 'center',marginTop:16}}>
Latitude: {this.state.currentLatitude}
</Text>
</View>
)
}
}
const styles = StyleSheet.create ({
container: {
flex: 1,
alignItems: 'center',
justifyContent:'center',
marginTop: 50,
padding:16,
backgroundColor:'white'
},
boldText: {
fontSize: 30,
color: 'red',
}
})