React Native
React Native – Get Current Date Time
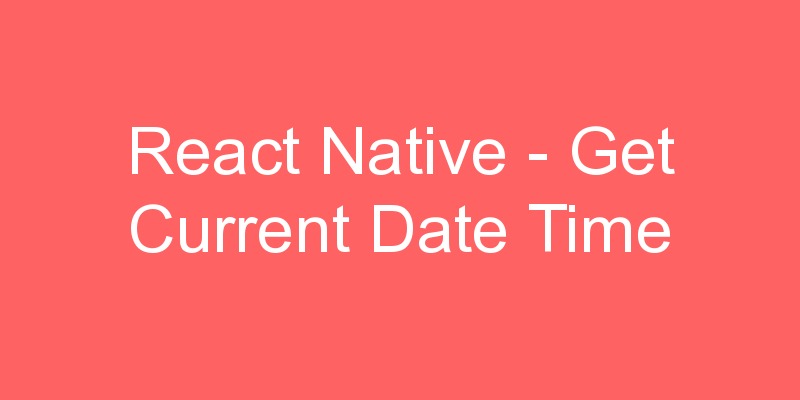
The JavaScript’s inbuilt function Date().getDate() gives us the facility to retrieve Get Current Date With Month And Year in both android and iOS applications. In this tutorial, we will see how to get the current date-time in React Native using the Date function directly and using the moment.js
which is very helpful when you deal with the date and time. Moment.js is used to parse, validate, manipulate, and display dates and times in JavaScript.
For getting date,time ,current year etc etc we use these functions
var date = new Date().getDate(); //To get the Current Date
var month = new Date().getMonth() + 1; //To get the Current Month
var year = new Date().getFullYear(); //To get the Current Year
var hours = new Date().getHours(); //To get the Current Hours
How to Get Current Time using moment.js
var date = moment()
.utcOffset('+05:30')
.format('YYYY-MM-DD hh:mm:ss a');
moment()
will return the current date-timeutcOffset
is used to set your time zoneformat
will decide the output format- For Time Format:
- Y/YYYY -> Year
- M -> Month in single-digit(Ex. 8)
- MM -> Month in double-digit(Ex. 08)
- MMM -> Month short name (Ex. Aug)
- MMMM -> Month full name (Ex. August)
- D -> Day in single-digit(Ex. 5)
- DD -> Day in single double-digit(Ex. 05)
- HH -> Hours in 24 hr format
- hh -> Hours in 12 hr format
- mm -> Minutes
- ss -> Seconds
- a -> am/pm
You can use either Date function or moment.js to get the Date Time but use Date function while you are dealing in 24hr format and if you are using 12hr (am/pm) format then go for moment.js.
App.js
import React, { Component } from 'react';
import { StyleSheet, View, Alert, Text } from 'react-native';
export default class App extends Component {
constructor(props) {
super(props);
this.state = {
date: '',
};
}
componentDidMount() {
var that = this;
var date = new Date().getDate(); //Current Date
var month = new Date().getMonth() + 1; //Current Month
var year = new Date().getFullYear(); //Current Year
var hours = new Date().getHours(); //Current Hours
var min = new Date().getMinutes(); //Current Minutes
var sec = new Date().getSeconds(); //Current Seconds
that.setState({
date:
date + '/' + month + '/' + year + ' ' + hours + ':' + min + ':' + sec,
});
}
render() {
return (
<View
style={{
flex: 1,
justifyContent: 'center',
alignItems: 'center',
}}>
<Text
style={{
fontSize: 20,
}}>
Current Date Time
</Text>
<Text
style={{
fontSize: 20,
marginTop: 16,
}}>
{this.state.date}
</Text>
</View>
);
}
}
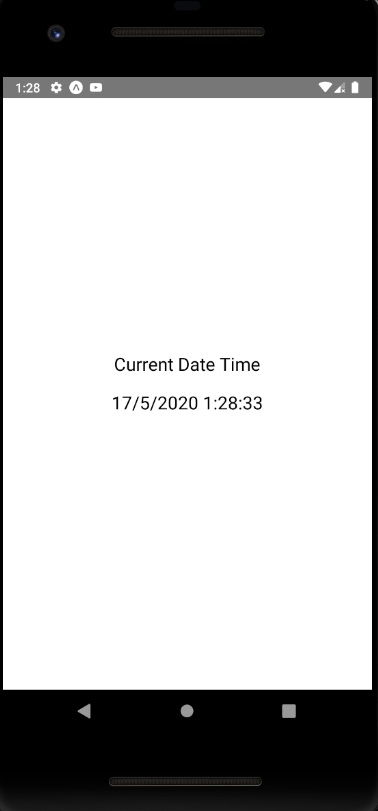