React Native – HTTP API Call

This post will give you an idea about how to Make HTTP Request to Fetch the Data From Web APIs in React Native. Here is an interesting example of posting and fetching the data from the server. Whenever you connect your application from the backend server (to get or post the data) you have to make an HTTP request. Here we will show you how to perform network tasks in React Native.
Code Snippet of Basic Network Call using Fetch
fetch('URL HERE', {
method: 'GET'
//Request Type
})
.then((response) => response.json())
//If response is in json then in success
.then((response) => {
//Success
console.log(response);
})
//If response is not in json then in error
.catch((error) => {
//Error
console.error(error);
});
In the example below, we are using free demo APIs. The example has two-button each has its own onPress which triggers our services. Just for the demo purpose, we are using buttons to trigger the request but If You need any data which needs to render while loading your screen you can use componentDidMount lifecycle method to load the data from the server as soon as the component is mounted.
App.js Code
import React, { Component } from 'react';
import { StyleSheet, View, Button, Alert} from 'react-native';
export default class App extends Component {
getDataUsingGet(){
//GET request
fetch('https://jsonplaceholder.typicode.com/posts/1', {
method: 'GET'
})
.then((response) => response.json())
.then((responseJson) => {
alert(JSON.stringify(responseJson));
console.log(responseJson);
})
.catch((error) => {
alert(JSON.stringify(error));
console.error(error);
});
}
getDataUsingPost(){
var dataToSend = {title: 'Hii', body: 'From CodeHunger', userId: 1};
var formBody = [];
for (var key in dataToSend) {
var encodedKey = encodeURIComponent(key);
var encodedValue = encodeURIComponent(dataToSend[key]);
formBody.push(encodedKey + "=" + encodedValue);
}
formBody = formBody.join("&");
fetch('https://jsonplaceholder.typicode.com/posts', {
method: "POST",//Request Type
body: formBody,//post body
headers: {//Header Defination
'Content-Type': 'application/x-www-form-urlencoded;charset=UTF-8'
},
})
.then((response) => response.json())
.then((responseJson) => {
alert(JSON.stringify(responseJson));
console.log(responseJson);
})
.catch((error) => {
alert(JSON.stringify(error));
console.error(error);
});
}
render() {
return (
<View style={styles.MainContainer}>
<Button title='Get Data Using GET' onPress={this.getDataUsingGet}/>
<Button title='Get Data Using POST' onPress={this.getDataUsingPost}/>
</View>
);
}
}
const styles = StyleSheet.create({
MainContainer :{
justifyContent: 'center',
flex:1,
margin: 10
}
});
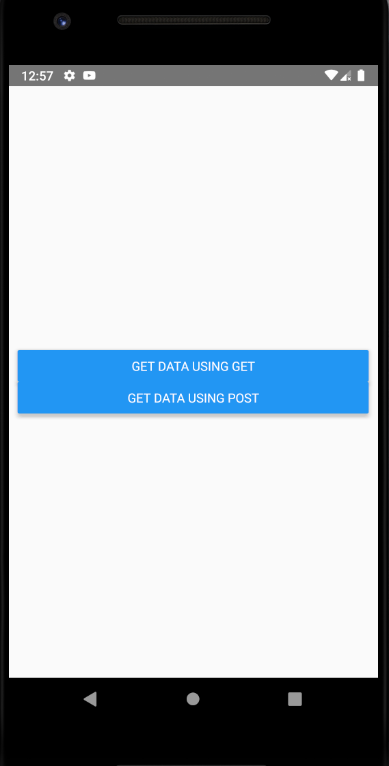
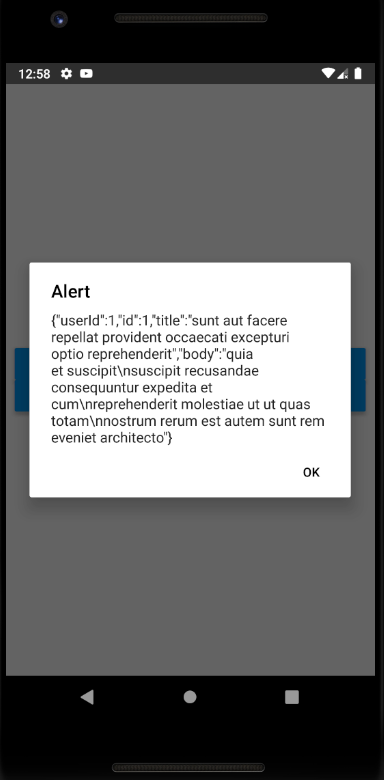
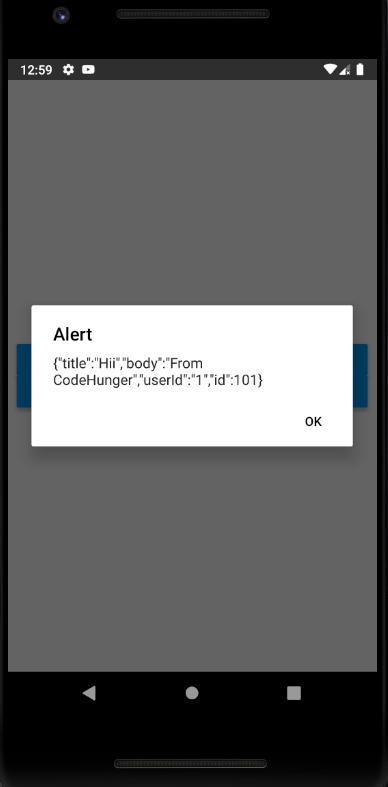
GET requests
Sending a GET request to a JSON API is the simplest use case. Just call fetch and supply it with the appropriate URL. It returns a promise that can be parsed as usual:
fetch('/users.json')
.then(function(response) {
return response.json()
})
POST requests
When submitting a POST request, supply the URL as the first argument and an object containing the request information as the second argument.
There are two things to note:
- Make sure you send the correct headers. Otherwise, the payload won’t get through.
- Stringify the JSON payload before sending it.
As an example, follow the documetation on the React Native website:
fetch('https://mywebsite.com/endpoint/', {
method: 'POST',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json',
},
body: JSON.stringify({
firstParam: 'yourValue',
secondParam: 'yourOtherValue',
})
})
Or add in some ES6!
Because React Native ships with the Babel Javascript Compiler as of version 0.5.0, we are free to use ES6 enhanced object literals and template strings!
let email = "joe@example.com";
let password = "donkeybrains";
let myApiUrl = "http://www.example.com/api"
let usersPath = "users"fetch(`${myApiUrl}/${usersPath}`, {
.
.
body: JSON.stringify({
user: {email, password}
})
})