string and StringBuilder
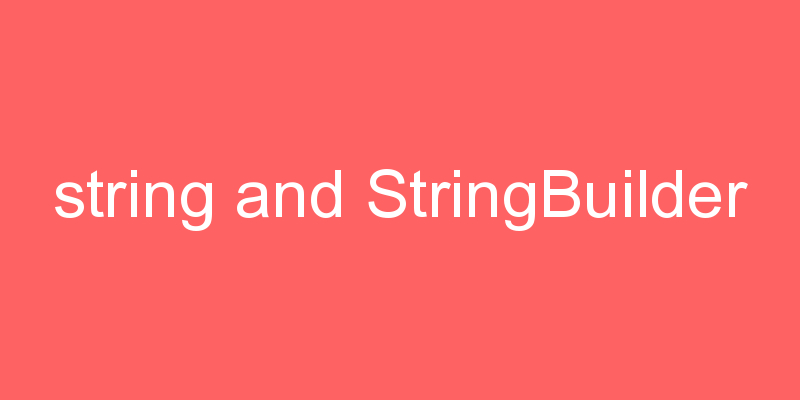
A string instance is immutable. Immutable means once we create a string object we cannot modify the value of the string Object in the memory. Any operation that appears to modify the string, it will discard the old value and it will create new instance in memory to hold the new value.
The System.Text.StringBuilder is mutable, that means once we create StringBuilder object we can perform any operation that appears to change the value without creating new instance for every time. It can be modified in any way and it doesn’t require creation of new instance.
String Example
string Name;
Name += "Piyush";
Name += "Kumar";
Name += "Gujral";
In the above code string color will alter 3 times, each time the code perfom a string operation (+=). That mean 3 new string created in the memory. When you perform repeated operation to a string, the overhead associated with creating a new String object can be costly.
StringBuilder Example
StringBuilder sbb = new StringBuilder("");
sbb.Append("Piyush");
sbb.Append("Kumar");
sbb.Append("Gujral");
string Name= sbb.ToString();
In the above code the StringBuilder object will alter 3 times, each time the code attempt a StringBuilder operation without creating a new object. That means, using the StringBuilder class can boost performance when concatenating many strings together in a loop.
But immutable objects have some advantages also, such as they can be used across threads without fearing synchronization problems. On the other hand, when initializing a StringBuilder, you are going down in performance. Also many actions that you do with string can’t be done with StringBuilder object.