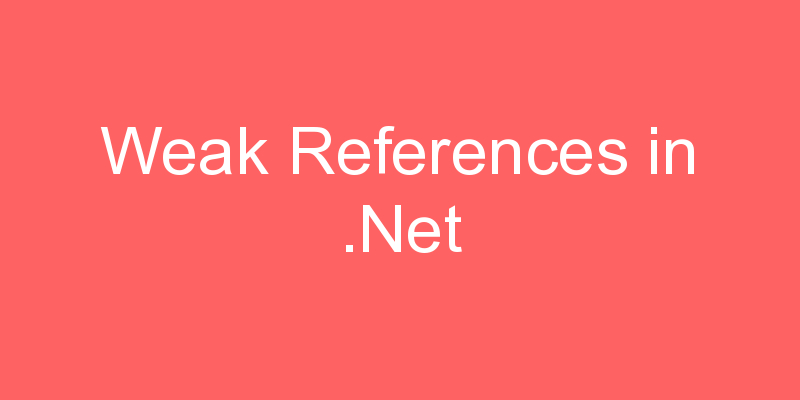
Weak references in .Net create references to large objects in your application that are used infrequently so that they can be reclaimed by the garbage collector if needed. Let me explain you what basically Weak Reference is.
A weak reference is a way to have some pointer to an object that does have any reference (strong reference). In .NET, any normal reference to another object is a strong reference . That is, when you declare a variable of a type that is not a primitive/value type, you are declaring a strong reference.
The Garbage Collector (GC) start cleaning memory for objects that do not have any reference. The GC (garbage collector) cannot collect an object in use by an application while the application’s code can reach that object. If you hold a strong reference to an object directly in a static variable or in a local variable, it can’t be collected. Also, if such an object holds references to other objects, those other objects can’t be collected either.
A weak reference permits the garbage collector to collect the object while still allowing the application to access the object. When we need to access a weak referenced object we can just check if the object is alive and then access it if the object is alive at all. Most objects that are referenced must be kept in memory until they are unreachable. But with WeakReference , objects that are referenced can be collected. A weak reference is valid only during the indeterminate amount of time until the object is collected when no strong references exist.
Programming weak reference in C#
To create a weak reference you would need to take advantage of the System.WeakReference class. Once you have created a weak reference to an object, you can use the Target property of the weak reference that you have created to check if the original object is still alive. The following code snippet shows how you can create a weak reference to an object.
Rectangle rectangle = new Rectangle(15, 10);
var weakReference = new WeakReference(rectangle);
You can use the IsAlive property to check if the weak reference to the object is still alive. Here’s a code listing that illustrates this.
static void Main(string[] args)
{
Rectangle rectangle = new Rectangle(15, 10);
var weakReference = new WeakReference(rectangle);
rectangle = null;
bool isAlive = weakReference.IsAlive;
if(isAlive)
Console.WriteLine("The object is still alive");
Console.Read();
}
If the strong reference to the object is no longer available, you can leverage the Target property of the weak reference to use the object as shown in the code snippet given below.
bool isAlive = weakReference.IsAlive;
if(isAlive)
{
Rectangle rectangle = weakReference.Target as Rectangle;
//You can now use the rectangle object as usual
}