
In this section I am going to show you how we can do the CRUD Operation in Asp.Net Web API.
What is Web API in ASP.NET
ASP.NET Web API is a framework for building HTTP based services that can be consumed from any client including browsers and mobile devices. ASP.NET Web API is an extension of WCF REST API.
API = Application Programming Interface.
ASP.NET Web API Characteristics
- It is based on RESTful architecture.
- Uses standard HTTP methods (GET, PUT, POST, DELETE).
- It supports multiple text formats like XML, JSON, etc.
- Completely stateless.
- It supports ASP.NET MVC features.
- Supports Self Hosting and IIS Hosting.
Step by step:
- First we have to choose a Visual Studio Blank Solution.
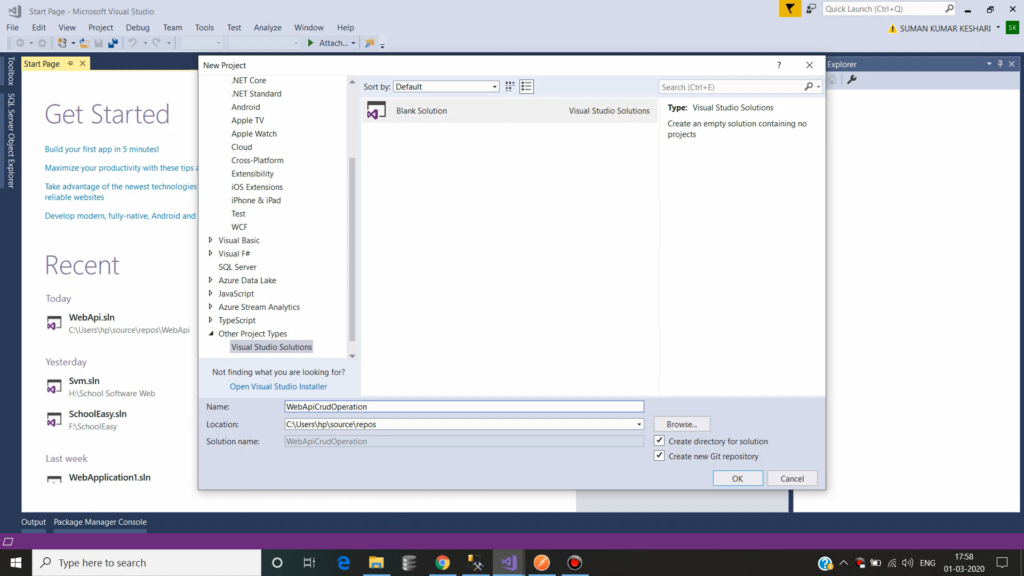
2. After that we have to add a C# Class Library for Data Access Layer where we can handle our database related things or you can say a business layer.

3. After that we have to add a Asp.Net Web Application where we write our code for CRUD Operation.
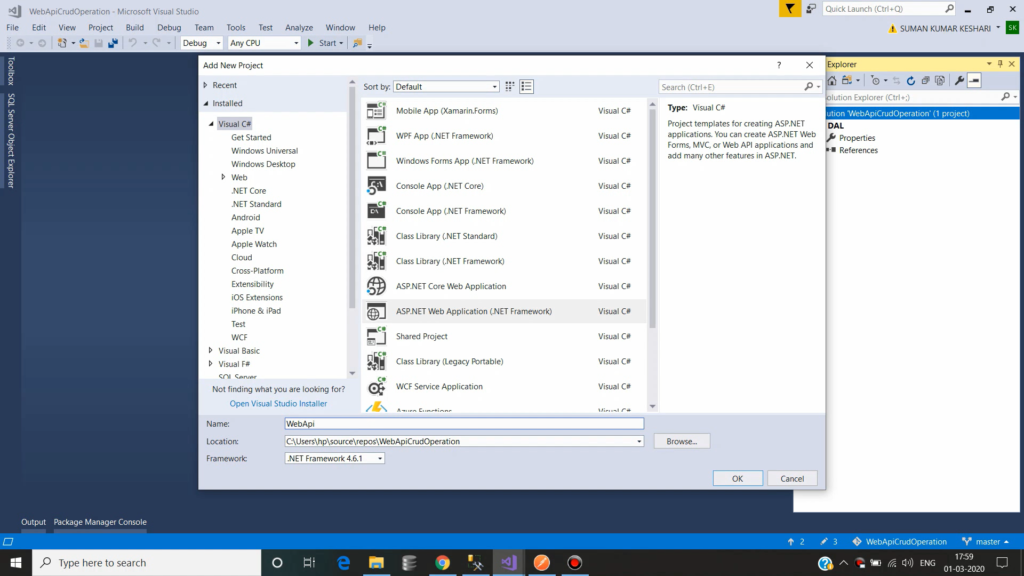
4. After that we have to select the layers like Web API for our project.

5. Then we have to Create a Database table for the operations.
USE [Testing]
GO
/****** Object: Table [dbo].[Product] Script Date: 19-04-2020 16:06:56 ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE TABLE [dbo].[Product](
[ProductId] [int] IDENTITY(1,1) NOT NULL,
[ProductName] [nvarchar](100) NULL,
[Quantity] [int] NULL,
[Price] [int] NULL,
PRIMARY KEY CLUSTERED
(
[ProductId] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
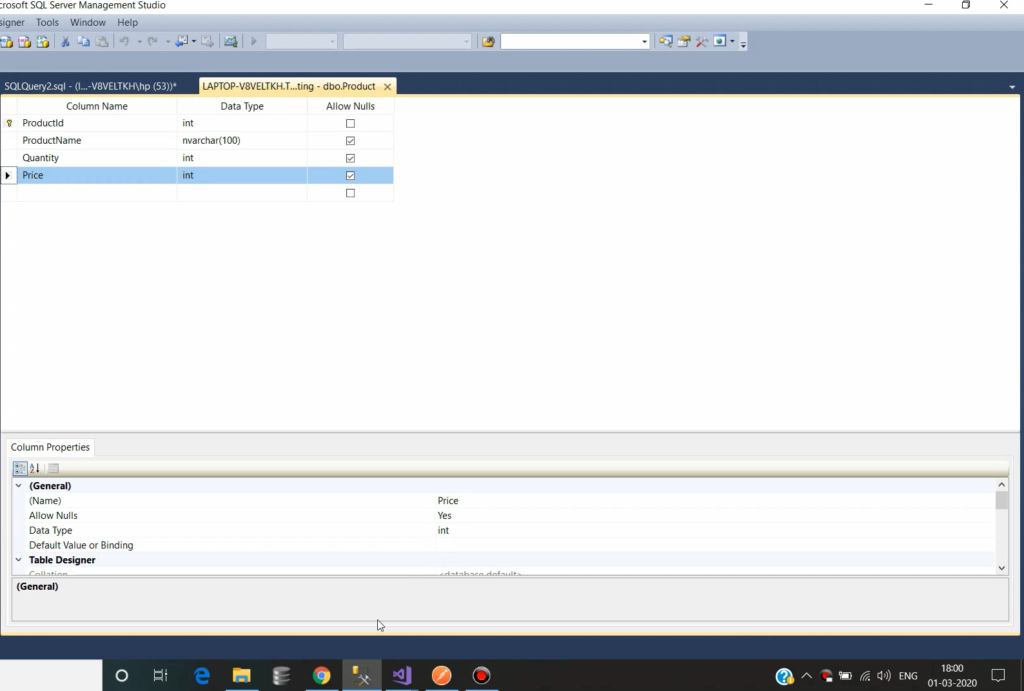
6. After that we have to install Entity Framework because we choose empty when we are creating our Web Application. So we install Entity through nuget package.

7. After that we have to add ADO.Net Entity Data Model for the EF Diagram and Database Connection for our project.

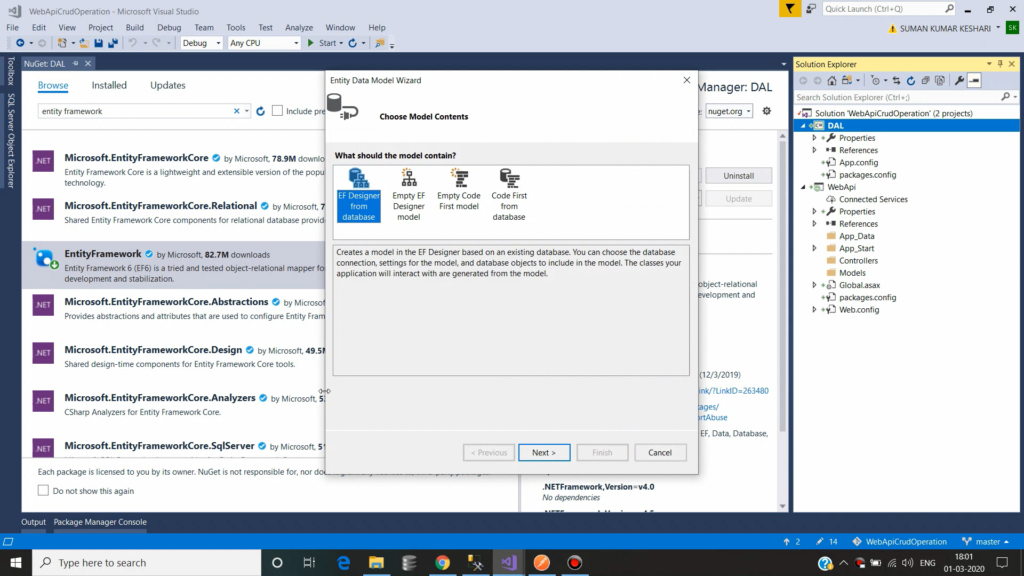

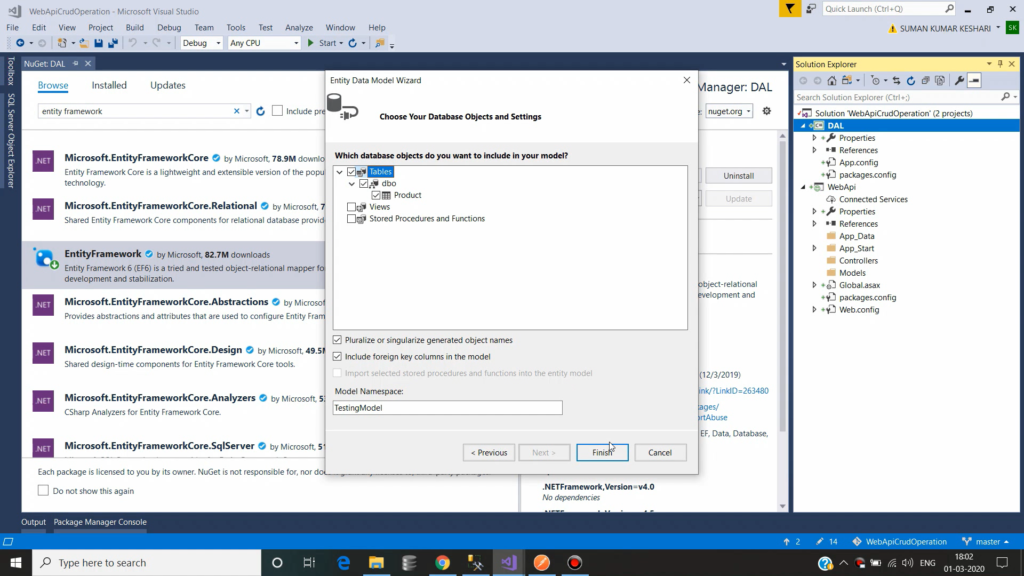
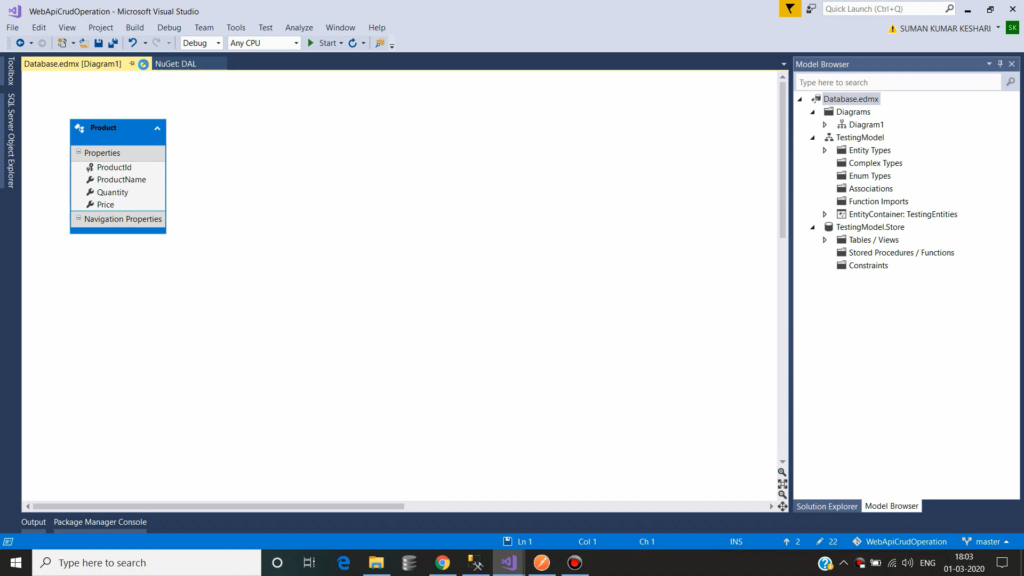
8. After that we have to add a Model folder in the DAL for the services and classes for CRUD Operation as we deal with all the Database related things in DAL.

Product Model Class
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DAL.Models
{
public class ProductModel
{
public int ProductId { get; set; }
public string ProductName { get; set; }
public Nullable<int> Quantity { get; set; }
public Nullable<int> Price { get; set; }
}
}
Product Service for CRUD Operation.
As it contains all the CRUD related codes like CREATE READ UPDATE DELETE – CRUD
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DAL.Models
{
public class ProductServices
{
TestingEntities db = new TestingEntities();
public bool Delete(int ProductId)
{
Product product = db.Products.Find(ProductId);
db.Products.Remove(product);
int result = db.SaveChanges();
if (result > 0)
return true;
else
return false;
}
public ProductModel GetProductById(int ProductId)
{
ProductModel model = new ProductModel();
Product product = db.Products.Find(ProductId);
model.ProductId = product.ProductId;
model.ProductName = product.ProductName;
model.Quantity = product.Quantity;
model.Price = product.Price;
return model;
}
public IEnumerable<ProductModel> GetAllProducts()
{
var Data = (from p in db.Products
select new ProductModel
{
ProductId = p.ProductId,
ProductName = p.ProductName,
Price = p.Price,
Quantity = p.Quantity
}).ToList();
return Data;
}
public bool Insert(ProductModel item)
{
Product product = new Product();
product.ProductName = item.ProductName;
product.Quantity = item.Quantity;
product.Price = item.Price;
db.Products.Add(product);
int result = db.SaveChanges();
if (result > 0)
return true;
else
return false;
}
public bool Update(ProductModel item, int ProductId)
{
Product product = db.Products.Find(ProductId);
product.ProductName = item.ProductName;
product.Quantity = item.Quantity;
product.Price = item.Price;
db.Entry(product).State = System.Data.Entity.EntityState.Modified;
int result = db.SaveChanges();
if (result > 0)
return true;
else
return false;
}
}
}
ASP.NETWeb API controller class is inherited from ApiController classes (System.Web.Http.ApiController) and ASP.NET MVC Controller class inherited from Controller (System.Web.MVC.Controller).
Asp.net Web API works with the following HTTP verbs:
HTTP VERBS | DESCRIPTION |
Get | To get collection(list) or single member. To get the list of members or particular member. http://www.csharpcorner.com/api/values : All Members List http://www.csharpcorner.com/api/values/1 : Particular member which id = 1 |
Post | To create a new member detail. |
Put | To update member detail |
Delete | To delete a particular member. |
Configure routing table for ApiController
When http request receives by Web API framework, it uses routing table to determine which action to be invoke. Public methods of ApiController are called as action methods.
Web API framework first selects controller by IHttpControllerSelector.SelectController method and then selects action method by IHttpActionSelector.SelectAction depending on matching value in request uri and configured route table.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web.Http;
namespace WebApi
{
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Web API configuration and services
// Web API routes
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
}
}
}
You can configure route table in WebApiConfig.cs file. So open this file from App_Start folder add below code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Http;
using System.Web.Routing;
namespace WebApi
{
public class WebApiApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
GlobalConfiguration.Configure(WebApiConfig.Register);
}
}
}
Make sure the above code is present in Global.asx.cs file.
9. Now we are going to add Controller in our WebAPI project for the further calling of Services and doing the CRUD Operation. Below are the codes for the Controller Side.
using DAL.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
namespace WebApi.Controllers
{
[RoutePrefix("api/Product")]
public class ProductMasterController : ApiController
{
readonly ProductServices services = new ProductServices();
[Route("GetAll")]
public IEnumerable<ProductModel> GetAllProducts()
{
return services.GetAllProducts();
}
[Route("GetProduct")]
public ProductModel GetProductById(int ProductId)
{
return services.GetProductById(ProductId);
}
[Route("InsertProduct")]
[HttpPost]
public HttpResponseMessage CreateProduct(ProductModel product)
{
if(services.Insert(product))
{
return Request.CreateResponse(HttpStatusCode.OK, "Date Saved Successfully");
}
else
{
return Request.CreateResponse(HttpStatusCode.InternalServerError, "Error Occured");
}
}
[Route("UpdateProduct")]
[HttpPost]
public HttpResponseMessage UpdateProduct(ProductModel product,int ProductId)
{
if (services.Update(product,ProductId))
{
return Request.CreateResponse(HttpStatusCode.OK, "Date Updated Successfully");
}
else
{
return Request.CreateResponse(HttpStatusCode.InternalServerError, "Error Occured");
}
}
[Route("DeleteProduct")]
[HttpGet]
public HttpResponseMessage DeleteProduct(int ProductId)
{
if (services.Delete(ProductId))
{
return Request.CreateResponse(HttpStatusCode.OK, "Date Deleted Successfully");
}
else
{
return Request.CreateResponse(HttpStatusCode.InternalServerError, "Error Occured");
}
}
}
}
As you see that I uses RoutePrefix and Route for the calling of the API from the URL or through POSTMAN. Below are the images when calling these API’s through POSTMAN.

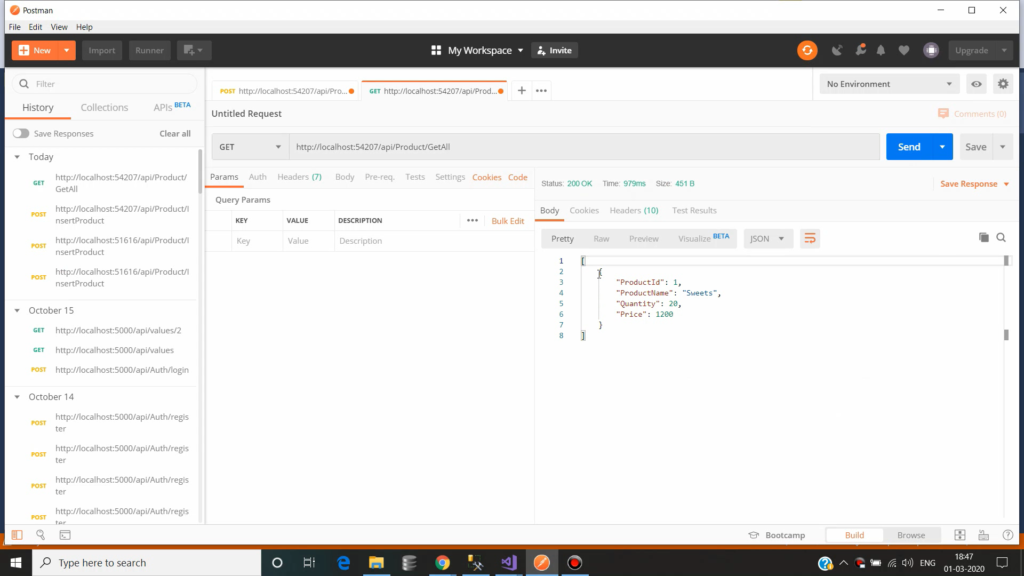
In the same way you can call the Update and Delete API.
I have also attach a YouTube tutorial for this where you can find a brief description about this topic. Thank You.
constantly i used to read smaller articles which as well clear their motive, and that is also happening with this paragraph which
I am reading at this time.
Thanks for your true feedback keep visit,
Team codehunger