
In this article we will see, how to create database seeder in laravel 8, I will guide you on how we create dummy data and insert it into our database.
I will insert data in our users table, before moving forward I want to tell you what is database seeder.
Laravel provides a tool to add sample or dummy data to our databases automatically. which calls it database seeding.
Laravel includes the ability to seed your database with test data using seed classes. All seed classes are stored in the database/seeders
directory. By default, a DatabaseSeeder
class is defined for you. From this class, you may use the call
method to run other seed classes, allowing you to control the seeding order. You can learn more about database seeding from the official website from here.
To create database seeder we will below steps
- Install Laravel 8
- Create Seeder
- Write Code In Seeder
- Run the Seeder
Step – 1 Installation of Laravel Application
Run the below command to install Laravel
composer create-project --prefer-dist laravel/laravel learn
Step – 2 Create Seeder In Laravel
Run the below command to create seeder in Laravel, this Below command will create a seeder under database/seeders named as UsersTableDataSeeder.php

Step – 3 Write Code in Seeder
Now below code will create dummy data for users table, I will insert username,email and password.
<?php
namespace Database\Seeders;
use App\Models\User;
use Illuminate\Database\Seeder;
class UsersTableDataSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
User::create([
'name' => 'code',
'email' => 'test@codehunger.in',
'password' => bcrypt('123456')
]);
}
}

Step – 4 Run the seeder
php artisan db:seed --class=UsersTableDataSeeder
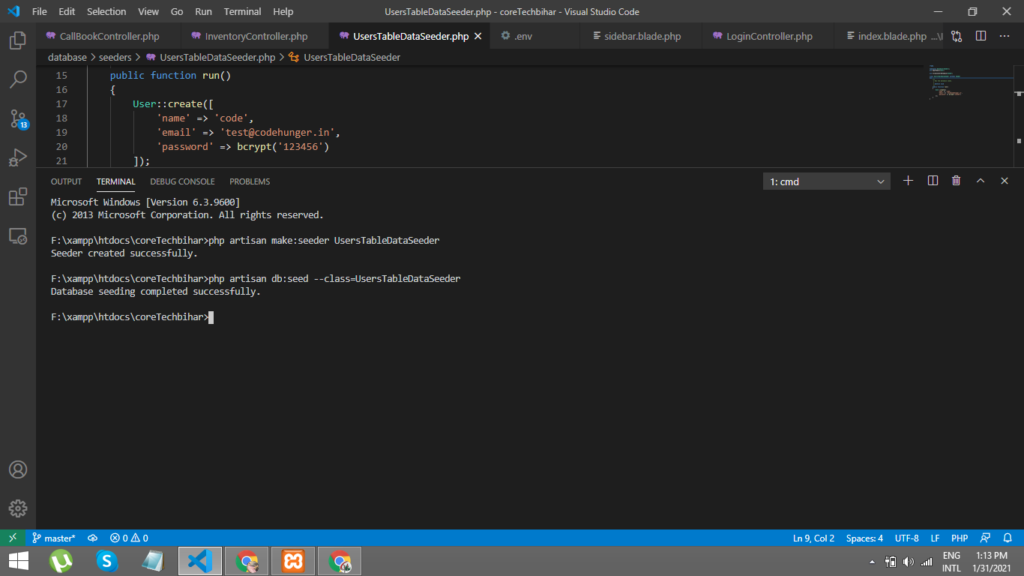

I hope you like the above article,still confused feel free to comment.