Variables & Constants In C
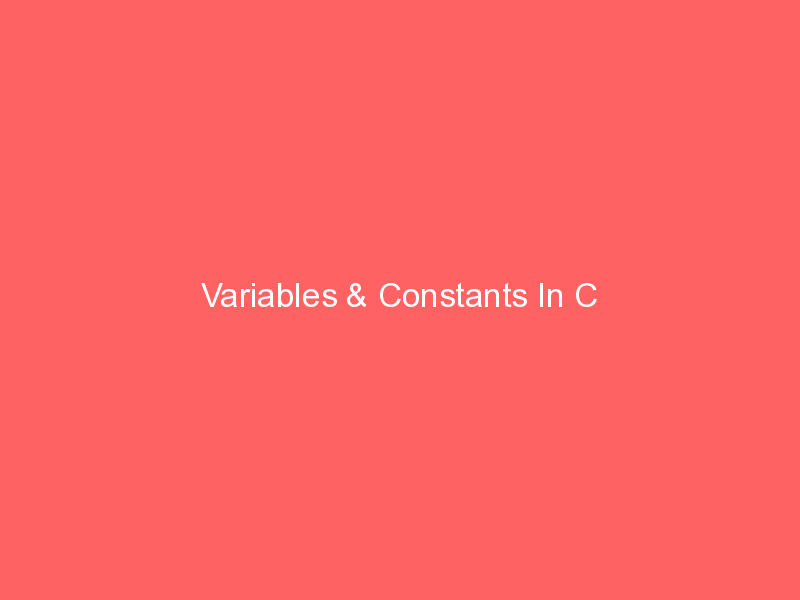
(1).Variables
- A variable in C Programming is called as container to store the data.
- A variable name may have different data types to identify the type of value stored.
- Suppose we declare variable of type integer then it can store only integer values.
- Variable is considered as one of the building blocks of C Programming which is also called as an identifier.
- A Variable is a name given to the memory location where the actual data is stored.
Following are the types of variable in C :
- Local Variables
- Global Variables
Local Variables
- Local Variable is Variable having Local Scope.
- Local Variable is accessible only from function or block in which it is declared.
- Local variable is given Higher Priority than the Global Variable.
Global Variables
- Global Variable is Variable that is Globally available.
- Scope of Global variable is throughout the program [ i.e in all functions including main() ]
- Global variable is also visible inside function , provided that it should not be re-declared with same name inside function because “High Priority is given to Local Variable than Global”
- Global variable can be accessed from any function.
(2). Rules For Variable Name
- Characters allowed : Underscore(_), Capital Letters ( A – Z ), Small Letters ( a – z ), and Digits ( 0 – 9 ).
- Blank spaces and commas are not allowed.
- No Special Symbols other than underscore(_) are allowed.
- First Character should be alphabet or Underscore.
- Variable name Should not be Reserved Keywords.
(3). Keywords.
Keywords are reserved words which have standard, predefined meaning in C. They cannot be used as program-defined identifiers
Generally all keywords are in lower case although uppercase of same names can be used as identifiers.
List of C keywords are as follows :
char int long union continue goto while if double enum const for sizeof do else struct register float signed volatile typedef switch break extern short void auto case static return unsigned default
(4). Data Types
- DataTypes are used for declaring variables And functions of different types.
- When Program store data in variables, It is necessary that each variable must be assigned a specific data type.
Following are the list of Data Types in C :
Keyword | Memory | Range |
---|---|---|
char or signed char | 1 Byte | -128 to 127 |
unsigned char | 1 Byte | 0 to 255 |
int or signed int | 2 Byte | -32,768 – 32,767 |
unsigned int | 2 Byte | 0 to 65535 |
short int or signed short int | 1 Byte | -128 to 127 |
unsigned short int | 1 Byte | 0 to 255 |
long or signed long | 4 Bytes | -2,147,483,648 to 2,147,483,647 |
unsigned long | 4 Bytes | 0 to 4,294,967,295 |
float | 4 Bytes | 3.4E – 38 to 3.4E + 38 |
double | 8 Byte | 1.7E – 308 to 1.7E + 308 |
long double | 10 Bytes | 3.4E-4932 to 1.1E + 4932 |
Declaration of variable :
main() { /* declaration */ //long int is datatype and amount is variable name long int amount; //int is datatype and code is variable name int code; char c; double average; float x,y; }
(5).Constants.
A constant is an identifier with an associated value which cannot be altered by the program during execution.
How to declare constant variable ?
We can declare constant variable using const keyword.
Example of declaring constant variable
//Syntax for declaring constant variable
data type const variable_name = value;
//float constant
float const pi = 3.14;
//integer constant
int const a = 5;
//character constant
char const yes = 'y';
(6). Symbolic Constants.
Symbolic Constant is a name that substitutes for a sequence of a characters or a numeric constant, a character constant or a string constant.
The syntax is as follow :
#define name text
where,
name implies symbolic name in a caps
text implies value or the text.
For Example :
#define printf print
#define MAX 100
#define TRUE 1
#define FALSE 0
#define SIZE 0
The # character is used for preprocessor commands. A preprocessor is a system program, which comes into action prior to Compiler, ans it replaces the replacement text by the actual tet. This will allow correct use of the statement printf
//Syntax for declaring constant variable
data type const variable_name = value;
//float constant
float const pi = 3.14;
//integer constant
int const a = 5;
//character constant
char const yes = 'y';
(6). Symbolic Constants.
Symbolic Constant is a name that substitutes for a sequence of a characters or a numeric constant, a character constant or a string constant.
The syntax is as follow :
#define name text
where,
name implies symbolic name in a caps
text implies value or the text.
For Example :
#define printf print
#define MAX 100
#define TRUE 1
#define FALSE 0
#define SIZE 0
The # character is used for preprocessor commands. A preprocessor is a system program, which comes into action prior to Compiler, ans it replaces the replacement text by the actual tet. This will allow correct use of the statement printf
Created By.
Ranjit Iwale.
Thank you.