React Native – AsyncStorage

AsyncStorage is an unencrypted, asynchronous, persistent, key-value storage system that is global to the app. It should be used instead of LocalStorage.
It is recommended that you use an abstraction on top of AsyncStorage instead of AsyncStorage directly for anything more than light usage since it operates globally.
On iOS, AsyncStorage is backed by native code that stores small values in a serialized dictionary and larger values in separate files. On Android, AsyncStorage will use either RocksDB or SQLite based on what is available.
The AsyncStorage JavaScript code is a facade that provides a clear JavaScript API, real Error objects, and non-multi functions. Each method in the API returns a Promise object.
Importing the AsyncStorage library:
import { AsyncStorage } from 'react-native';
Persist Data:
React Native AsyncStorage saves the data using setItem() method as:
AsyncStorage.setItem('key', 'value');
Example of persisting the single value:
let name = "Michal";
AsyncStorage.setItem('user',name);
Example of persisting multiple values in an object:
let obj = {
name: 'Michal',
email: 'michal@gmail.com',
city: 'New York',
}
AsyncStorage.setItem('user',JSON.stringify(obj));
Fetch Data:
React Native AsyncStorage fetches the saved data using getItem() method as:
await AsyncStorage.getItem('key');
Example to fetch the single value:
await AsyncStorage.getItem('user');
Example to fetch value from an object:
let user = await AsyncStorage.getItem('user');
let parsed = JSON.parse(user);
alert(parsed.email);
App.js
import React, {Component} from 'react';
import {Platform, StyleSheet, Text,
View,TouchableOpacity, AsyncStorage,
} from 'react-native';
export default class App extends Component{
saveData(){
let name = "CodeHunger";
AsyncStorage.setItem('user',name);
}
displayData = async ()=>{
try{
let user = await AsyncStorage.getItem('user');
alert(user);
}
catch(error){
alert(error)
}
}
render() {
return (
<View style={styles.container}>
<TouchableOpacity onPress ={this.saveData}>
<Text>Click to save data</Text>
</TouchableOpacity>
<TouchableOpacity onPress ={this.displayData}>
<Text>Click to display data</Text>
</TouchableOpacity>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
});
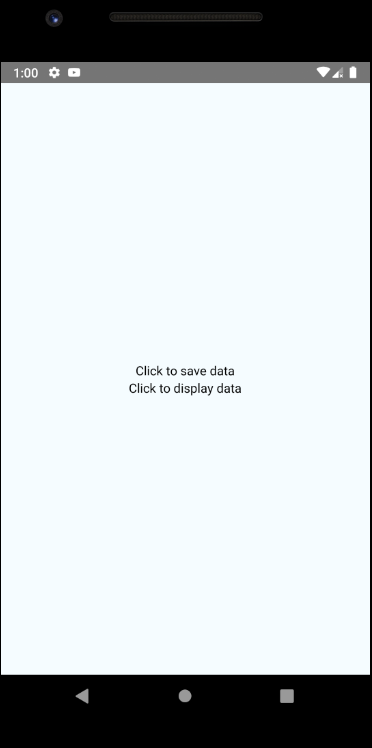
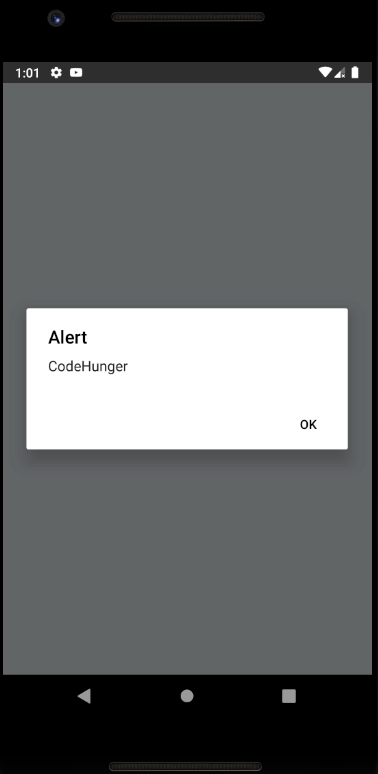
Methods
setItem()
static setItem(key: string, value: string, [callback]: ?(error: ?Error)=>void)
The setItem() sets the value for a key and invokes a callback upon compilation. It returns a Promise object.
getItem()
static getItem(key: string, [callback]: ?(error: ?Error, result: ?string)) =>void)
The getItem() fetches an item from a key and invokes a callback upon completion. It returns a Promise object.
removeItem()
static removeItem(key: string, [callback]: ?(error: ?Error) => void)
The removeItem() removes an item for a key and invokes a callback upon compilation. It returns a Promise object.
mergeItem()
static mergeItem(key: string, value: string, [callback]: ?(error: ?Error) => void)
The mergeItem() merges the existing key’s value with the input value and assuming both values are stringified JSON. It returns a Promise object.
NOTE: This method is not supported by all the native implementations.
clear()
static clear([callback]: ?(error: ?Error) => void)
The clear() method erases all AsynchStorage from all clients, libraries, etc. It is suggested that don’t call this, instead of this you may use removeItem or multiRemove to clear only your app’s keys. It returns the Promise object.