
In this section we are going to see the different image components in our React Native Application.
Full-Screen Background Image
In this section we see how we can set the full screen background image in our application. For that we have to import the Built-in Component ImageBackground from react-native library.
App.js code
import React from 'react';
import { View, Text, ImageBackground, StyleSheet, Image } from 'react-native';
export default class App extends React.Component {
render() {
return (
<ImageBackground
style={{ flex: 1 }}
source={require('./assets/img.jpg')}
>
<View style={styles.MainContainer}>
<Image
source={require('./assets/react.png')}
style={{ width: 40, height: 40, marginTop: 90 }}
/>
<Text style={styles.TextStyle}>https://blog.codehunger.in</Text>
</View>
</ImageBackground>
);
}
}
const styles = StyleSheet.create({
MainContainer: {
flex: 1,
alignItems: 'center',
},
TextStyle: {
color: '#000',
textAlign: 'center',
fontSize: 30,
marginTop: 10,
},
});
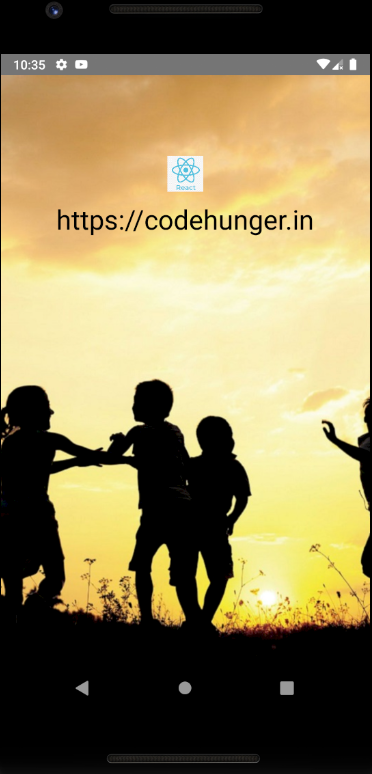
Slider Image Gallery
In this section we are going to see how we use Slider Image Gallery in our React Native Application. We will use Gallery component provided by react-native-image-gallery to make a Slider Image Gallery in React Native. To use Gallery we have to install react-native-image-gallery package.
npm install react-native-image-gallery --save
App.js
import React from 'react';
import Gallery from 'react-native-image-gallery';
export default class App extends React.Component {
constructor() {
super();
this.state = {
imageuri: '',
ModalVisibleStatus: false,
};
this.state = { items: [] };
}
componentDidMount() {
var that = this;
let itemss=[]
let items = Array.apply(null, Array(60)).map((v, i) => {
return {
source: {
uri: 'http://placehold.it/100x200?text=' + (i + 1),
},
};
});
that.setState({ items });
}
render() {
//Image Slider
return (
<Gallery
style={{ flex: 1, backgroundColor: 'black' }}
initialPage="1"
images={this.state.items}
/>
);
}
}
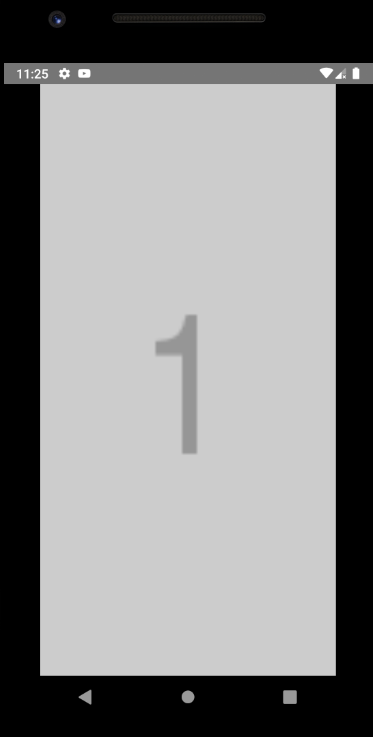
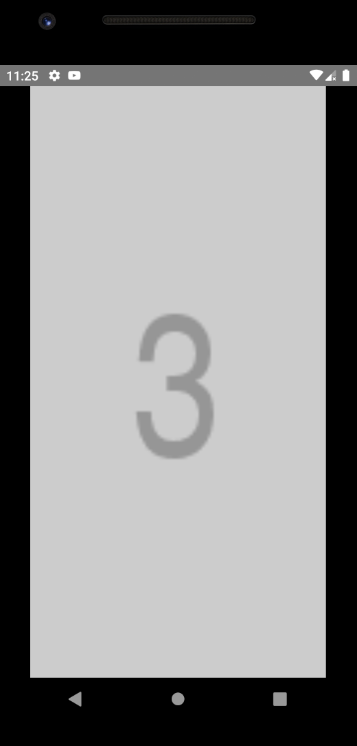