React Native
React Native – Play Audio

In this tutorial we are going to see how we play Audio in React Native Application. First we gonna see how we play Audio.
To Play Audio in React Native
To play Sound / Music in React Native app we are going to use Sound
component provided by react-native-sound
which supports playing sound clips on iOS, Android, and Windows. react-native-sound
does not support streaming.
You can run 3 different types of sounds using this library which are listed below:
- aac
- mp3
- wav
For playing Audio we have to install some dependency:
npm install react-native-sound --save
After that we have to auto link.
react-native link react-native-sound
To play the sound from remote URL
var sound1 = new Sound('your url here', '',
(error, sound) => {
if (error) {
alert('error' + error.message);
return;
}
sound1.play(() => {
sound1.release();
});
});
App.js
import React, { Component } from 'react';
import {
StyleSheet,
Text,
TouchableOpacity,
View,
ScrollView,
SafeAreaView,
} from 'react-native';
import Sound from 'react-native-sound';
const audioList = [
{
title: 'Phir Mohabbat',
isRequire: true,
url: require('./assets/songs/04 - Phir Mohabbat.mp3'),
},
{
title: 'Awari - Ek Villain',
url: require('./assets/songs/04 Awari - Ek Villain (PagalWorld.com) 320Kbps.mp3'),
},
{
title: '3 Peg',
isRequire: true,
url: require('./assets/songs/3 Peg -(Singlejatt.in).mp3'),
}
];
var sound1, sound2, sound3;
function playSound(item, index) {
if (index == 0) {
sound1 = new Sound(item.url, (error, sound) => {
if (error) {
alert('error' + error.message);
return;
}
sound1.play(() => {
sound1.release();
});
});
} else if (index == 1) {
sound2 = new Sound(item.url, '', (error, sound) => {
if (error) {
alert('error' + error.message);
return;
}
sound2.play(() => {
sound2.release();
});
});
} else if (index == 2) {
sound3 = new Sound(item.url, (error, sound) => {
if (error) {
alert('error' + error.message);
return;
}
sound3.play(() => {
sound3.release();
});
});
}
}
function stopSound(item, index) {
if (index == 0 && sound1) {
sound1.stop(() => {
console.log('Stop');
});
} else if (index == 1 && sound2) {
sound2.stop(() => {
console.log('Stop');
});
} else if (index == 2 && sound3) {
sound3.stop(() => {
console.log('Stop');
});
}
}
function componentWillUnmount() {
sound1.release();
sound2.release();
sound3.release();
}
class App extends Component {
constructor(props) {
super(props);
Sound.setCategory('Playback', true);
this.state = {
tests: {},
};
}
render() {
return (
<SafeAreaView style={{ flex: 1 }}>
<View style={styles.container}>
<Text style={styles.headerTitle}>
Example to Play Music in React Native
</Text>
<ScrollView style={styles.container}>
{audioList.map((item, index) => {
return (
<View style={styles.feature} key={item.title}>
<Text style={{ flex: 1, fontSize: 14 }}>{item.title}</Text>
<TouchableOpacity
onPress={() => {
return playSound(item, index);
}}>
<Text style={styles.buttonPlay}>Play</Text>
</TouchableOpacity>
<TouchableOpacity
onPress={() => {
return stopSound(item, index);
}}>
<Text style={styles.buttonStop}>Stop</Text>
</TouchableOpacity>
</View>
);
})}
</ScrollView>
</View>
</SafeAreaView>
);
}
}
export default App;
const styles = StyleSheet.create({
container: {
flex: 1,
},
headerTitle: {
fontSize: 16,
color: 'white',
fontWeight: 'bold',
paddingVertical: 20,
textAlign: 'center',
backgroundColor: 'rgba(00,00,80,1)',
},
buttonPlay: {
fontSize: 16,
color: 'white',
backgroundColor: 'rgba(00,80,00,1)',
borderWidth: 1,
borderColor: 'rgba(80,80,80,0.5)',
overflow: 'hidden',
paddingHorizontal: 15,
paddingVertical: 7,
},
buttonStop: {
fontSize: 16,
color: 'white',
backgroundColor: 'rgba(80,00,00,1)',
borderWidth: 1,
borderColor: 'rgba(80,80,80,0.5)',
overflow: 'hidden',
paddingHorizontal: 15,
paddingVertical: 7,
},
feature: {
flexDirection: 'row',
padding: 10,
alignSelf: 'stretch',
alignItems: 'center',
borderTopWidth: 1,
borderTopColor: 'rgb(180,180,180)',
borderBottomWidth: 1,
borderBottomColor: 'rgb(230,230,230)',
},
});
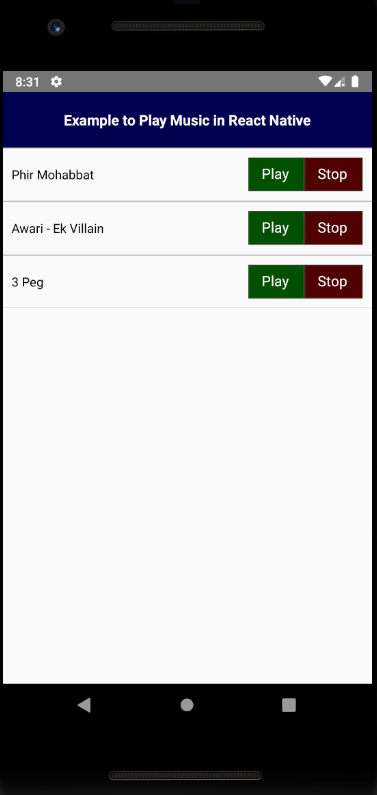