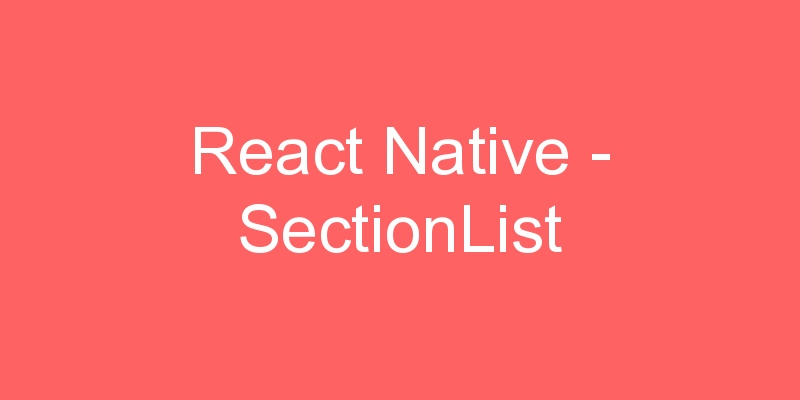
The SectionList component allows us to create a list of content that is broken up into scrollable sections. SectionLists are similar to Flatlists, but they extend the FlatList functionality even further. The broken data can be implemented using its section header prop renderSectionHeader.
To create a list view in React Native using SectionList, first import the SectionList component in code from the React Native library.
import { SectionList } from 'react-native';
Props
sections | renderItem | initialNumToRender | keyExtractor |
renderSectionHeader | renderSectionFooter | onRefresh | inverted |
extraData | onEndReached | keyExtractor | legacyImplementation |
onViewableItemsChanged | refreshing | removeClippedSubviews | ListHeaderComponent |
SectionSeparatorComponent | stickySectionHeadersEnabled | onEndReachedThreshold | ListEmptyComponent |
import React from 'react';
import { StyleSheet, ScrollView, View, Text, SectionList } from 'react-native';
export default class SectionListBasics extends React.Component {
render() {
return (
<View style={styles.container}>
<SectionList
sections={[
{
title: 'Top Google Seaches in 2020',
data: [
'World Cup',
'Avicii',
'Mac Miller',
'Avengers',
'Black Panther',
],
},
{
title: 'Top News Trends of 2020',
data: [
'World Cup',
'Hurricane Florence',
'Mega Millions Result',
'Royal Wedding',
'Election Results',
],
},
{
title: 'Top Searched Movies of 2020',
data: [
'Black Panther',
'Dead Pool 2',
'Venom',
'Avengers: Infinity War',
'Bohemian Rhapsody',
],
},
{
title: 'Top Searched Athletes of 2020',
data: [
'Tristan Thompson',
'Alexis Sánchez',
'Lindsey Vonn',
'Shaun White',
'Khabib Nurmagomedov',
],
},
]}
renderItem={({ item }) => <Text style={styles.item}>{item}</Text>}
renderSectionHeader={({ section }) => (
<Text style={styles.sectionHeader}>{section.title}</Text>
)}
keyExtractor={(item, index) => index}
/>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: 22,
},
sectionHeader: {
paddingTop: 2,
paddingBottom: 2,
paddingLeft: 10,
paddingRight: 10,
fontSize: 22,
fontWeight: 'bold',
color: '#fff',
backgroundColor: '#F55145',
},
item: {
padding: 10,
fontSize: 18,
height: 44,
},
});
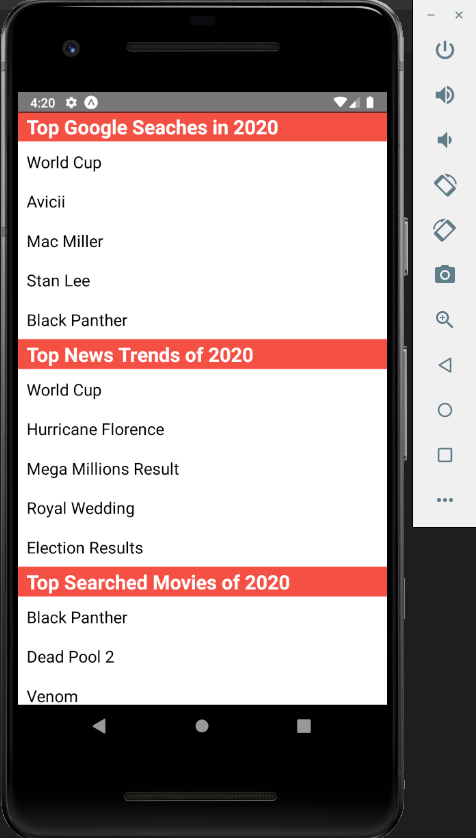
This is a convenience wrapper around <VirtualizedList>
, and thus inherits its props (as well as those of <ScrollView>
that aren’t explicitly listed here, along with the following caveats:
- Internal state is not preserved when content scrolls out of the render window. Make sure all your data is captured in the item data or external stores like Flux, Redux, or Relay.
- This is a
PureComponent
which means that it will not re-render ifprops
remain shallow-equal. Make sure that everything yourrenderItem
function depends on is passed as a prop (e.g.extraData
) that is not===
after updates, otherwise your UI may not update on changes. This includes thedata
prop and parent component state. - In order to constrain memory and enable smooth scrolling, content is rendered asynchronously offscreen. This means it’s possible to scroll faster than the fill rate and momentarily see blank content. This is a tradeoff that can be adjusted to suit the needs of each application, and we are working on improving it behind the scenes.
- By default, the list looks for a
key
prop on each item and uses that for the React key. Alternatively, you can provide a customkeyExtractor
prop.