React Native – Vector Icons
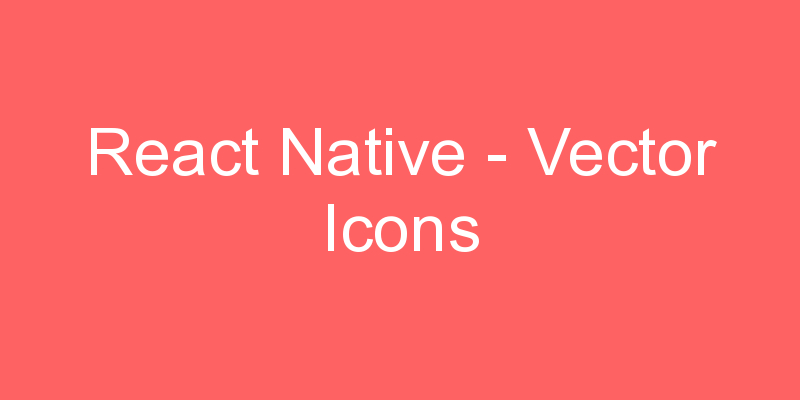
React Native Vector Icons are very popular icons in React Native. In this post, we will see Example to Use Vector Icons in React Native using react-native-vector-icons. Vector Icons are perfect for buttons, logos and nav/tab bars. Vector Icons are easy to extend, style and integrate into your project.
You can use Vector Icons very anywhere easily. You can visit the react-native-vector-icons directory to find a variety of icons.
How to use Icon Component?
For the Vector Icons, we have to import react-native-vector-icons
dependency which will provide two components:
1. Icon Component
You can use this Icon component to create Icons. Prop “name” will render the icon in Android and IOS.
<Icon name="rocket" size={30} color="#900" />
Prop | Description | Default |
---|---|---|
size | Size of the icon can also be passed as fontSize in the style object. | 12 |
name | What icon to show, see Icon Explorer app or one of the links above. | None |
color | The color of the icon. | Inherited |
2. Icon.Button Component
A convenience component for creating buttons with an icon on the left side.
<Icon.Button
name="facebook"
backgroundColor="#3b5998"
onPress={this.loginWithFacebook}>
Login with Facebook
</Icon.Button>
Prop | Description | Default |
---|---|---|
color | Text and icon color, use iconStyle or nest a Text component if you need different colors. | white |
size | Icon size. | 20 |
iconStyle | Styles applied to the icon only, good for setting margins or a different color. Note: use iconStyle for margins or expect unstable behaviour. | {marginRight: 10} |
backgroundColor | Background color of the button. | #007AFF |
borderRadius | Border radius of the button, set to 0 to disable. | 5 |
onPress | A function called when the button is pressed. | None |
We are also going to use these components in our example.
Run the following command for installing Vector Icons
npm install react-native-elements --save
npm install react-native-vector-icons --save
After that link the vector icons to our project.
react-native link react-native-vector-icons
We need to install pods for the iOS
cd ios && pod install && cd ..
App.js
import React, { Component } from 'react';
import { Platform, StyleSheet, Text, View } from 'react-native';
import Icon from 'react-native-vector-icons/FontAwesome';
export default class App extends Component {
render() {
return (
<View style={styles.container}>
<View style={{flexDirection:'row',padding:10}}>
<Icon name="home" size={25} color="#000" />
<Text style={{marginLeft:10}}>Home</Text>
</View>
<View style={{flexDirection:'row',padding:10}}>
<Icon name="camera" size={25} color="#000" />
<Text style={{marginLeft:10}}>Camera</Text>
</View>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
});
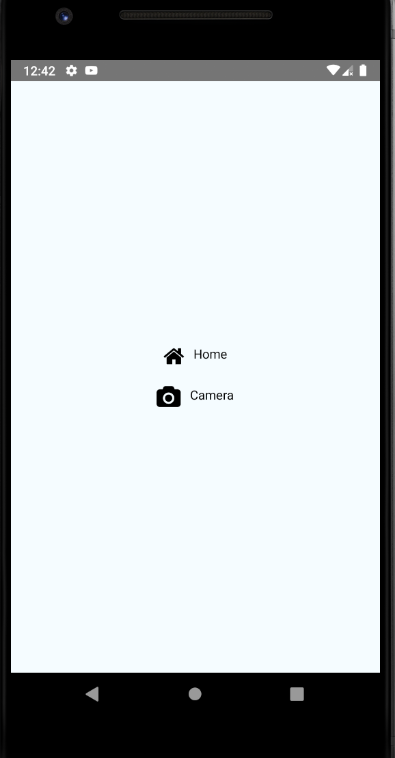