React Native – Integration of Google Map

In this tutorial we are going to implement Google Map in our React Native App using react-native-maps. Many of the developers wants to implement map in their application as per the client requirement. So lets start the tutorial.
First we have to install the react-native-maps using npm.
npm install react-native-maps --save
//After that we have to link this
react-native link react-native-maps
Generate the API Key to Integration of Google map
To get the Map API key from the Google Developer console
– Open the Developer console and log in from your Google account.
– If you have already created any project you can use that or you can create a new one like this.
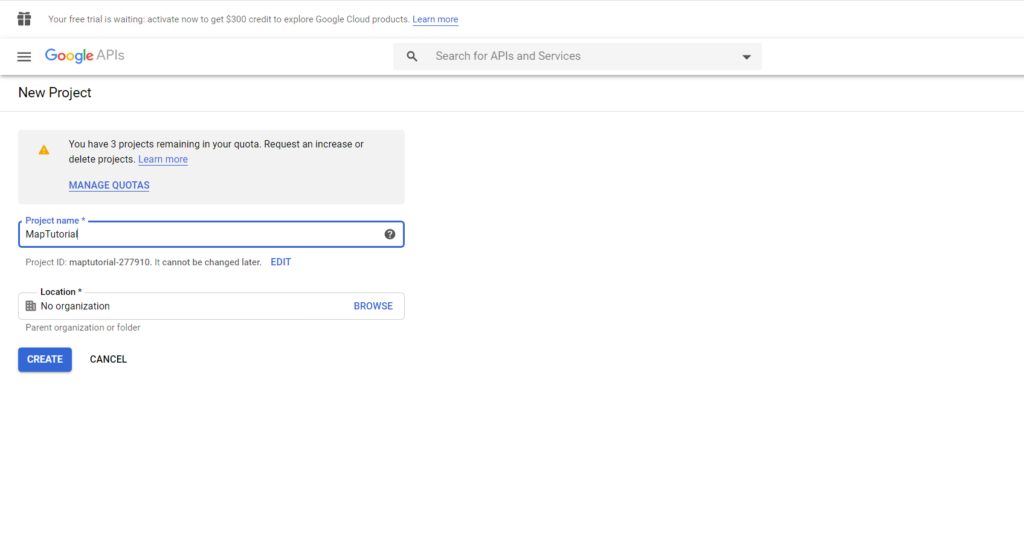
After that go to the credentials section.
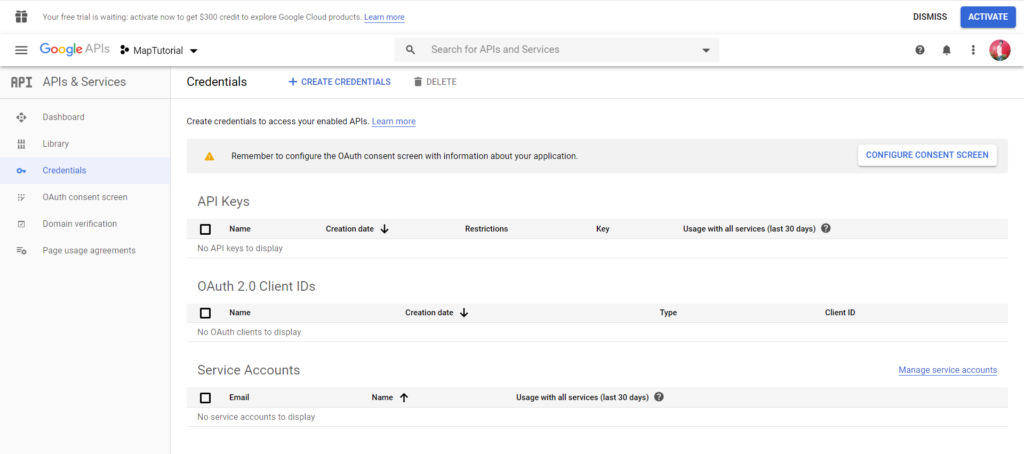
Then click on the create credentials and click on the Api key.
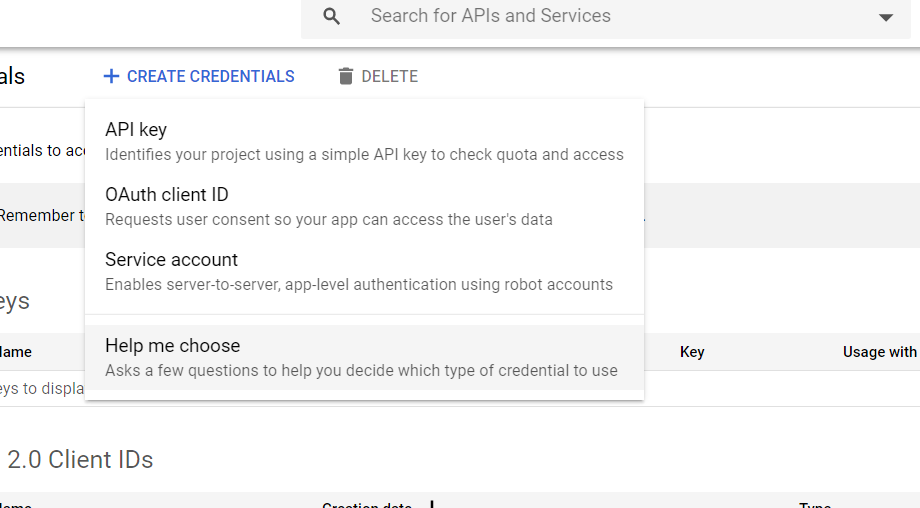
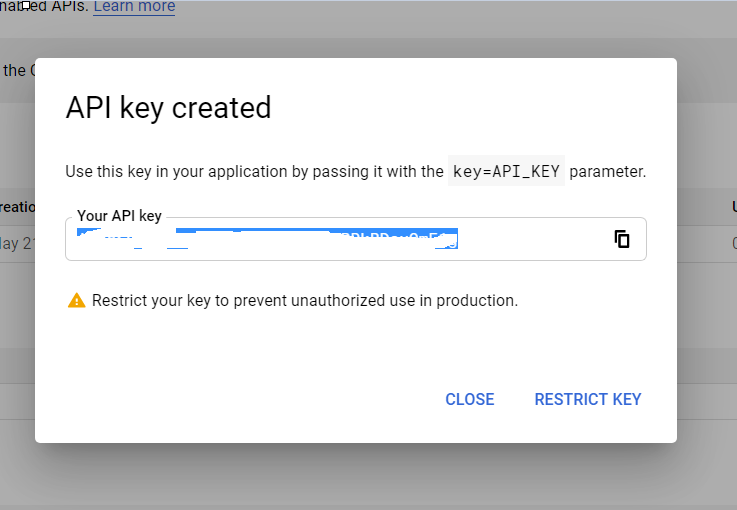
Copy the Api key and then Enable the Api in the library section.
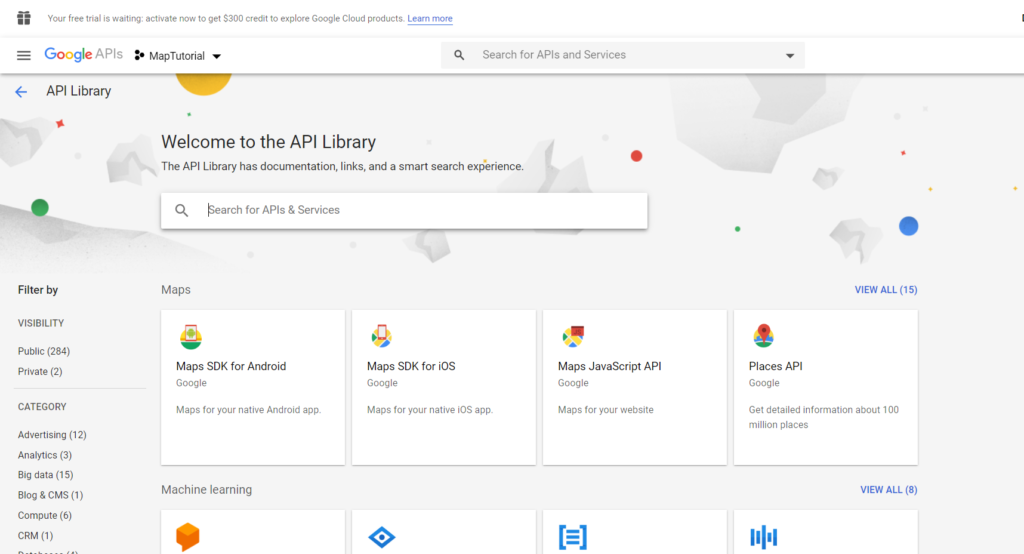
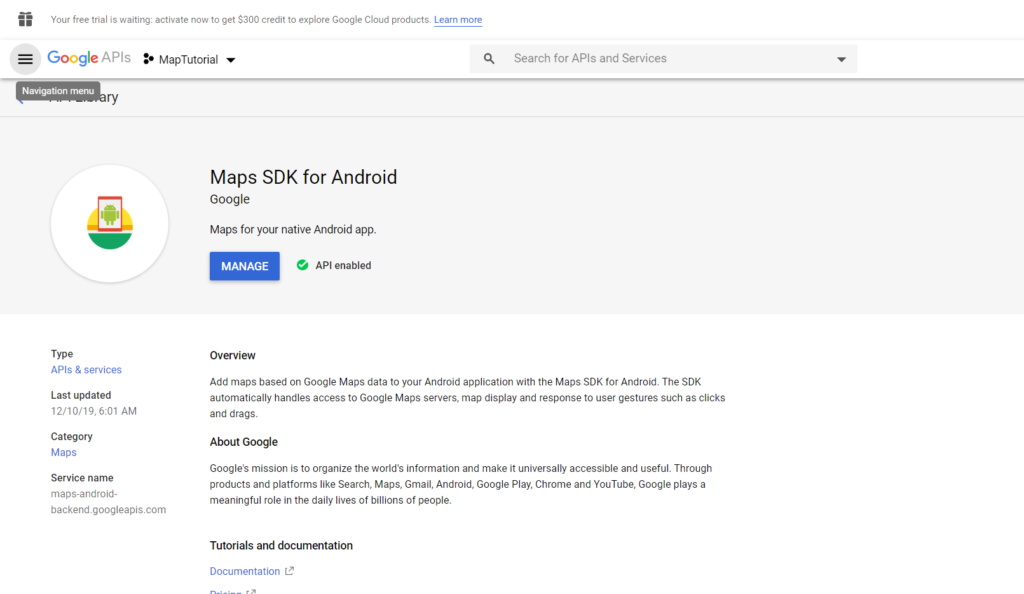
Now after enabling the API key, we have to add this API key to our project’s AndroidManifest.xml file. Just copy the following lines and paste it into your manifest file with your generated API key.
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="Replace this with your API key"/>
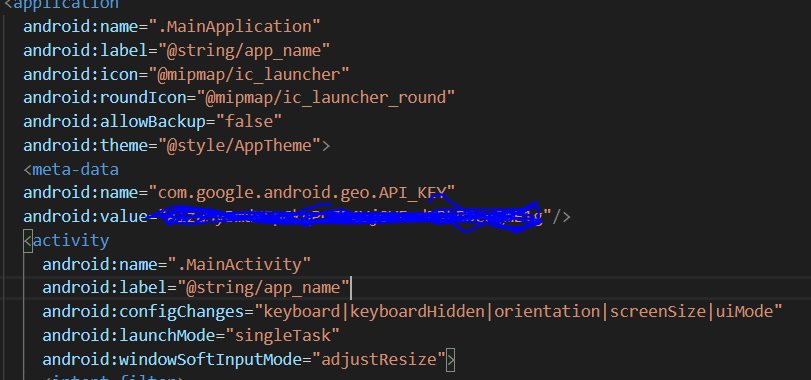
Now Open App.js in any code editor and replace the code with the following code
Please note that we have used customMapStyle={mapStyle} which is for the custom styling of the map in which you just have to provide the mapStyle JSON which will help you to customize your map. To get the custom style JSON please visit https://mapstyle.withgoogle.com/.
App.js
/*This is an Example of React Native Map*/
import React from 'react';
import { StyleSheet, Text, View , TextInput} from 'react-native';
import MapView, {Marker} from 'react-native-maps';
export default class App extends React.Component {
onRegionChange(region) {
this.setState({ region });
}
render() {
var mapStyle=[
{
"elementType": "geometry",
"stylers": [
{
"color": "#ebe3cd"
}
]
},
{
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#523735"
}
]
},
{
"elementType": "labels.text.stroke",
"stylers": [
{
"color": "#f5f1e6"
}
]
},
{
"featureType": "administrative",
"elementType": "geometry.stroke",
"stylers": [
{
"color": "#c9b2a6"
}
]
},
{
"featureType": "administrative.land_parcel",
"elementType": "geometry.stroke",
"stylers": [
{
"color": "#dcd2be"
}
]
},
{
"featureType": "administrative.land_parcel",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#ae9e90"
}
]
},
{
"featureType": "landscape.natural",
"elementType": "geometry",
"stylers": [
{
"color": "#dfd2ae"
}
]
},
{
"featureType": "poi",
"elementType": "geometry",
"stylers": [
{
"color": "#dfd2ae"
}
]
},
{
"featureType": "poi",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#93817c"
}
]
},
{
"featureType": "poi.park",
"elementType": "geometry.fill",
"stylers": [
{
"color": "#a5b076"
}
]
},
{
"featureType": "poi.park",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#447530"
}
]
},
{
"featureType": "road",
"elementType": "geometry",
"stylers": [
{
"color": "#f5f1e6"
}
]
},
{
"featureType": "road.arterial",
"elementType": "geometry",
"stylers": [
{
"color": "#fdfcf8"
}
]
},
{
"featureType": "road.highway",
"elementType": "geometry",
"stylers": [
{
"color": "#f8c967"
}
]
},
{
"featureType": "road.highway",
"elementType": "geometry.stroke",
"stylers": [
{
"color": "#e9bc62"
}
]
},
{
"featureType": "road.highway.controlled_access",
"elementType": "geometry",
"stylers": [
{
"color": "#e98d58"
}
]
},
{
"featureType": "road.highway.controlled_access",
"elementType": "geometry.stroke",
"stylers": [
{
"color": "#db8555"
}
]
},
{
"featureType": "road.local",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#806b63"
}
]
},
{
"featureType": "transit.line",
"elementType": "geometry",
"stylers": [
{
"color": "#dfd2ae"
}
]
},
{
"featureType": "transit.line",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#8f7d77"
}
]
},
{
"featureType": "transit.line",
"elementType": "labels.text.stroke",
"stylers": [
{
"color": "#ebe3cd"
}
]
},
{
"featureType": "transit.station",
"elementType": "geometry",
"stylers": [
{
"color": "#dfd2ae"
}
]
},
{
"featureType": "water",
"elementType": "geometry.fill",
"stylers": [
{
"color": "#b9d3c2"
}
]
},
{
"featureType": "water",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#92998d"
}
]
}
];
return (
<View style={styles.container}>
<MapView
style={styles.map}
initialRegion={{
latitude: 37.78825,
longitude: -122.4324,
latitudeDelta: 0.0922,
longitudeDelta: 0.0421,
}}
customMapStyle={mapStyle}
>
<Marker
draggable
coordinate={{
latitude: 37.78825,
longitude: -122.4324,
}}
onDragEnd={(e) => alert(JSON.stringify(e.nativeEvent.coordinate))}
title={'Test Marker'}
description={'This is a description of the marker'}
/>
</MapView>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
position:'absolute',
top:0,
left:0,
right:0,
bottom:0,
alignItems: 'center',
justifyContent: 'flex-end',
},
map: {
position:'absolute',
top:0,
left:0,
right:0,
bottom:0,
},
});
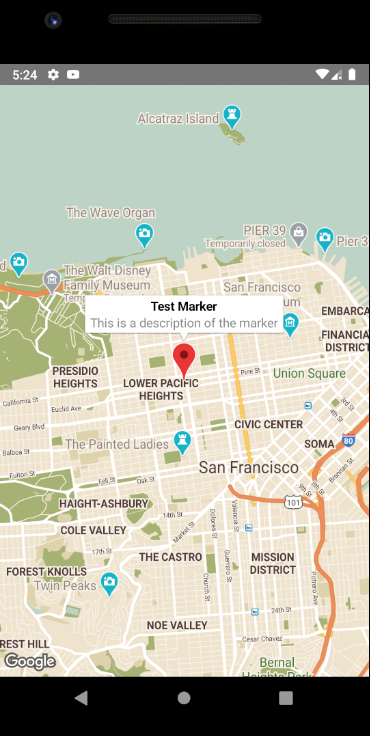