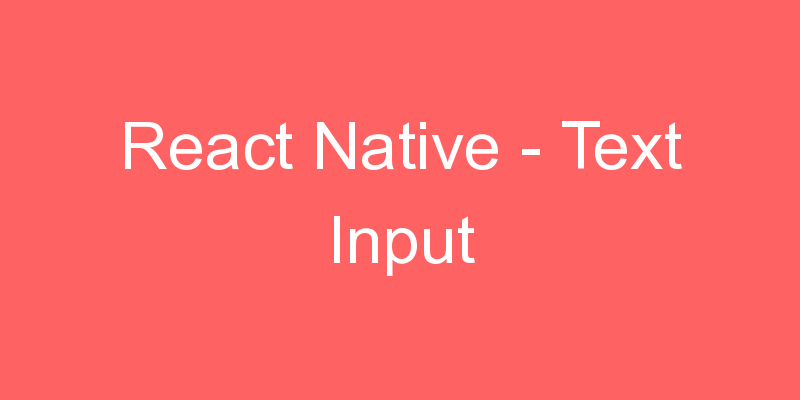
The most basic use case is to plop down a TextInput and subscribe to the onChangeText events to read the user input.
Inputs allow users to enter text into a UI. They typically appear in forms and dialogs. It has an onChangeText prop which requires a function that will be called every time when the text changes, and it also has a value prop that can set a default value into it.
To Import TextInput in the Code
import { TextInput } from 'react-native'
Render Using
<TextInput placeholder = "Email" onChangeText = {this.state.email}/>
Props
allowFontScaling | autoCapitalize | autoCorrect | autoFocus |
blurOnSubmit | caretHidden | clearButtonMode | clearTextOnFocus |
contextMenuHidden | dataDetectorTypes | defaultValue | disableFullscreenUI |
editable | enablesReturnKeyAutomatically | inlineImageLeft | inlineImagePadding |
keyboardAppearance | keyboardType | maxLength | multiline |
numberOfLines | onBlur | onChange | onChangeText |
onContentSizeChange | onEndEditing | onFocus | onKeyPress |
onLayout | onScroll | onSelectionChange | onSubmitEditing |
placeholder | placeholderTextColor | returnKeyLabel | returnKeyType |
scrollEnabled | secureTextEntry | selection | selectionColor |
selectionColor | selectionState | selectTextOnFocus | spellCheck |
textContentType | style | textBreakStrategy | underlineColorAndroid |
import React from 'react';
import { TextInput, View, StyleSheet, Text } from 'react-native';
export default class App extends React.Component {
constructor(props) {
super(props);
this.state = {
username: ''
};
}
render() {
return (
<View style={styles.container}>
<Text style={{color: 'black'}}>{this.state.username}</Text>
<TextInput
value={this.state.username}
onChangeText={(username) => this.setState({ username })}
placeholder={'Username'}
style={styles.input}
/>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: '#ffffff',
},
input: {
width: 250,
height: 44,
padding: 10,
marginBottom: 10,
backgroundColor: '#ecf0f1'
},
});

We can also use multiline prop by making it true.