React Native
React Native – Restart/Reset Current Screen

In some cases, we need to restart or reset the screen again and React Native does not provide the default refresh component to restart the screen. So in that case react-native-restart
can help you by providing RNRestart
Component.
To Import the Component
import RNRestart from 'react-native-restart';
To Restart or Reset the Current Screen
RNRestart.Restart();
For using react-native-start we have to install it using npm
npm install react-native-restart --save
after that you have to link that using
react-native link react-native-restart
App.js code
import React, {Component} from 'react';
import { Text, View, Button, StyleSheet } from 'react-native';
import RNRestart from 'react-native-restart';
export default class App extends Component {
constructor(props) {
super(props);
this.state = { counter: 0 };
}
componentDidMount() {
this._interval = setInterval(() => {
this.setState({
counter: this.state.counter + 1,
});
}, 1000);
}
componentWillUnmount() {
clearInterval(this._interval);
}
onButtonClick = () => {
RNRestart.Restart();
};
render() {
return (
<View style={styles.container}>
<Text style={styles.paragraph}>Example of React Native Restart</Text>
<Text style={styles.paragraph}>{this.state.counter}</Text>
<View>
<Button title="Restart Screen" onPress={this.onButtonClick} />
</View>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
paddingTop: 30,
backgroundColor: '#ecf0f1',
padding: 8,
},
paragraph: {
margin: 24,
fontSize: 18,
fontWeight: 'bold',
textAlign: 'center',
},
});
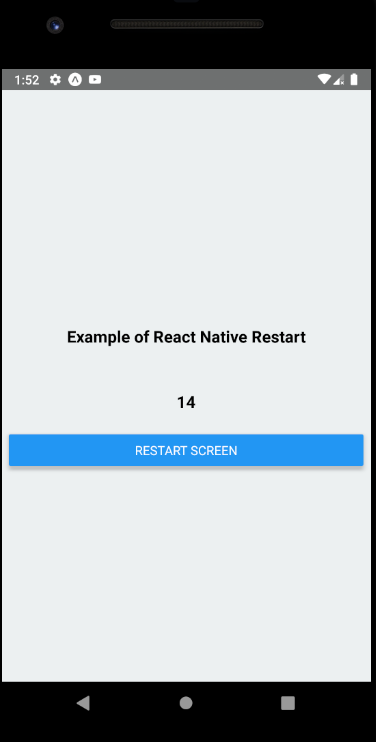